Floyd-Warshall Algorithm and its Implementation
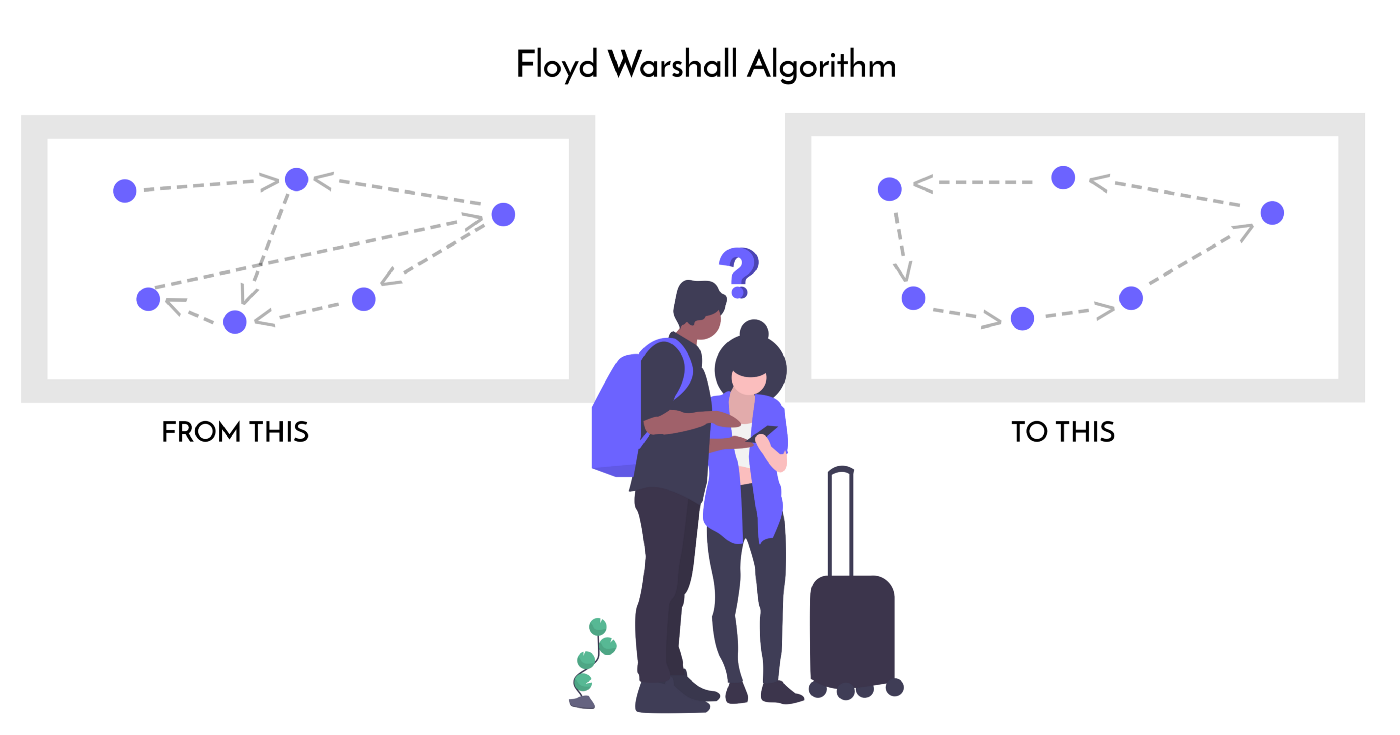
Introduction
In this article, you will learn how floyd-warshall algorithm works. We also present C++ implementation and examples to help you understand it in a lucid manner.
A weighted graph's Floyd-Warshall method is used to determine the shortest route between all of the vertex pairs. Both the directed and undirected weighted graphs may be solved using this approach. However, it is ineffective for graphs with negative cycles (where the sum of the edges in a cycle is negative). A weighted graph is one in which each edge is assigned a numerical value. Floyd's algorithm, Roy-Floyd algorithm, Roy-Warhshall algorithm, or WFI algorithm are other names for the Floyd-Warhshall algorithm.
Implementation
For a graph with N vertices:
- Set Infinity as the initial value for the shortest pathways between any two vertices.
- In order to reach a state where all N vertices are utilized as intermediate nodes, determine the shortest routes between all pairs of vertices that employ 0 intermediate vertices, 1 intermediate vertice, and so on.
- Reduce any two pairs' shortest routes from the preceding procedure.
- The shortest path will be: min(dist[i][k]+dist[k][j],dist[i][j]), therefore for each two vertices (i,j), one should really reduce the distances between this pair using the first K nodes. The shortest path that only passes through the first K vertices is represented by dist[i][k], while the shortest path between two vertices is represented by dist[k][j]. The concatenation of the shortest paths from I to k and then from k to j will result in the shortest path.
Code
Complexity Analysis
Three loops are present. The complexity of each loop never changes. Consequently, the Floyd-Warshall algorithm's time complexity is O (n3).
The Floyd-Warshall algorithm's space complexity is O (n2).
Applications:
- In a directed graph, the shortest path is determined using this Algorithm.
- To locate directed graphs' transitive closure.
- To determine real matrices' inversion.
- To determine if a bipartite undirected graph exists