What is Composition in Java?
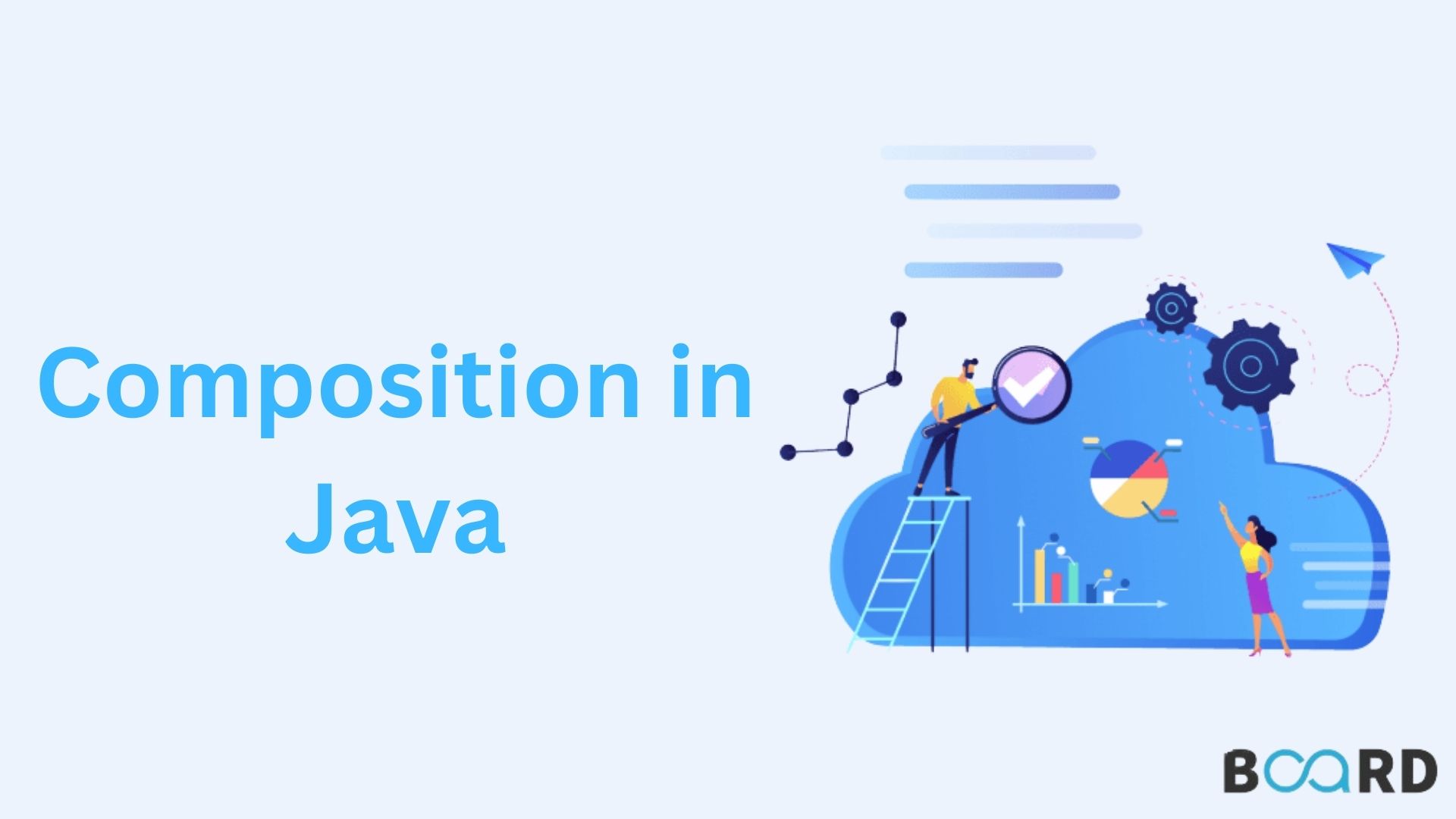
Introduction
Composition is a connection that depicts a relationship between a component and a whole in which a part cannot exist without a whole. Java uses the composition design method to implement a connection.
Implementation of Composition in Java
Composition allows us to reuse code in the same way that Java inheritance does. An instance variable that makes references to other objects is used to create the composition. To put it another way, composition refers to a reference between two or more classes that use instance variables. In contrast to an "is-a" relationship, which is inherited, we also refer to composition as a "has-a" relationship frequently.
For instance, a classroom has a blackboard, or to say it another way, a classroom has a blackboard. Hence, the board "belongs to" the classroom.
Why composition over inheritance?
There is a considerable degree of flexibility in composition. Private member objects of the new class are normally unavailable to client programmers that use the class. This enables us to alter those elements without affecting the client code that is already in place.
Additionally, you may alter the member objects while your program is running to modify its behavior on the fly. This flexibility is not possible with inheritance since classes constructed using inheritance must be subject to compile-time limitations.
Benefits of composition
Composition is important because it allows us to reuse only the parts we actually need while controlling which additional objects are visible to client classes. When necessary, composition enables the construction of back-end classes.
Here is an example of composition in Java:
Code
OUTPUT