Bellman Ford Algorithm in detail with code
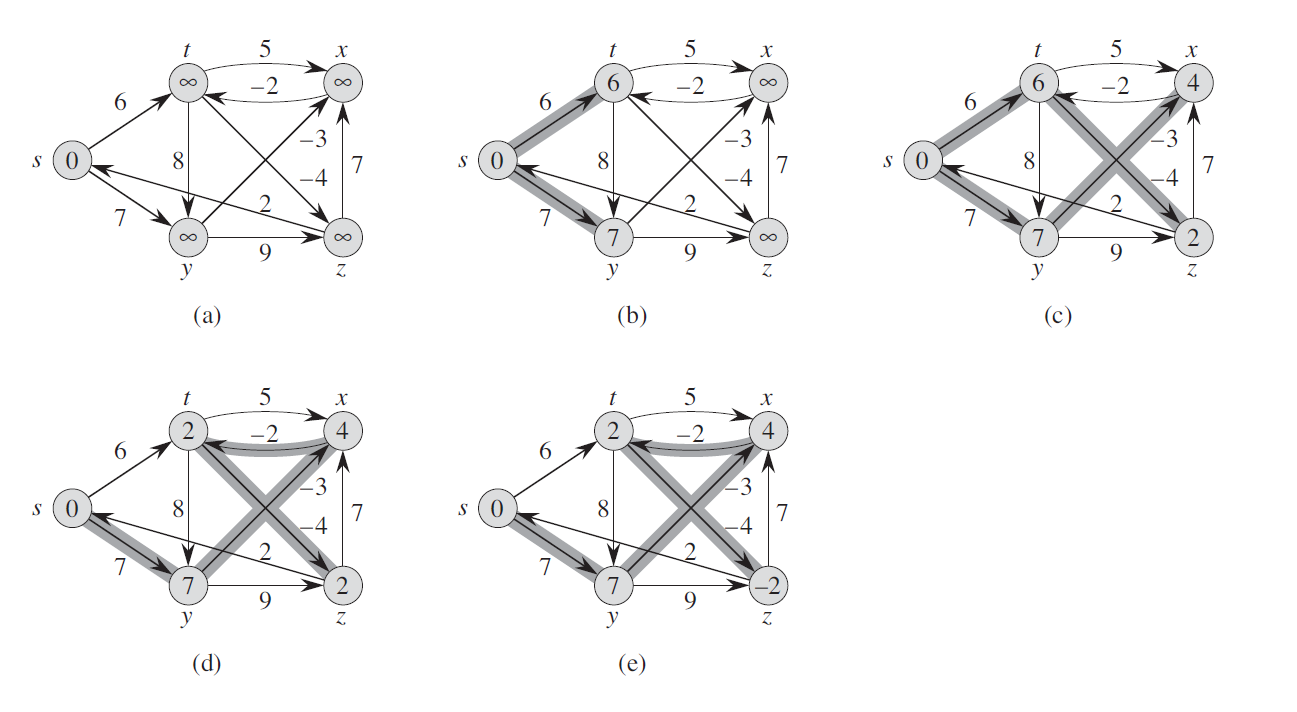
Introduction
In this article you will understand the Bellman-Ford algorithm step-by-step, using the example as a guide. C++ implementation with the code for calculating the distance from the source vertex in a weighted graph is also covered in this article.
Approach
The Bellman-Ford algorithm imitates the shortest routes in a weighted digraph from a single site vertex to all other vertices. Since it can handle graphs with certain edge weights that are negative values, it is more flexible than Dijkstra's method for the identical issue. The Bellman-Ford algorithm makes use of dynamic programming. It starts with a beginning vertex and determines the shortest paths an edge can take to reach additional vertices. The process then continues in search of a path with two edges. The bottom-up method is employed by the Bellman-Ford algorithm.
Negative Cycles
Negative weight edges can produce negative weight cycles that, by going back to the same location, shorten the overall path length. Negative weight cycles could provide the wrong answer while trying to identify the shortest path. Because they may experience a negative weight cycle that shortens the trip, shortest path methods like Dijkstra's Algorithm that cannot identify such a cycle may generate inaccurate results.
Steps
- Step 1: Make a list of every edge in the graph. If the graph is represented as an adjacency list, then this is easy.
- Step 2: The number of iterations is calculated using the formula "V - 1". The number of iterations will grow the same number of vertices since the shortest path to an edge can only be changed V - 1 times.
- Step 3: Start with a random vertex at a distance of 0 minimum. You are overstating the real lengths, hence all other nodes ought to be given a distance of infinite. Relax the vertices' route lengths for each edge u-v:
- Distance[v] = distance[u] + edge weight uv if distance[v] is larger than distance[u] + edge weight uv.
- Step 4: Alter the distance for each Edge in every iteration if the new distance is smaller than the old one. The distance covered from the beginning node to each individual node is the distance to each node.
- Step 5: You must take alliterations into account to make sure all potential pathways are taken into account. If you accomplish this, the distance you have traveled will be the shortest.
Code
Output:
Vertex : 0 1 2 3 4
Distance (from the source)
Complexity Analysis
The graph might present a worst-case scenario when you encounter a negative cycle. You would need to relax |E| * (|E| - 1) / 2 edges, (|V| - 1) number of times in a full graph with edges connecting every pair of vertices, assuming you identified the shortest route in the first few iterations or repetitions but continued with edge relaxation.
The complete graph will lead to the worst case scenario where the time complexity will be:
O(|V|^2) = O(E V). O(|V|) = O (V^3)
Applications of Bellman Ford Algorithm
- Finding weight-loss relapse cycles
- Calculate the least amount of heat that can acquire or lose during a chemical process.
- Figuring out the most effective currency conversion technique. looking at a graph to see if there are any negative weight cycles.
- Find the graph's shortest route using negative weights.
- In data networks, the idea of routing is applied.