Understanding Polymorphism in Java
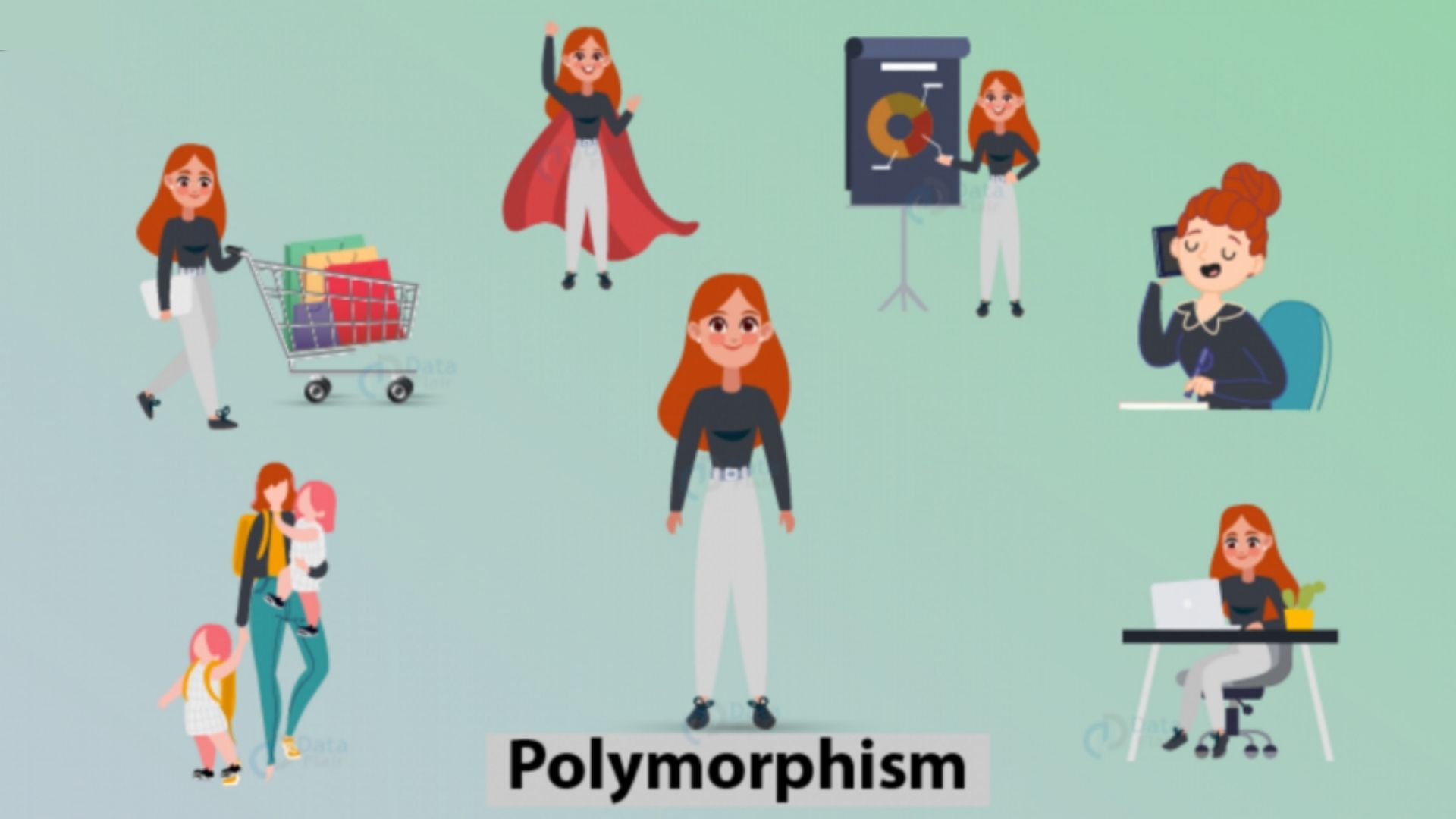
Introduction
Polymorphism describes an object's ability to assume different forms. Depending on what type of object a method gets passed to, a class with polymorphism can offer different implementations of that method.
Object-oriented programming languages, such as Java, support polymorphism, which means you can perform similar tasks in multiple ways.
Technically speaking, polymorphism in Java allows multiple implementations by defining a single interface.
What is Polymorphism?
The word polymorphism originates from two different Greek words- poly and morph. "Poly" means numerous, while “Morphs” means forms.
Polymorphism means having innumerable forms. Thus, polymorphism is one of the most important features of Object-Oriented Programming.
What is Polymorphism in Java?
In Java, polymorphism refers to the ability to perform the same action in different ways. A language without polymorphism would not be an 'Object-Oriented Language' but rather an 'Object-Based Language'. Ada is an excellent example of this.
Because Java supports polymorphism, it is an Object-Oriented Language. There is polymorphism when several classes are related, i.e. when there is inheritance. In Java, inheritance is a powerful feature. Inheritance in Java allows classes to share properties and attributes.
As a result of polymorphism, we can use these inherited properties for different purposes in Java. In this way, we can accomplish the same action in various ways.
Types Of Polymorphism In Java
There are two types of polymorphism in java programming:
Compile time polymorphism and run time polymorphism. Static and dynamic polymorphism are also terms used to describe this java polymorphism.
1. Static Polymorphism (Or Compile-Time Polymorphism)
Similarly to many other OOP languages, Java polymorphism allows classes to have multiple methods. While the names of the methods are the same, the parameters differ. Polymorphism at the static level gets represented by this.
As a result of method overloading, this polymorphism gets resolved at compile time. (Compile-time Polymorphism) Java uses static polymorphism to determine which method to call.
Static polymorphism involves objects behaving differently for the same trigger. Hence, a class may contain multiple methods.
- There should be a variation in the parameter number.
- There should be a difference in parameter types.
- Parameters get ordered differently.
For instance, one method accepts a string and a long, and the other will accept both. The API finds this sort of ordering challenging to understand. Method signatures differ due to the difference in parameters. Java compilers know which method should get called.
2. Run-time polymorphism (or dynamic polymorphism in Java)
Polymorphism in Java is a form in which the compiler doesn't decide which method to execute. Java Virtual Machine (JVM) is the program that executes the process at runtime. In Java, dynamic polymorphism refers to overriding methods at runtime.
Superclasses call their overridden methods through their reference variables. In contrast to static polymorphism, dynamic polymorphism occurs among different classes. Java's dynamic polymorphism enables runtime polymorphism through overriding methods.
Java Polymorphism Example
Hope this article gave you a clear understanding of Polymorphism in Java. To learn about similar topics visit Discussion Forum and Board Infinity Blog.