Fundamentals of Python Programming
Everything you need to know about Strings in Python
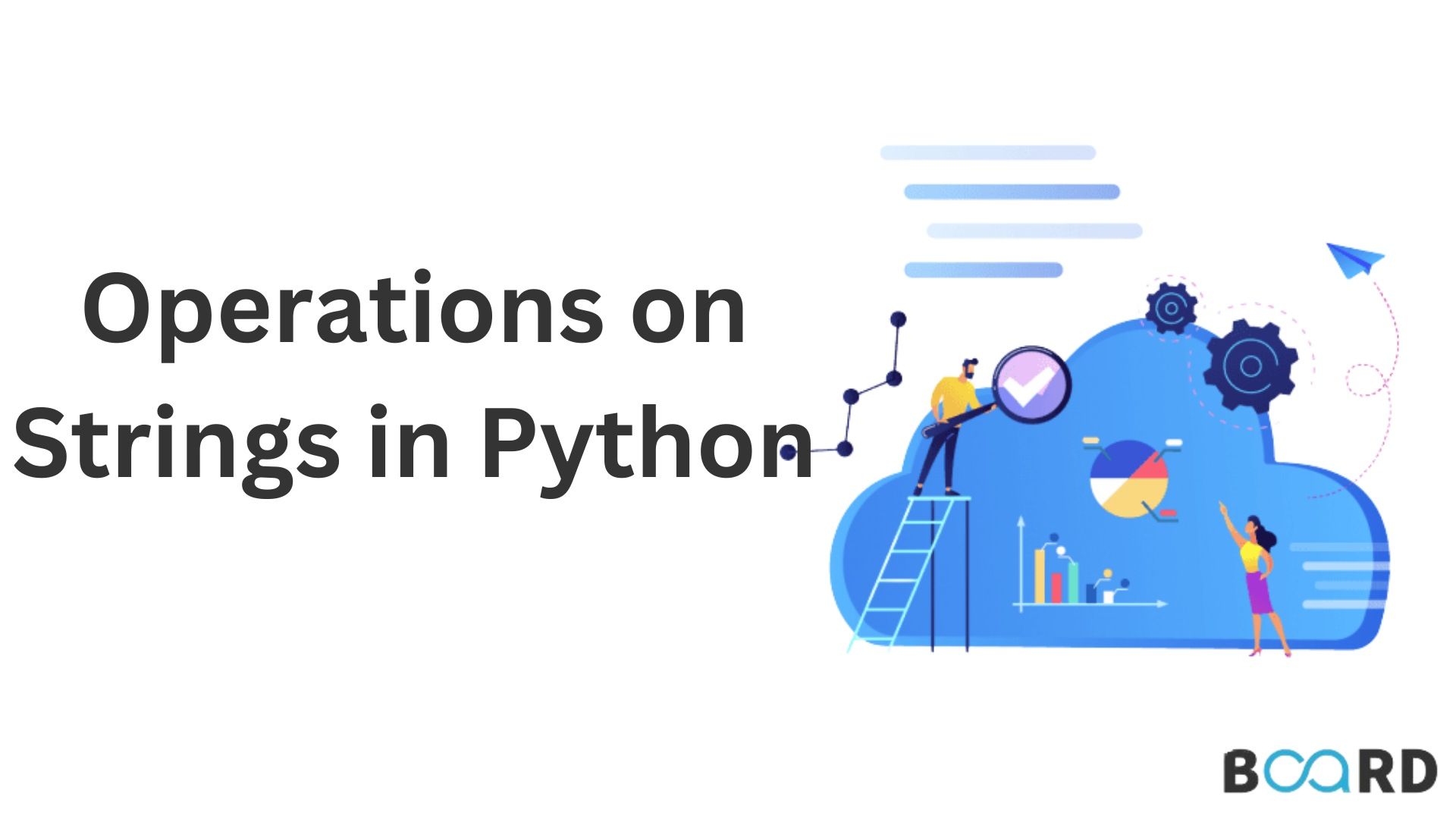
Introduction
A Python string is one of the standard datatypes of Python. First, let us understand what a “string” means.
We define a string as a sequence of characters. By characters, we mean alphabets, digits, symbols, and special characters. A typical Python string is enclosed within single quotes or double quotes or even triple quotes. Strings can also span over multiple lines as depicted below
The utmost important characteristic is that it is an “immutable datatype”. An immutable datatype is a datatype whose value cannot be changed after the assignment of it
Let us understand how to initialize a string through an example :
Example
Output
The further section dwells on the various operations that can be performed on it
Accessing The Elements of a String
The individual characters of a string are indexed or in layman’s terms numbered starting from 0 to the length of the string - 1. With the help of this unique number, we have access to all characters of a string.
For example, the number 0 corresponds to the first character, 1 to the second character, and so on. Another feature supported by Python is negative indexing, which means the individual characters are indexed by negative numbers such as -1 corresponding to the last character, -2 to the second last character, and so on.
The diagram illustrates the indexing of a string indicating both positive as well as negative indexing
Traversing Through a String
Traversal through a string refers to iterating through a string - one character at a time
Let us consider the following code snippet in which we print the string character by character
Example
Output
Quick Activity: Try printing the characters of a string in the reverse order using negative indexing
Output
String Slicing
Sometimes, we may need to access a specific sequence of characters as opposed to a single character by indexing, this is where string slicing comes into play. The range of characters is specified within square brackets of the form [m:n]. The characters starting from the mth index to (n-1)th index will be selected.
If the value of m is not specified, 0 indexes are taken by default and on the other hand, if the value of n is not specified the (length -1) value will be taken by default
Let us understand this through an example:
Output
Formatting of Strings
Python allows you to format your strings using the built-in format method. The format method contains curly braces which correspond to a specific keyword pair or a position or to the word itself. This is illustrated by the following code snippet
Output
Updating/Deleting a Python String
Strings in Python are immutable, that is we cannot change the value of the individual character in place. So how do we modify strings?
This can be achieved through string slicing and concatenation as discussed previously. Concatenation if you don’t know refers to the act of joining two strings together
Example: “Board” + “Infinity” = “BoardInfinity”
Example: Consider the string “BoardqInfinity”, we would like to remove the character q from the string which can be done by the code snippet below:
Output
Similarly, if we would like to add a space between the words it can be done in a similar manner as well
Output
Moreover, the entire string itself can be changed but not a single character directly. The following code demonstrates this:
Output
In addition, the entire string can be deleted with the help of the del keyword. However, once deleted it is not possible to print it as it no longer exists.
String Methods in Python
Python provides an inbuilt method for the manipulation as well as performing various operations on the string. Some of them are listed below for your reference:
Thus, by the end of this tutorial, you would have developed a good understanding of strings in Python regarding how to access them, format them as well as manipulate them.