Understanding Multiple Inheritance in Java
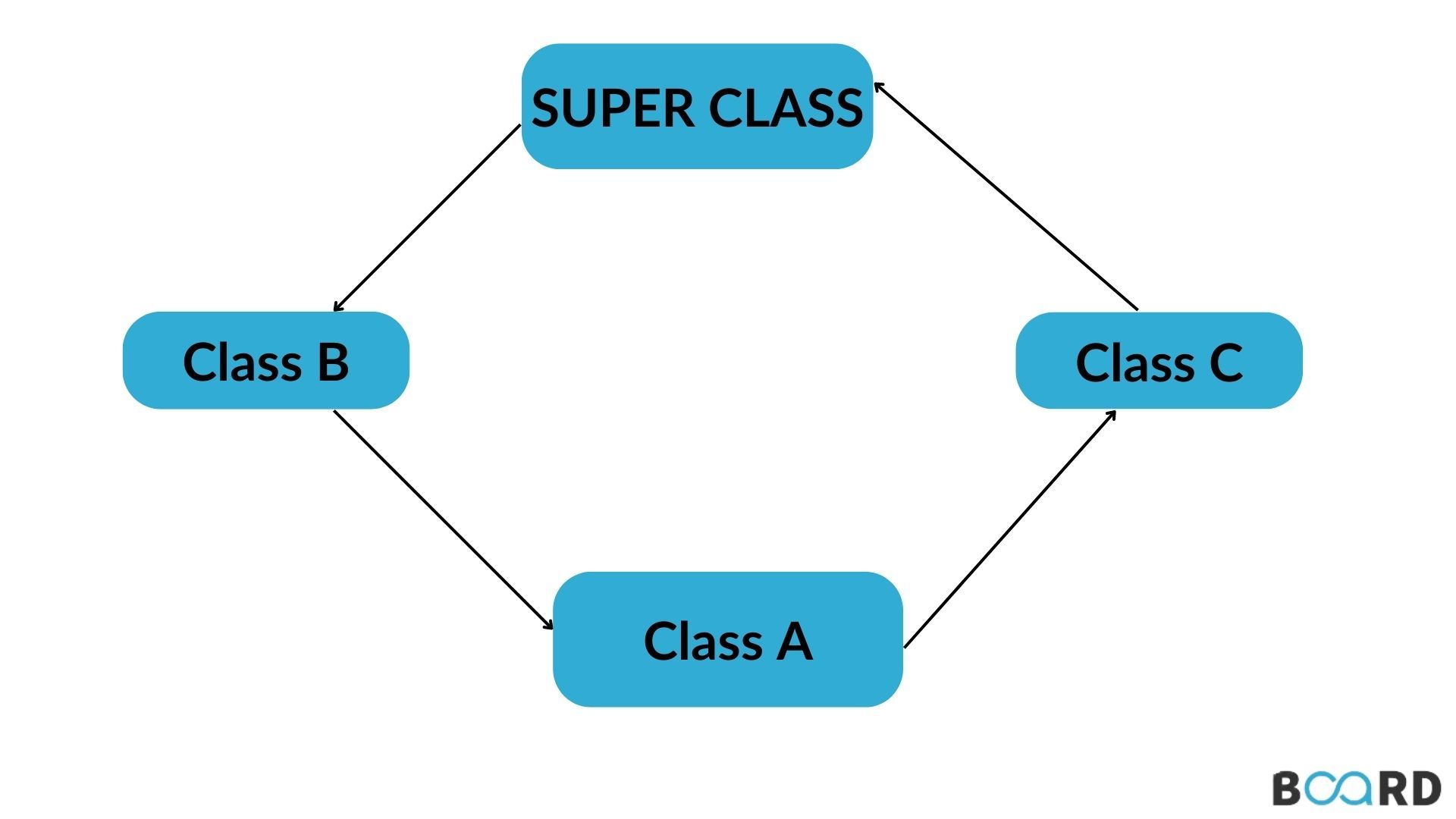
Multiple inheritance allows you to inherit properties or behaviors from multiple classes at once. Java developers often run into the problem of multiple inheritance. The purpose of this post is to explain what it means, how it gets implemented, and why you would want to use it.
What is meant by Multiple Inheritance?
Multiple inheritance occurs in some object-oriented programming languages and refers to how a class or an object inherits characteristics from multiple parent classes. As opposed to single inheritance, which allows objects and classes to inherit from a single-parent class.
In simple words, Multiple inheritance means creating a single class with multiple superclasses.
Java does not support multiple inheritances like C++ or most other popular object-oriented programming languages.
Why Java Doesn't Support Multiple Inheritance?
The Java language does not support multiple inheritances in classes because it can result in a diamond problem, whereas there are better ways to achieve the same result than using multiple inheritances.
Consider the situation where class B extends class A and class C, and both classes have the same display() method. It is now impossible for java compilers to decide which display method to inherit.
To prevent such situations, Java does not allow multiple inheritances. Java has overcome the problem of multiple inheritances by allowing classes to implement one or more interfaces.
Then, How to achieve Multiple Inheritance in Java?
Java allows Multiple Inheritance via Interface.
It is possible to avoid Multiple Inheritance by using interfaces. Due to the lack of multiple inheritance support in Java programming language, interfaces are a good choice. Unlike class hierarchy, interfaces are not part of the class hierarchy. Any class can implement an interface.
Java- Interface Implementation.
The following bit of code will help you understand how to implement interfaces in java and achieve the benefits of Multiple Inheritance.
Output:
Conclusion
Multi-inheritance is one of the most important concepts in object-oriented programming and can get achieved through Java interfaces. We can achieve multiple inheritances in Java by implementing interfaces in our classes and extending the functionality of Java objects.