A quick guide to Access Modifiers in Java
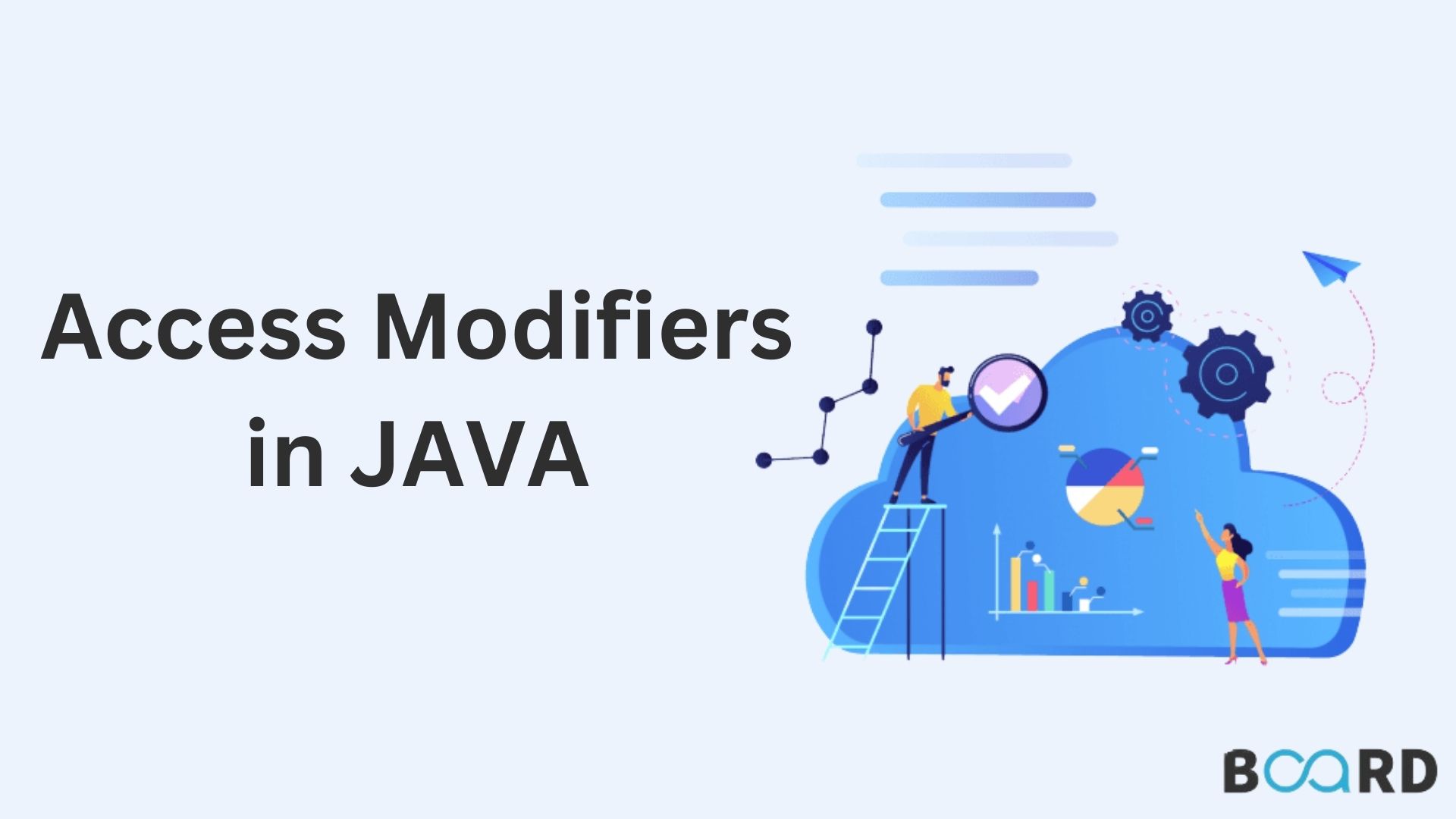
Introduction
When you are programming a Java program, you will be dealing with actions, triggers, and events all the time. Access modifiers help you manage program access in these three different ways.
Access modifiers in java have their importance in programming. It is a very important concept that can be used while creating classes and objects. Understanding the access modifier of classes and objects will help you in making them effective to use.
This blog will help you to understand what are the access modifiers in java and how they can help you control your applications.
What Are Access Modifiers In Java?
Access modifiers in java are keywords that control the field visibility, method, and constructor visibility.
Additionally, access modifiers enable other variables in the class or package to access variables (methods and fields) of the same class. These access specifiers/modifiers ensure that objects are encapsulated and reusable.
There are four types of access modifiers in Java: public, protected, default, and private.
#1. Private:
The private modifier can only be accessed inside a class, not outside.
The following code will help understand the implementation of the Private access modifier in Java
#2. Default:
Default modifiers are only accessible within a package, not from outside. Furthermore, if we don't specify the access modifiers, default modifiers will get applied.
This code demonstrates the Java implementation of the Default access specifier in java.
#3. Protected:
With the child class, we can access the protected modifier within and outside of the package. Without a child class, we cannot access it from outside the package. Inheritance is necessary to access it outside of the package.
You can use the following code to understand how Java implements the Protected access modifier.
#4. Public:
Public modifiers are accessible from anywhere. The public modifiers can get accessed within or outside the class, along with within and outside the package.
Here is a Java code example that illustrates how Public access modifiers get implemented.
Conclusion
In conclusion, modifiers are the Java keywords that describe which and how a method or variable is accessed by program code. Therefore, they are very important in writing quality code. The java modifiers are: public, private, and protected.
Java access modifiers allow us to control the visibility of classes and methods. these statements are also called annotations.