Understanding Java Comparator Interface
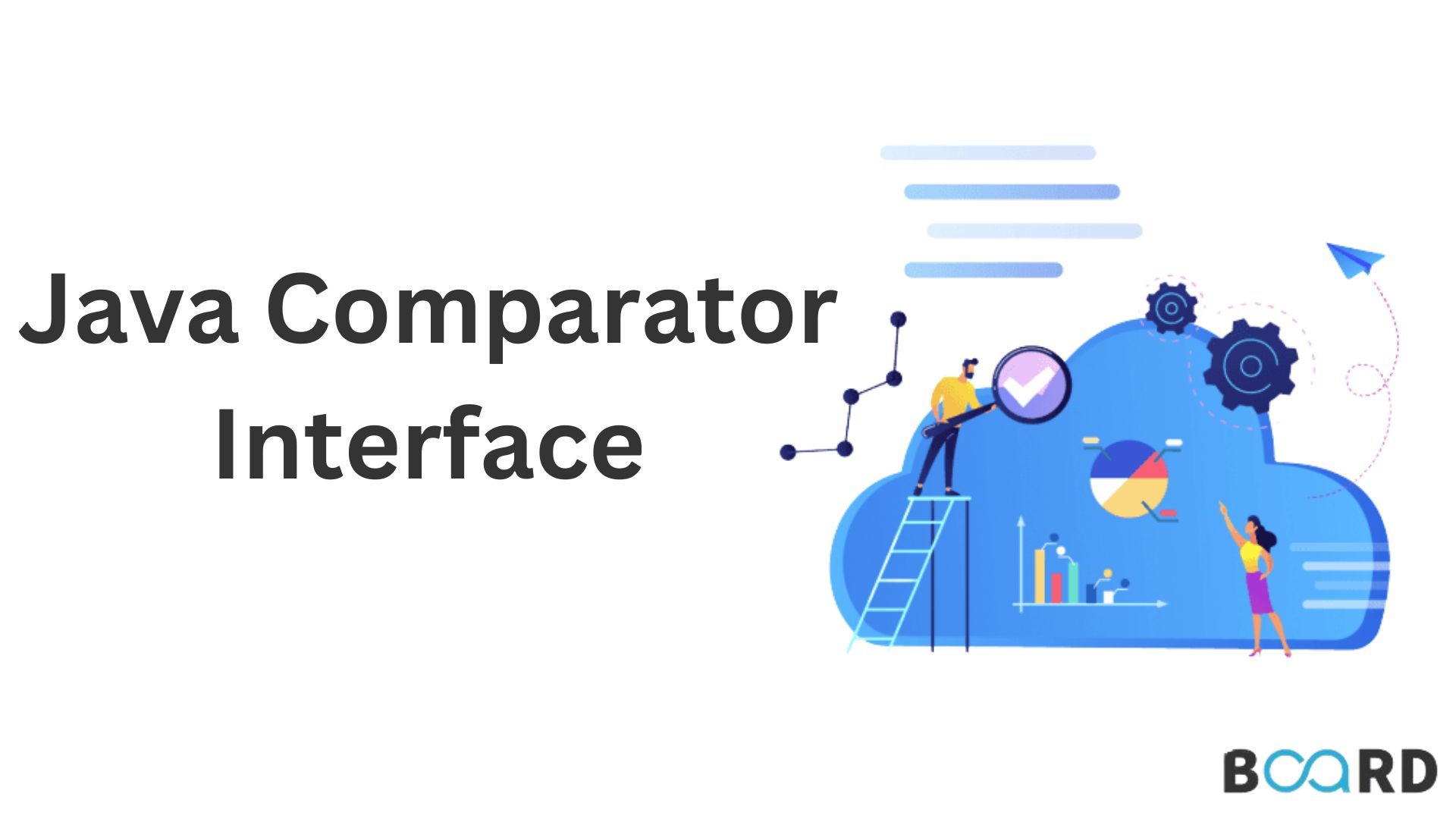
Java Comparator Interface Definition
The Java Comparator interface, java.util.Comparator, allows you to compare two objects in order to sort them using Java's sorting functionality. For example, if you want to sort a Java List, you can pass it to a Java Comparator. During sorting, the Comparator compares the List objects. As opposed to Java Comparable, Java Comparator has a different interface.
A Comparator maintains a list of objects getting compared, while a Comparable maintains a list of getting compared objects. Package java.util contains the Java Comparator interface. Java Comparator has the following syntax:
In comparison(), two objects are passed to the Comparator implementation to compare. When comparing two objects, compare() returns an int that indicates which was larger. This return value has the following semantics:
- Objects with negative values are smaller than objects with positive values.
- 0 indicates that both objects are equal.
- If the value is positive, then the first object is larger than the second.
Java 8 Comparator Example
The following example shows how the List elements can get sorted based on the Students's age and name.
Conclusion
Now you have gone through a simple Comparator class example in Java and you should have a fair idea of how to use comparator example and the syntax to implement it. You can also try to solve new Comparator examples at your finger tips.