Method Signature In Java
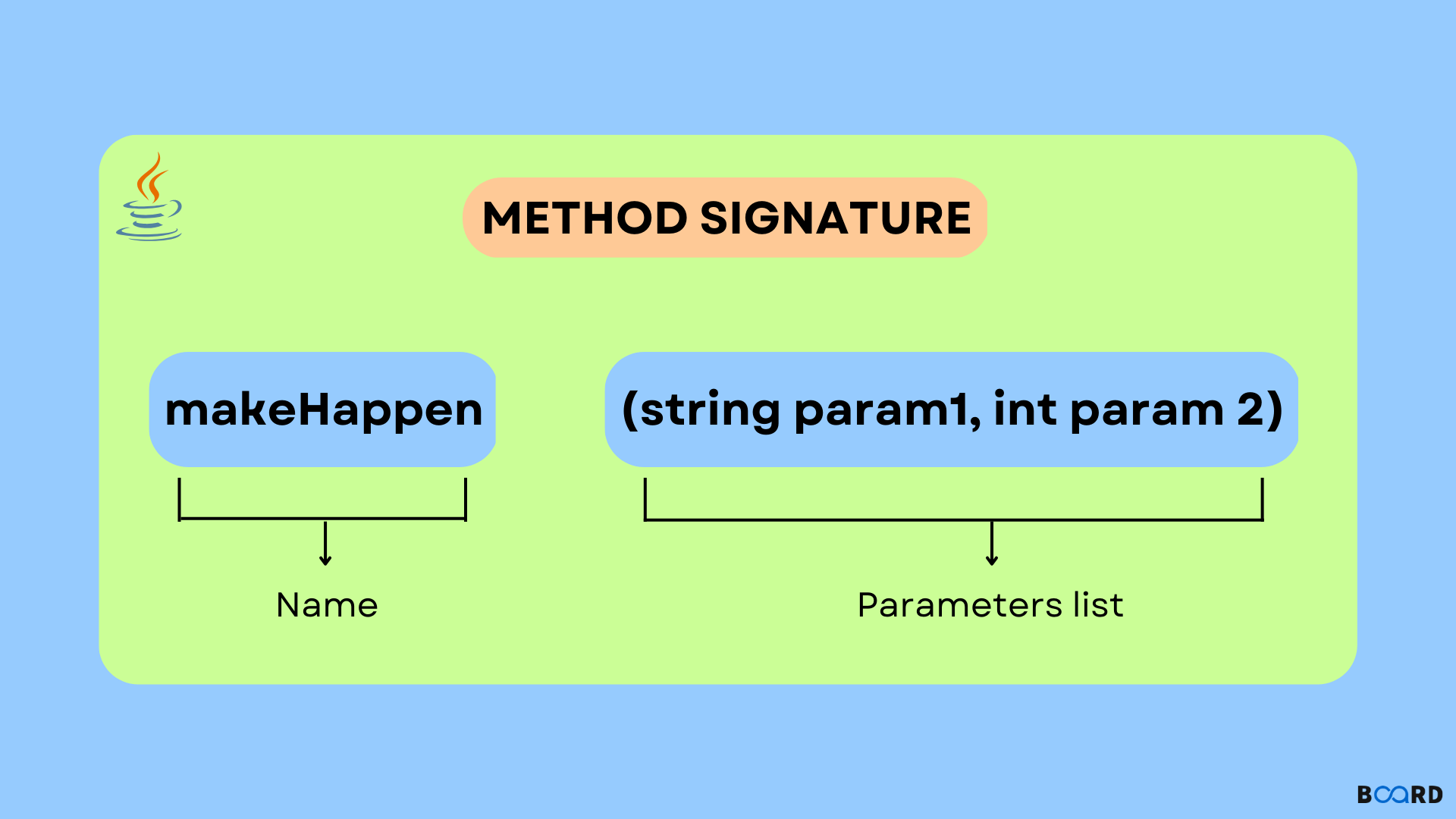
Overview
Java is a statically typed, compiled, and general-purpose programing language. The signature of a function in Java is the combination of its name and parameters. No two functions defined within a common class can have the same signature. In this article, we shall learn how to use the signature of a method in Java to define multiple methods with the same names.
Scope
This article is all about:
- Requirement Of Method Signature In Java.
- How To Modify The Signature Of A Java Method:
- Modifying the name.
- Changing the sequence of parameters.
This article however is not about:
- Any other unrelated Java concepts.
Why Do We Need Java Method Signature
Have a look at the code block below as well as its output
Output
The signature, i.e., the combination of the name and parameter sequence of both the “sum” functions of the Main class is the same. This is not allowed in Java. But by modifying the signature of our sum function(s) we can define multiple sum functions within the same (Main) class!
How To Change The Signature Of A Java Method
Change the name of function(s)
As seen in the previous example, we need multiple function definitions with the same name and sequence of parameter da-types. We can assign names to all these functions such that the naming is still relevant to the purposes that these functions serve. Example:
Output
Chaing the parameter sequence
Parameter sequence means the order of the data types of the function arguments. If the number of parameters in two function definitions differ, changing the parameter sequence is not required. The code will work just fine. Even if the number of parameters in two function definitions is the same, so long as there is a minimum of one position at which the parameters are of different types in the two functions, there should not be a problem.
But what if the parameter sequence of two functions is of the same size and the same order of data types? A compilation error is thrown, as we observed in the very first example. The following example dictates how we can change the order of parameters based on the sequence of their data types, to make the code compilation error free:
Output