Introduction to StringBuffer in Java
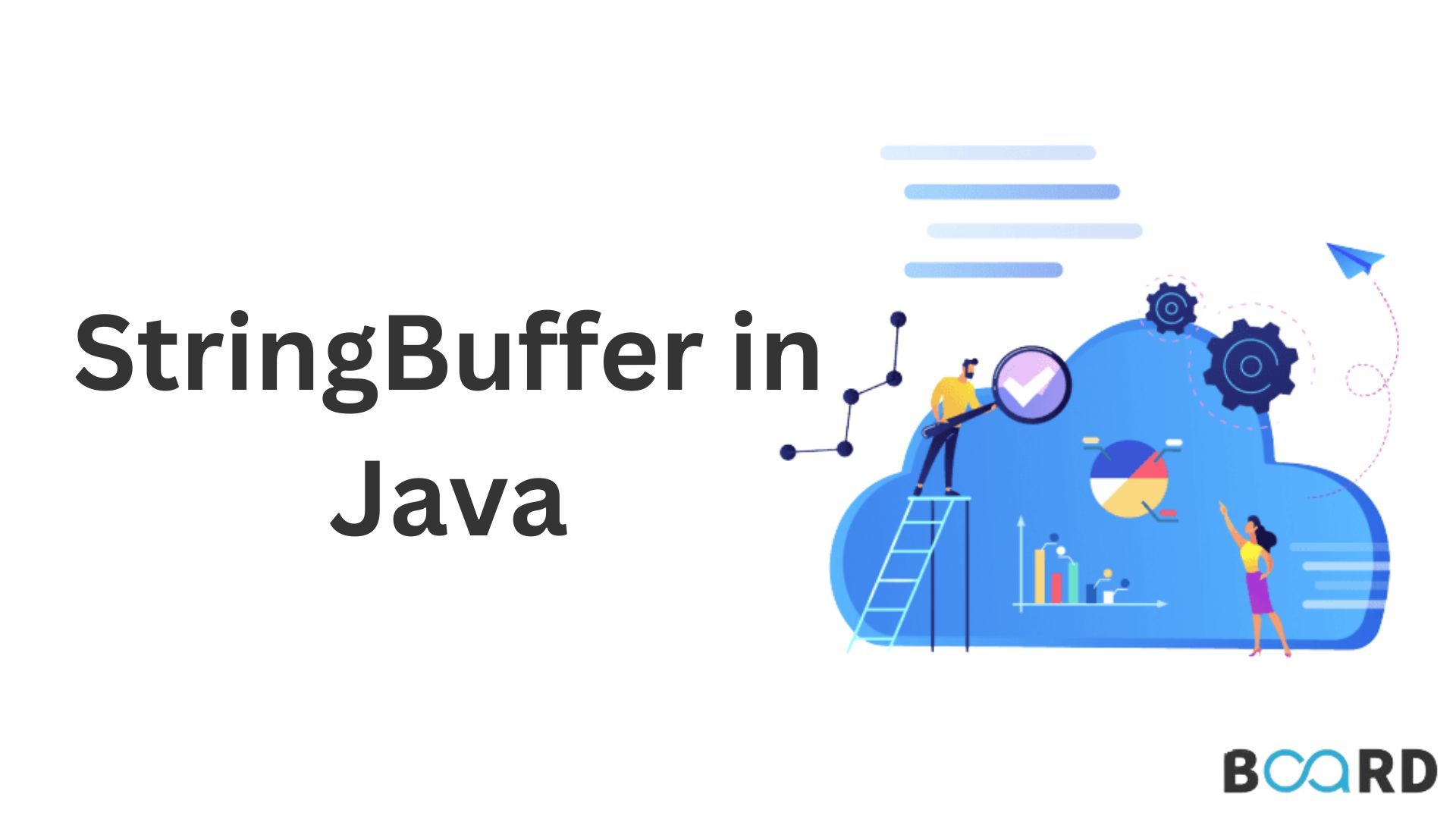
Introduction
To build a mutable string object, utilize the StringBuffer class. It implies that it can be modified after creation. It stands for a character sequence that may be written and grown. Similar to Java's String class, which is also used to create strings, stringbuffer objects can be modified. Therefore, the StringBuffer class is used when we need to modify our string frequently. Additionally, it is thread safe, meaning that only one thread at a time can access it. StringBuffer has four constructors defined.
- StringBuffer(): It produces a string buffer that is empty and sets aside room for 16 characters.
- StringBuffer(int size): It produces a blank string and accepts an integer input to specify the buffer's capacity.
- StringBuffer(String str): From the specified string, a stringbuffer object is created.
- StringBuffer(charSequence []ch): From the charSequence array, a stringbuffer object is created.
Creating a StringBuffer Object
Using the StringBuffer class, we create a string buffer object in this example and test its mutability.
StringBuffer vs. String differences
In this example, we create and edit instances of the StringBuffer and String classes, but only the StringBuffer object is altered. See the illustration below.
Explanation:
Due to String objects' immutability, the output is what it is. Since the same String object won't be changed by concatenation, StringBuffer produces mutable instances instead. Consequently, it is modifiable.
Important methods of StringBuffer class
Some of the most frequently used methods of the StringBuffer class are those listed below.
append()
Any type of data's string representation will be placed at the end of StringBuffer object using this function. Multiple variants of the add() function are overloaded.
Each parameter's string representation is added to the StringBuffer object.
insert()
One string is inserted into another using this procedure. Here are a couple variations on the insert() technique.
Here, the second argument's string representation is entered into the StringBuffer object while the first parameter specifies the index at which the string will be inserted.
reverse()
A StringBuffer object's characters are reversed by this method.
replace()
By using the supplied start index and finish index, this function substitutes the string.
capacity()
The StringBuffer object's current capacity is returned by this method.
There are 16 characters reserved for empty constructors. Consequently, the output is 16.
ensureCapacity()
The minimum StringBuffer object capacity is guaranteed using this approach. There won't be any change in capacity if the parameter to the ensureCapacity() function is smaller than the current capacity. The ensureCapacity() function will adjust the current capacity according to the following rule if the parameter is more than the current capacity: