What is Immutable Class in Java?
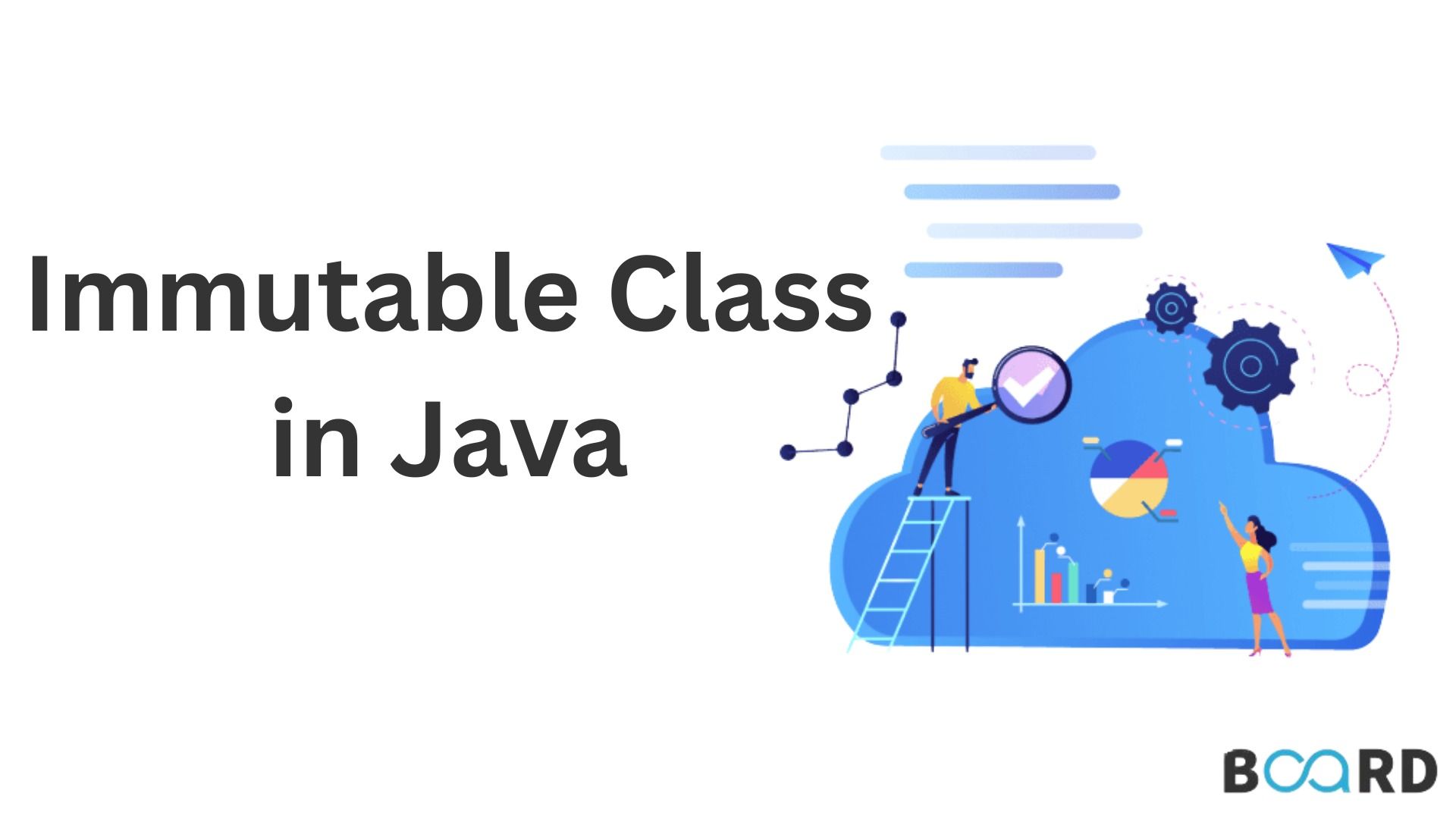
Introduction
Something is said to be immutable if it cannot be altered. An immutable class in Java is one whose state cannot be altered after creation. In this article, we will see an example of how to construct an immutable class and it will serve as your guide for making classes immutable. Although the Java documentation provides a number of recommendations for developing an immutable class, we'll try to comprehend it better.
Usage of Immutable Classes
The "must-have" requirement for any software application today is that it be distributed and multi-threaded. Multi-threaded applications are notoriously difficult for developers to create because they require them to safeguard their objects' state from concurrent modifications from multiple threads.
To accomplish this, developers typically use Synchronized blocks whenever they change an object's state. Each time a state is changed, a new instance is created, hence immutable classes prevent states from ever-changing. Because each thread would utilize a distinct instance, developers wouldn't have to worry about concurrent updates.
Creating an immutable class
These actions must be taken to make a class immutable:
- Declare that the course is over.
- All fields should be final and confidential.
- Make sure the class only returns the copied value to the calling code for any mutable fields.
- Never offer any setter methods.
Why are all the aforementioned steps necessary to make a class immutable?
- We must make sure that none of the class methods are replaced by the subclasses. Making a class final guarantees that it cannot be overridden.
- Making the function private and using a factory method to produce an instance of the class is a more advanced strategy. All of the fields are made private to prevent changes outside of the class. This will prevent us from accidentally changing the field.
- By constructing a protective duplicate of the object and then only returning that copy to the caller code, we ensure that no other external object might alter the values in any of the changeable fields.
- The purpose of an immutable class is to prevent state changes, hence we do not supply any setter methods because they are typically used to modify an object's state.
Steps for making a class immutable
- Declare that the course is over
- Finalize all of its fields
- Classes must create defensive copies of all mutable fields and only ever return the copies to the calling code.
- Disallow the use of setter methods.
Let's examine an illustration of an immutable class:
In the class, there are three fields
- The initial field is of the type Integer.
- The following field is a String.
- A date field is the third one down.
The Date class is changeable although the String and Integer classes are immutable. As a result, when we assigned the Date to the class field, a new Date object was generated.
Conclusion
When utilized properly in a multi-threaded context, immutable classes offer several benefits. The sole drawback is that they use more memory than standard classes since each time they are modified, a new object is produced in memory. However, developers shouldn't exaggerate this drawback because it is insignificant in comparison to the benefits that these types of classes offer.
Last but not least, an object is said to be immutable if it can only display one state to other objects, regardless of how and when other objects use its functions. In that case, it is thread-safe by any standard.