Introduction to Static Keyword in JAVA
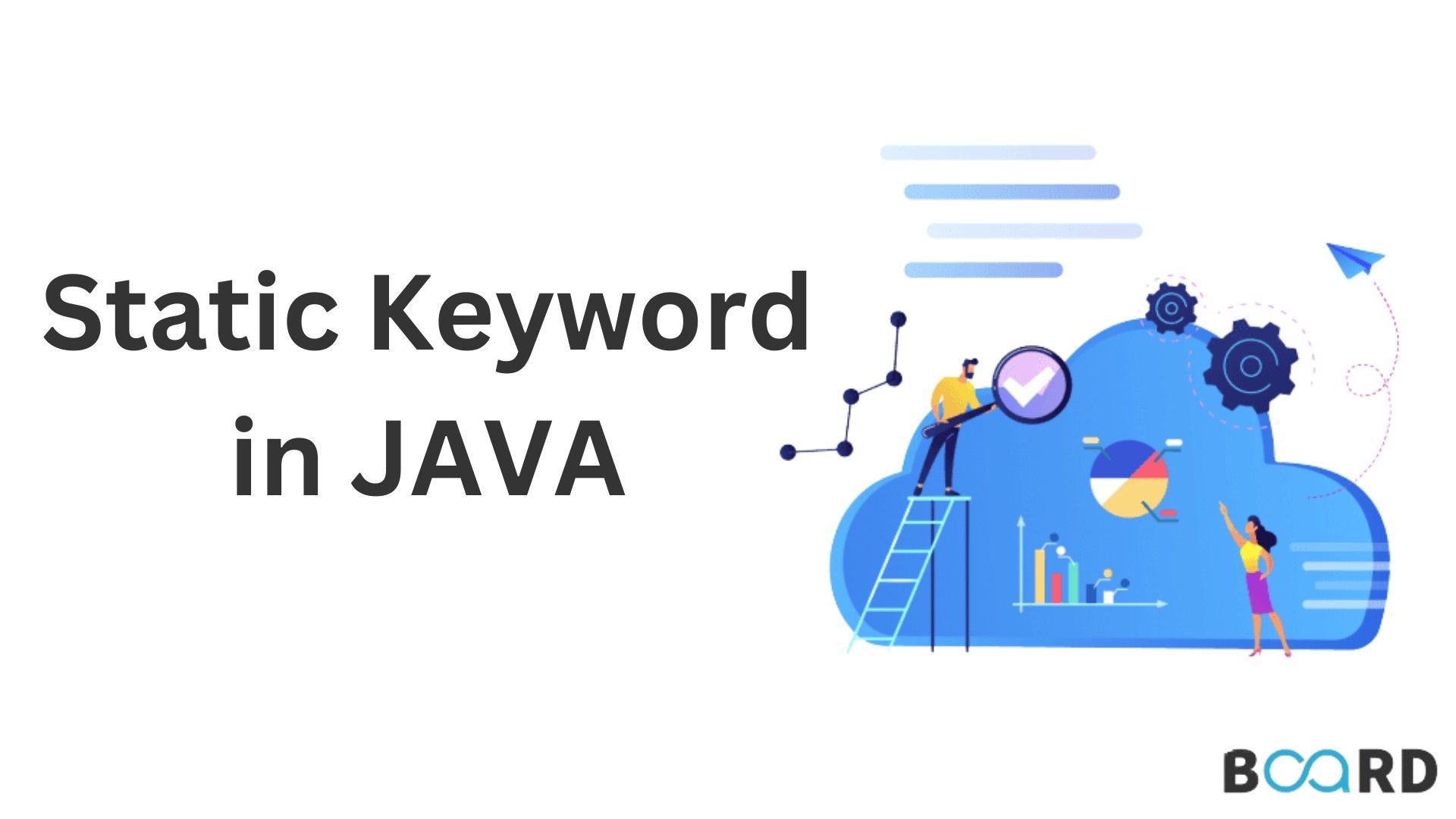
Introduction
The Java Static Keyword and Its Use in Variables, Methods, Blocks, and Classes are Explained in this Tutorial. Additionally explains the distinction between static and non-static members. To describe the scope and behavior of its classes, variables, methods, etc., Java allows a variety of declaration types. The terms final, sealed, static, etc. are some examples.
When they are used in a Java application, each of these declarations has a distinct meaning. As this course moves further, we will examine each of these keywords. Here, we'll go through the specifics of one of Java's most significant keywords: "static."
Basics of Static Keyword
The keyword "static" can be used to designate a member in a Java program to be static before it is declared or defined. When a member of a class is marked as static, it simply indicates that all instances of the class share the member without producing a copy for each instance.
As a result, the following members can have static applied to them in Java:
- Classes
- Blocks
- Methods
- Variables
When a member is marked as static, it can be used directly without an object. This indicates that the static member is available and active before a class is created. The static member remains clearly active after the class object exits scope, unlike all other non-static members of the class that vanish.
Static Variable in Java
The term "Static Variable" refers to a class member variable that has been designated as static. Memory is only allocated once when a variable is declared static, not each time a class is created. So you don't need a reference to an object to access the static variable.
Code Example:
A and B are two static variables used in the aforementioned application. Both in "main" and the function "printStatic," we change these variables. Keep in mind that even when the function's scope expires, the value of such static variables are maintained throughout the functions. The values of two functions' variables are displayed in the output.
Why Are Static Variables Necessary and How Can They Be Used?
The best uses for static variables are programs that require counters. As you are aware, counters will produce inaccurate results if specified as regular variables. For instance, let's imagine that in an application that contains a called "vehicle,", you have a regular variable set as a counter.
The standard counter variable will thus be initialized each time we construct a vehicle object. Regardless of whether the counter variable is a static or class variable, it will only be initialised once when the class is created.
Later, this counter will be increased by one for each instance of the class. Contrary to a typical variable, in which the counter is constantly at 1, its value is not increased with each usage.
Therefore, even if you produce 100 instances of the class "car," the counter, when implemented as a regular variable, will always have a value of 1, as opposed to displaying the proper count of 100 when implemented as a static variable.
Given below is another example of Static counters in Java:
The application mentioned above makes clear how the static variable functions. The static variable count has been defined with an initial value of 0. The static variable is then increased in the class' function. Three counter-class objects are produced in the main function. The static variable's value is displayed in the output each time the counter object is generated. We can see that every time an object is created, the static variable's value is increased rather than reinitialized.