Solving N Queens Problem using Backtracking
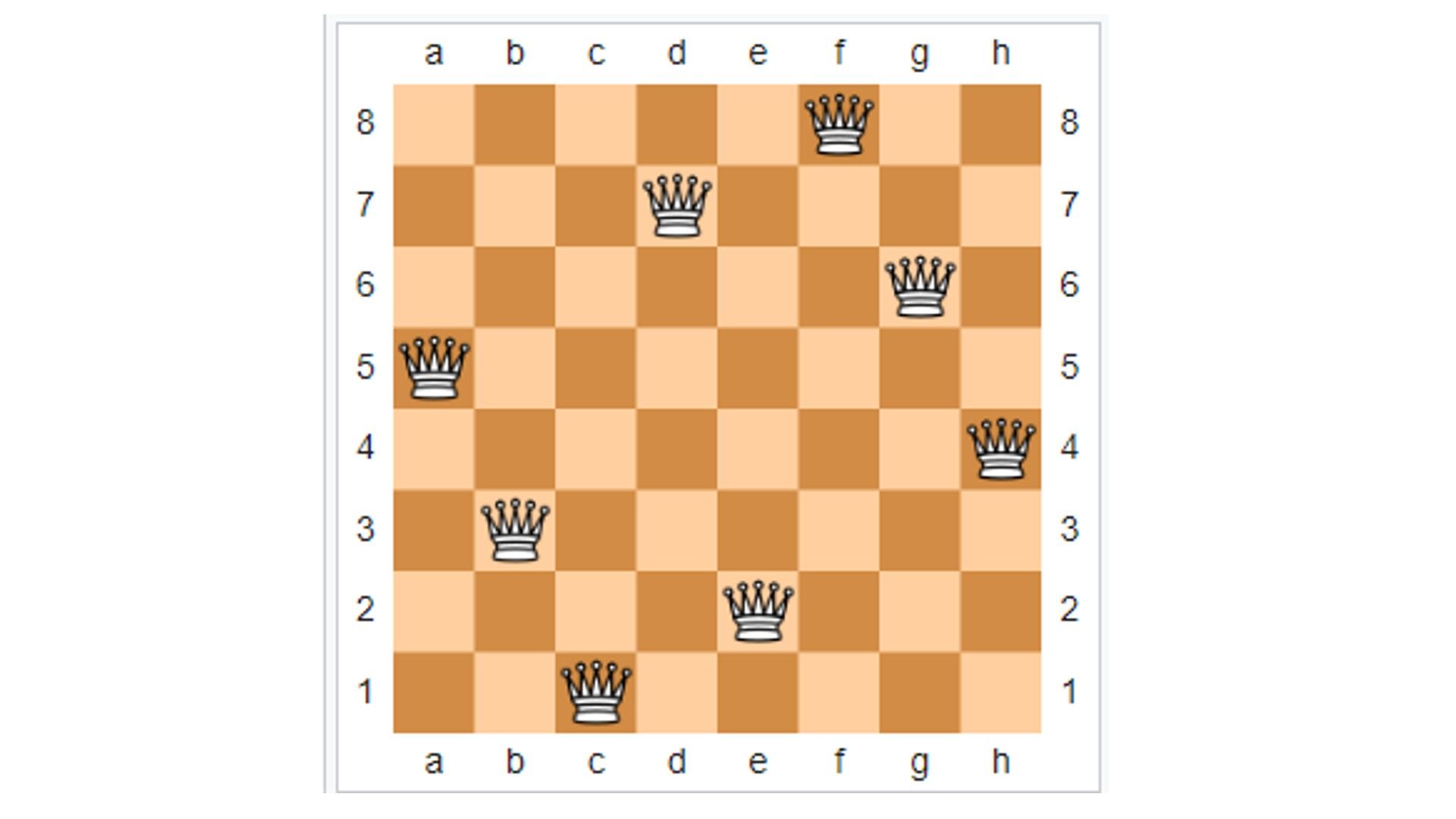
Introduction
In this article, we will study a classic Backtracking problem. This is a considerably hard problem and implements several programming paradigms. The N-queens problem is something that chess enthusiasts will find interesting. Backtracking is a nice skill to comprehend. When regressing, we begin with one possible move out of a variety of options. Then, we attempt to resolve the issue. If the problem can be solved using the chosen move, the solution will be printed. If not, we will go back and choose another move to try to solve it. We assert that there is no solution to the problem if none of the moves succeed.
Scenarios and Steps
Problem Statement: How can N queens be arranged on a NxN chessboard without any of them attacking one another?
In interviews, N is usually considered to be 4 in the problem statement so for this article we will assume it to be the same,
Input: n = 4
Output: [[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]]
Explanation: There can be two possible movesets for the posed question
You must understand how the chess queen moves in order to solve this puzzle.
- A queen can move in any direction and take any number of steps.
- The only restriction is that it cannot reverse course while moving.
- The movement of the queen makes it obvious that no two queens can be found in the same row or column. As a result, we can only put one queen for each row and column.
- By positioning a queen at a position and attempting to rule out the possibility that it is being attacked, we attempt to solve this problem.
- Each row or column gets one queen. If we notice that the queen is being attacked at the position it has selected, we move on to the next one.
- If a queen is being attacked at each position in a row, we go back and move the queen who was in that position before the current one.
- Until all N queens are successfully placed, we keep doing this backtracking and placing a queen cycle.
Code
Complexity Analysis
Since we are calculating every move and considering every single position on the chessboard for a possible move, even the optimized backtracking approach will yield a Time Complexity of O(N!) and the Auxiliary Space required will be constant O(N) since the size of the chessboard is fixed.