Understanding the LifeCycle of an Applet in Java
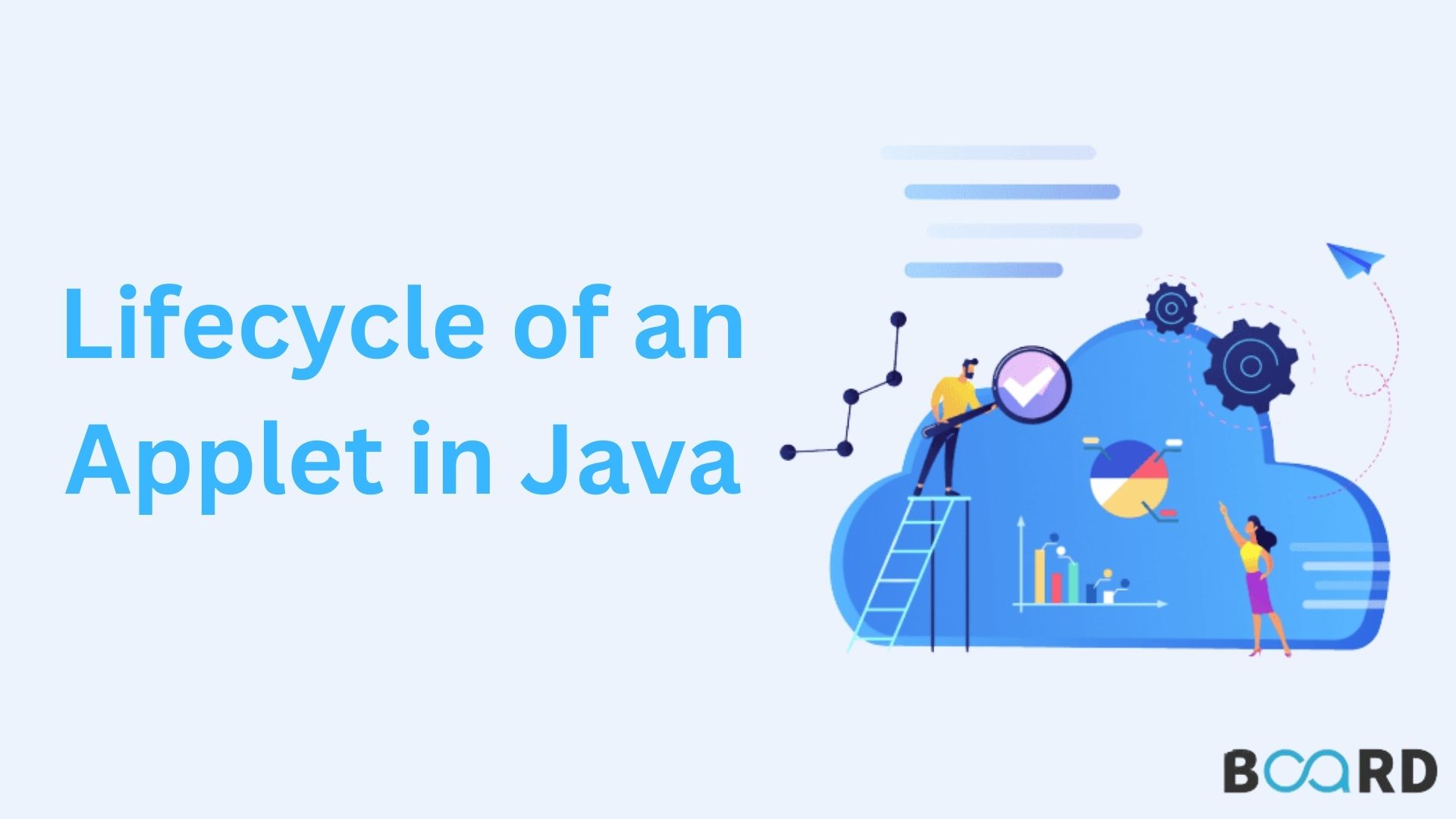
Introduction
An applet is a Java program that one can embed on a web page. It executes on the web browser and works on the client's side. Developers may use applets to make websites more dynamic and interesting.
Some of the important properties of applets are listed below:
- All applets are derived from (subclasses) from java.applet class.
- Applets are not considered a stand-alone program.
- Applets execute in a web browser or an applet viewer.
- An applet is not initiated in the main() method generally.
- System.out.println() doesn't perform the output of an applet window. Though, We can handle it with various AWT methods.
Lifecycle of an applet
When the functioning of an applet begins the following methods are called in a sequence:
- init()
- start()
- paint()
When the functioning of an applet is about to stop, then the following methods are called in a sequence:
- stop()
- destroy()
Now we will go through each of these methods one by one:
- init(): This is the very first method that is called. It is the place where we should initialize variables. This method is called only one time.
- start(): This method is called after init() method. This is called to restart the applet after it terminates.
- paint(): This method is called each time an AWT-based applet’s output is reconstructed. This situation can take place frequently for many reasons.
- stop(): This method is invoked whenever there is a situation that a web browser leaves out the HTML document that contains the applet.
- destroy( ) : The method is invoked whenever the environment signifies that the applet requires to be removed completely from the memory. At this point, we should release any resources that is begin used by the applet.
Source Code
If we try the following program then the compiler will produce an error this is because it’s an application, not a program. Thus, the way we can run both is quite different from each other.
Let us discuss two methods using which we can run them:
- Running an applet by using a Java-compatible web browser.
- Using an applet viewer. An applet viewer is responsible for executing an applet in a window.
These methods are discussed below in detail:
- Java-enabled web browsers:
For executing an applet in a web browser, we are required to write an HTML text file that consists of a tag that can be loaded into an applet. APPLET or OBJECT tag can be used for this purpose. Using APPLET, here is the HTML file that executes myFile :
The width and height statements are used to determine the display area of used by the applet.
It is important to note that browsers like chrome and firefox no longer supports NPAPI (technology required for Java applets).
- Using appletviewer:
It is the easiest way to run an applet. To execute the myFile with an applet viewer, you may also execute the HTML file shown earlier. For example, if the preceding HTML file is saved with
myFileht.html, then the following command line will run myFile:
Conclusion
In this article, we discussed applets in Java: what are they and how we can run them in our web browser. I believe that this article will definitely help you to strengthen your knowledge in the field of Java.