What is A* Search Algorithm?
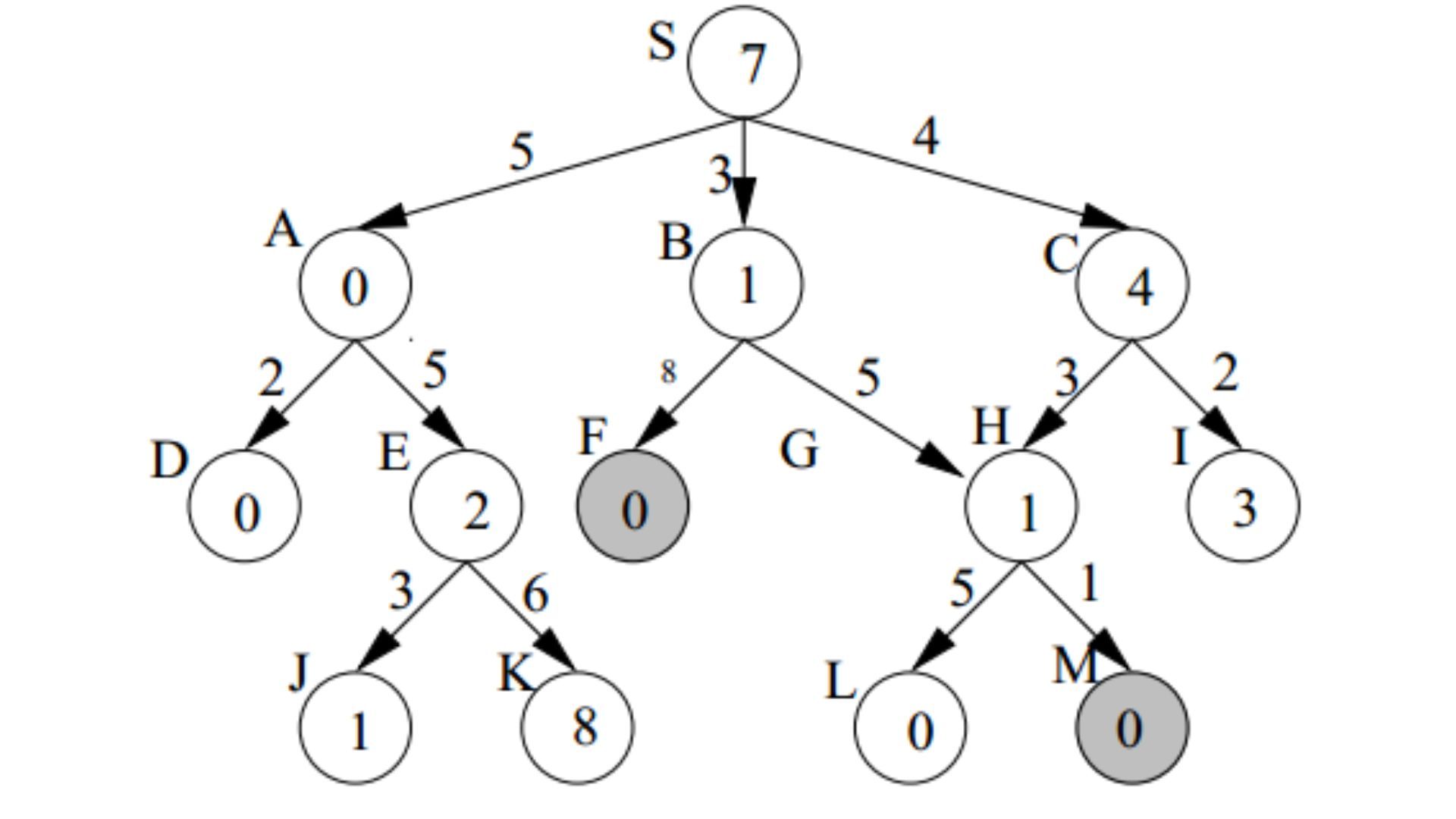
Introduction
A* is a popular pathfinding and graph traversal technique that is pronounced "A star." The programme effectively creates a path on the network that can be traversed by people.
- One of the finest and most often used methods for path discovery and graph traversals is the A* algorithm.
- This technique is often used in games and online maps to effectively discover the shortest distance.
- It resembles a best-first search algorithm in many ways.
Working of A* algorithm
A* also constructs a lowest-cost path tree from the start node to the target node, much like Dijkstra. The fact that A* utilizes a function f(n)f(n) for each node to estimate the overall cost of a path involving that node sets it apart from other search engines and makes it superior for many queries.
Using this function, A* extends the pathways that are already less expensive: f(n)=g(n)+h(n),f(n)=g(n)+h(n),where:
- Total estimated cost of route across node nn is given by f(n)f(n).
- g(n)g(n) is the cost to go to node nn.
- Estimated cost from nn to goal is h(n)h(n).
Since this is the heuristic portion of the cost function, it is somewhat of a guess.
Steps:
- Create an OPEN list. OPEN is composed of just one node at first, the start node S.
- If the list is empty, give an error message and quit.
- The node n with the least f(n) value should be moved from the list OPEN to the list CLOSED. Return success and leave if node n represents the desired state.
- Increase node n.
- Trace the route from any successor to n to S, and if it is the destination node, return success and the solution. If not, proceed to the next step.
- Apply the evaluation function f to the node for each successor node. Add the node to OPEN if it hasn't already been there.
- Repeat from Step 2
Code:
Conclusion
A-star (A*) is a powerful artificial intelligence algorithm with many applications. But it is only as effective as its heuristic function. A* is the most often used pathfinding algorithm because of its reasonable flexibility. It has found use in a variety of software systems, including game creation where characters must navigate through difficult terrain and obstacles to get to the user, as well as machine learning and search optimization.