Understand HashSet in Java
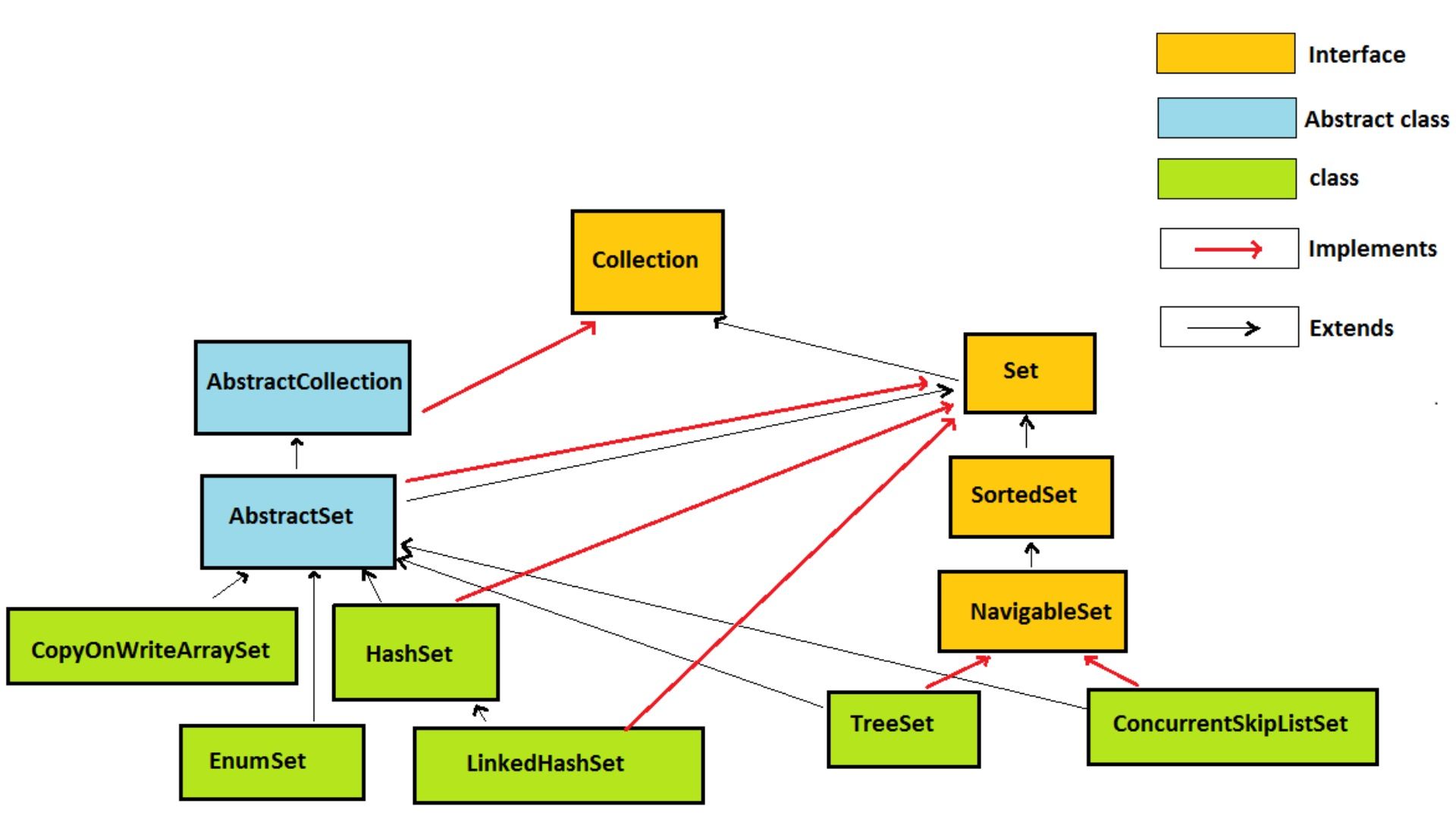
What Is Hashset In Java?
In Java, HashSet extends the Abstract class and implements the Set interface. The tool is helpful because it allows you to store unique items and access them continuously (on average).
You can not store duplicate values inside HashSet. To insert objects into the HashSet, you need to use a hash code; thus, you can store and retrieve HashSet elements in any random order. If you prefer storing and retrieving elements in sorted order, you can use TreeSet.
The most helpful advantage of HashSet is that you can store Null elements in HashSet. The HashSet class is not thread-safe; as a result, multiple threads cannot synchronize the results if they change the same HashSet instance.
Here are some important points about Java HashSet:
- Hashing is the mechanism used by HashSet to store the elements.
- A HashSet only contains unique elements.
- A HashSet can contain a null value.
- A HashSet is a non-synchronized class.
- HashSet does not maintain insertion order. Instead, elements are inserted based on their hashcodes.
- In terms of search operations, HashSets are the best option.
HashSet Declaration
The keyword HashSet is used to define a HashSet in Java. HashSet is defined as:
In a HashSet, E represents the type of elements stored.
Commonly used methods
#1. add(element):
It adds the given element in the HashSet if no such element already exists, otherwise, it doesn't.
#2. remove(element):
Using this method, you can remove an element from the HashSet.
#3. clear():
This method is used to clear all the values stored in the HashSet.
#4. size():
By using this method, you can find out how many elements are present in a HashSet.
#5. contains():
Using this method, you can determine whether a certain element is present in a HashSet. The process usually takes O(1) time.
#6. isEmpty():
Using this method, you can check whether the HashSet contains any elements. If the method finds no elements, it returns true.
#7. toArray() :
The HashSet is converted to an array using this method. Each element of the HashSet gets copied into a new array.
Code Implementation
Here is a simple implementation of a HashSet in java: