Prim's Algorithm: Explanation, Code, and Applications
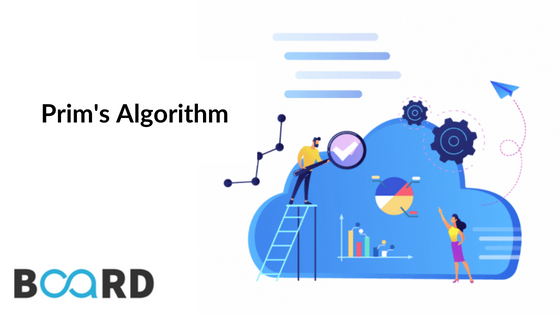
Introduction
Prim's Algorithm turns a given graph into a tree with the least possible sum of its edges. It is known as a Minimum Spanning Tree. In order to discover this least-spanning tree, it employs the greedy method. The chosen data structure affects how long the algorithm takes to run.
- The article provides a detailed explanation of Prim's algorithm as well as an algorithm walkthrough.
- We go over the method's code and talk about how long various C++ implementations of the algorithm take.
- The justification additionally included The algorithm's adjacency matrix implementation
Implementation
Prims Algorithm is a Greedy Algorithm meaning that at every step, the algorithm will make a decision depending on what is the best choice at that point.
It provides the best solution for the immediate issue and makes the assumption that the greatest solution will ultimately be found for the entire issue.
Steps:
- Pick a random vertex on the graph to begin with, then mark it as visited.
- Choose the least-weighted edge that has not yet been processed after iterating through all the visited vertices.
- Step 2 should be repeated until all nodes have been visited.
Code:
Time Complexity
The adjacency matrix implementation utilizes a nested for loop in findMST() that iterates from 0 to V. Therefore, the time complexity is O(V^2). Can be minimized depending on the data structure.
The time it takes to choose a vertex with minimum weight at each step will be reduced if the input graph's vertices are stored in a binary heap and organized by weight.
Applications
- Maze generation: The prim's approach can be used to create a maze for a weighted graph random in nature. The exit and entry nodes for the maze might be any two nodes.
- Cable laying, electric grids layout, and LAN networks, especially in cases where the graphs are dense: Can be achieved by connecting all the nodes while checking that the least amount of cable is being used for any situation. Hence optimizing.