Understanding Overloading in Java
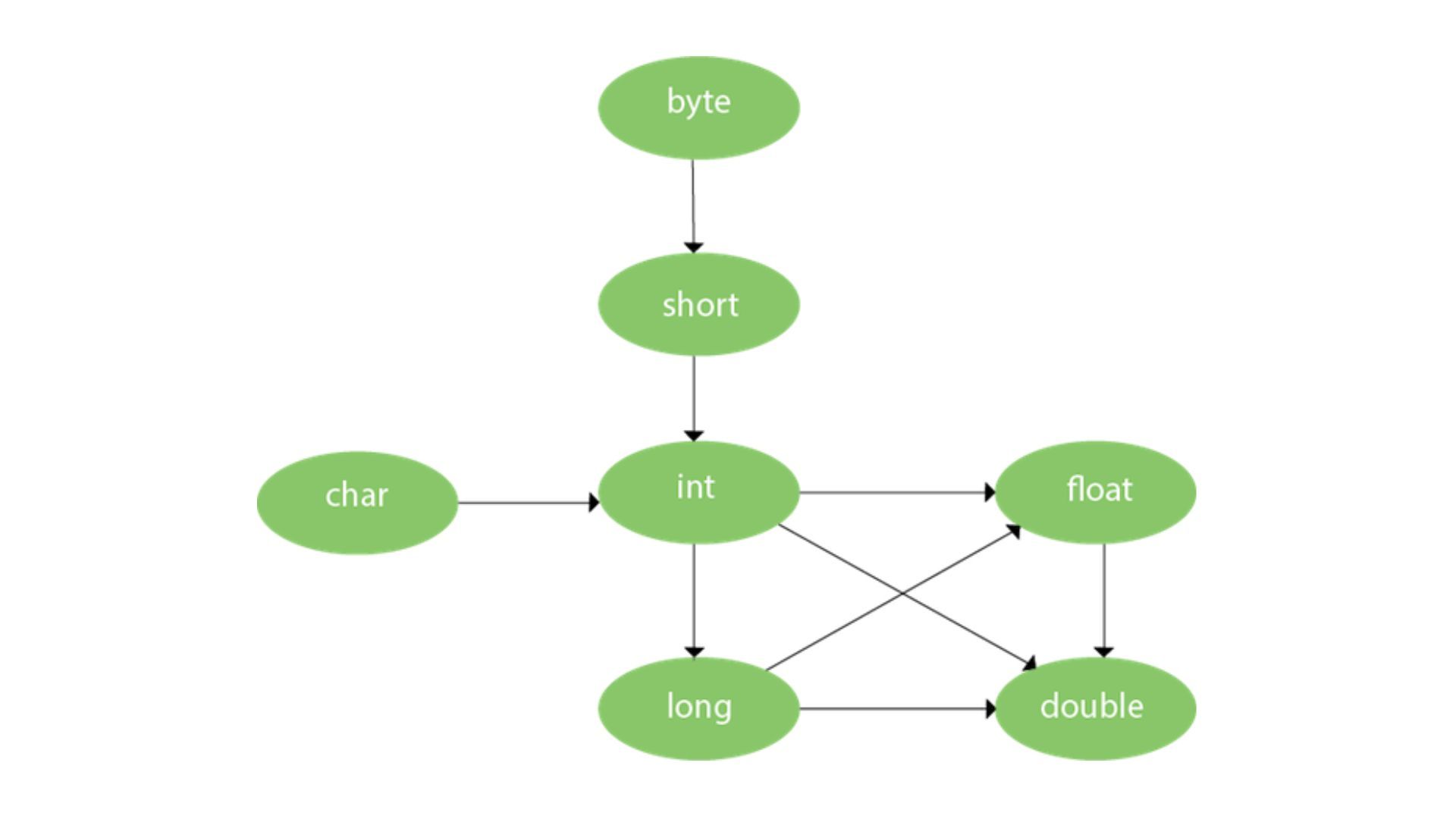
Introduction
If you are a programmer, especially if you are writing a Java program for the first time, you might encounter this problem about method overloading.
Do you know what method overloading is?
If not, then don't worry.
There are many times when you have multiple methods that have the same name.
This can happen if you have a class containing lots of methods and you want to make it possible for other classes to extend your interface.
In this case, it's best to use method overloading in java.
This article aims to explain step by step so that you understand it clearly and easily.
What is Overloading in Java?
In Java, two or more methods can have the same name if their parameters are different (different number of parameters, different types of parameters, or both).
This feature is called method overloading, and these methods are called overloaded methods. Here are some examples:
A method called function_name() overloads in this case. Despite having the same name, these methods accept different arguments.
Why Method Overloading in Java?
Consider the following scenario:
you have to add given numbers, but there can be any number of arguments (let's assume either 2 or 3 arguments for simplicity).
To accomplish the task, you can create two methods sum2num(int, int) and sum3num(int, int, int), for two and three parameters as appropriate. However, other programmers, such as you in the near future, may get confused as both methods behave similarly, but they differ by name.
Overloading methods is the better way to accomplish this task. And depending upon the argument passed, one of the overloaded methods gets called. It results in increasing the readability of the program.
In Java, there are different ways of overloading methods
- By changing the Number of Parameters.
- By changing Data Types of the Arguments.
- By changing the Order of the Parameters in Methods
1. By changing the Number of Parameters.
To overload a method, you must change its number of parameters when passing when you call the method.
2. By changing Data Types of the Arguments.
In many cases, you can overload methods by passing the same parameter name but with a different data type.
3. By changing the Order of the Parameters in Methods
Rearranging the parameters of two or more overloaded methods can also result in method overloading. For instance, if method 1's parameters are (String fullname, int employee_id) and method 2's parameters are (int employee_id, String fullname), both methods should be considered overloaded.
Benefits of using Method Overloading
- The use of method overloading in java improves the readability of a program.
- It gives programmers the flexibility to call the same method for different types of data.
- The code looks cleaner this way.
- Due to this, execution time gets reduced since binding occurs during compilation.
- It reduces the complexity of the code by overloading methods.
- This allows us to reuse the code again, which saves memory.
Code
As shown in the example below, there are two methods, each with a different type of data.
Output:
Conclusion
In short, method overloading is a way of allowing classes to define more than one version of an operation for a user to perform. In other words, the same method can accept different sets of parameters and return different types.
As such, it should be known that when you are writing code that uses method overloading, you should pay careful attention to your naming conventions.