Print Fibonacci Series in JAVA
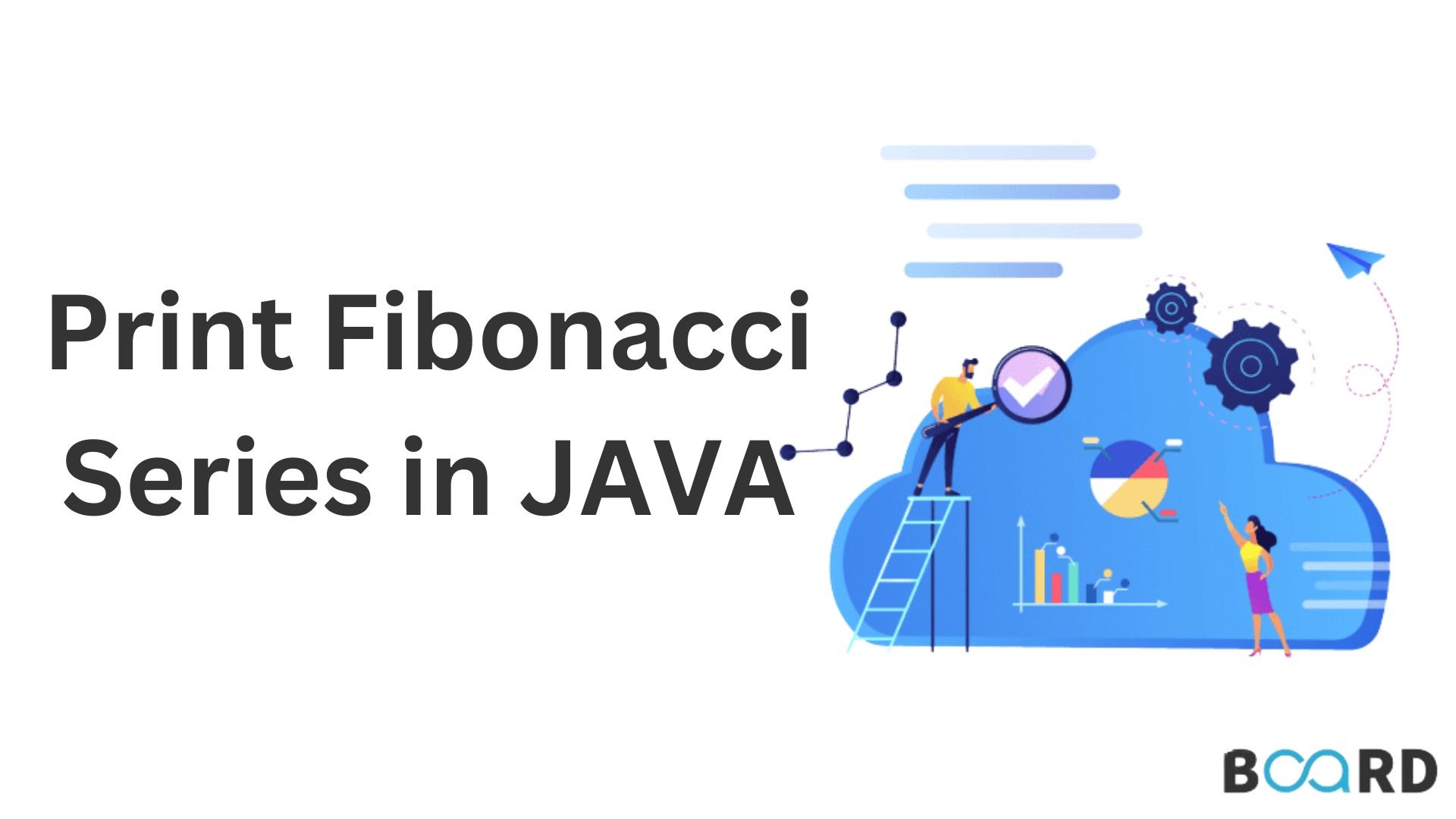
Introduction
In Java, the Fibonacci series is a program that returns a Fibonacci series of N numbers when given an integer input N. To solve a Fibonacci Series problem, it is essential to understand what a Fibonacci Series is.
What is a Fibonacci series in Java?
The Fibonacci series in Java consists of numbers, with every third number being the sum of the two previous numbers. Here is an example of the Fibonacci series
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ... n
Here, adding the two last numbers leads to the following number. i.e., adding the two numbers before 13 results in 13, hence (5+8). In the same way, 21 can be obtained by adding two numbers before it (8+13), Furthermore, 34 is (13+21).
Ways to Display Fibonacci Series in Java
Fibonacci series programs can be written in Java in two ways:
Finding Fibonacci series in Java: Without Recursion
Finding Fibonacci series in Java: With Recursion
Code Sample
Here is the code that can display the Fibonacci series until a specific value is reached