Abstraction Vs Encapsulation in Java
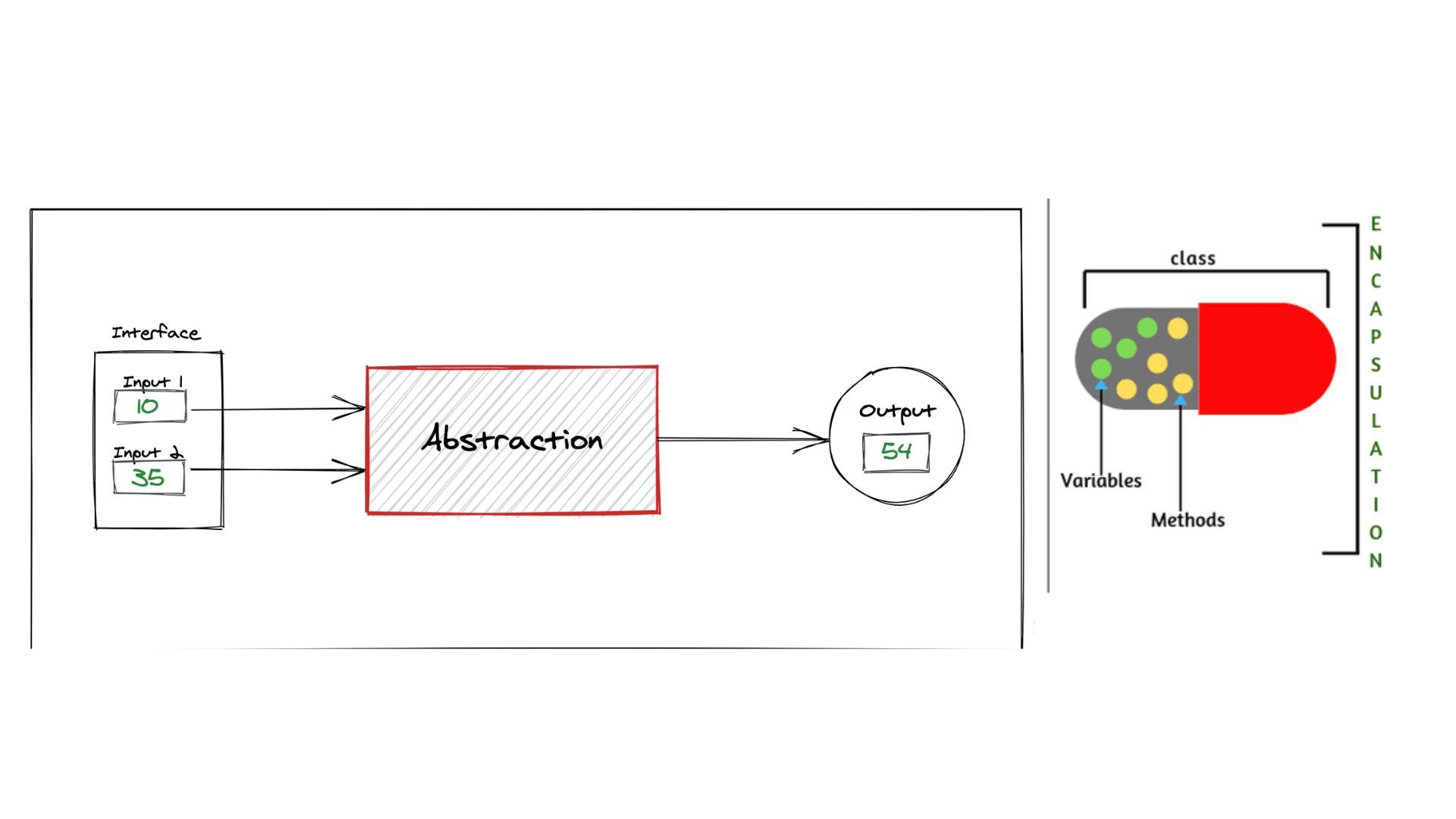
Introduction
Object-oriented programming is at the core of Java, which follows OOPs concepts. OOPs provides two other features, which are abstraction and encapsulation. Although they seem very similar, their concepts and implementations are quite different.
A prime difference between abstraction and encapsulation is that abstraction hides the complexity of the code, while encapsulation hides its internal functions.
What is Abstraction in Java?
Abstraction is the process of hiding unnecessary information from a user while displaying essential information that is valuable to them.
It does not display trivial or non-essential units to the user. By using interfaces and abstract classes, you can accomplish this. A user can only access the methods of an interface.
A TV remote, for example, only shows the keys to the user, not the codes on which it operates since those are of no use to them.
Code:
Output:
What is Encapsulation in Java?
This is a feature of OOP that binds data into a single unit called a class. This mechanism provides the ability to hide data. From a security point of view, it is also essential.
Technically, an encapsulated class serves as a protective shield that prevents other classes from accessing its variables and data. It is also known as data hiding because the data in a class is hidden from other classes.
To encapsulate a class, declare all variable names as private, and implement methods to set and retrieve variable values in the class.
Code:
Output:
Key Difference Between Abstraction and Encapsulation
- Encapsulation wraps code and data for unnecessary information, while Abstraction presents only useful data.
- Encapsulation focuses on how it should get done, while Abstraction focuses on what should get done.
- The Abstraction technique hides complexity by providing a more abstract picture, and the Encapsulation technique hides work within the system so it can get changed in the future.
- Abstraction allows you to separate the program into many independent parts, while Encapsulation enables you to change it as per your requirement.
- Abstraction addresses design problems, while Encapsulation addresses implementation problems.
- Abstraction hides unnecessary details in code, while Encapsulation makes it easier for developers to organize the entire program.
Conclusion
There is a subtle difference between abstraction and encapsulation, but they do go hand in hand within many programming languages. Both help programmers keep code more organized by separating unrelated items within the code. They are both beneficial to the program's overall structure.