Understanding Wrapper Class in Java
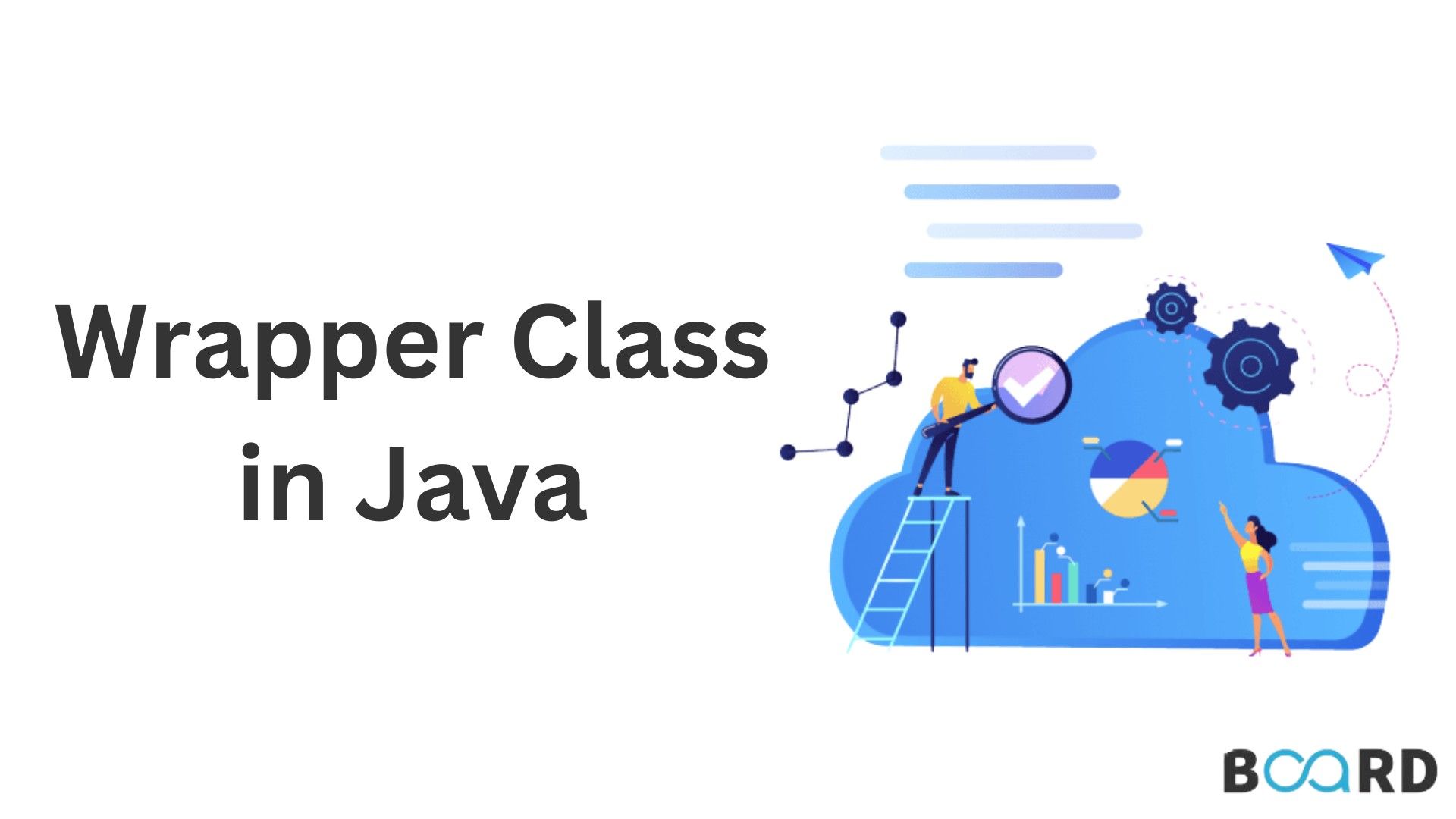
Introduction
Java provides us with a wrapper class in which objects wrap up or contain primitive data types. When an object of a wrapper class is created, it has fields that accommodate primitive data types. In simple words, we can say that an object of the wrapper class can wrap primitive values.
Why do we need a wrapper class?
- A wrapper class can convert primitive data types into objects.
- The Java.util class can handle only objects and therefore wrapper class can be helpful in this scenario also.
- The collection framework provides data structures like Arraylist and Vector that can store only objects.
- Objects must support synchronization in multithreading.
Concept of autoboxing and unboxing:
Let us understand the meaning of autoboxing and unboxing in Java:
Autoboxing
It is defined as the automatic conversion of primitive data types into objects of the corresponding wrapper classes. For example, int to Integer, double to Double, etc.
Let us consider the program below:
Source Code:
Output:
Unboxing
Unboxing is defined as the reverse process of autoboxing. It automatically converts an object of a wrapper class to its primitive type equivalent. For example, conversion of double to Double, etc.
Now let us consider the program below:
Source Code
Output:
Custom wrapper class in Java
The java wrapper class is responsible for wrapping out the primitive data types. Previously, we discussed inbuilt wrapper classes. Now we will see how we can create a custom wrapper class in Java.
Source Code:
Output:
Output description:
As you can see in the output, we have wrapped three integer values by creating a custom wrapper class.
Conclusion
In this article, we studied wrapper classes in Java. I hope this article will help you to improve your knowledge in the field of Java.