Understanding Singleton Class in Java
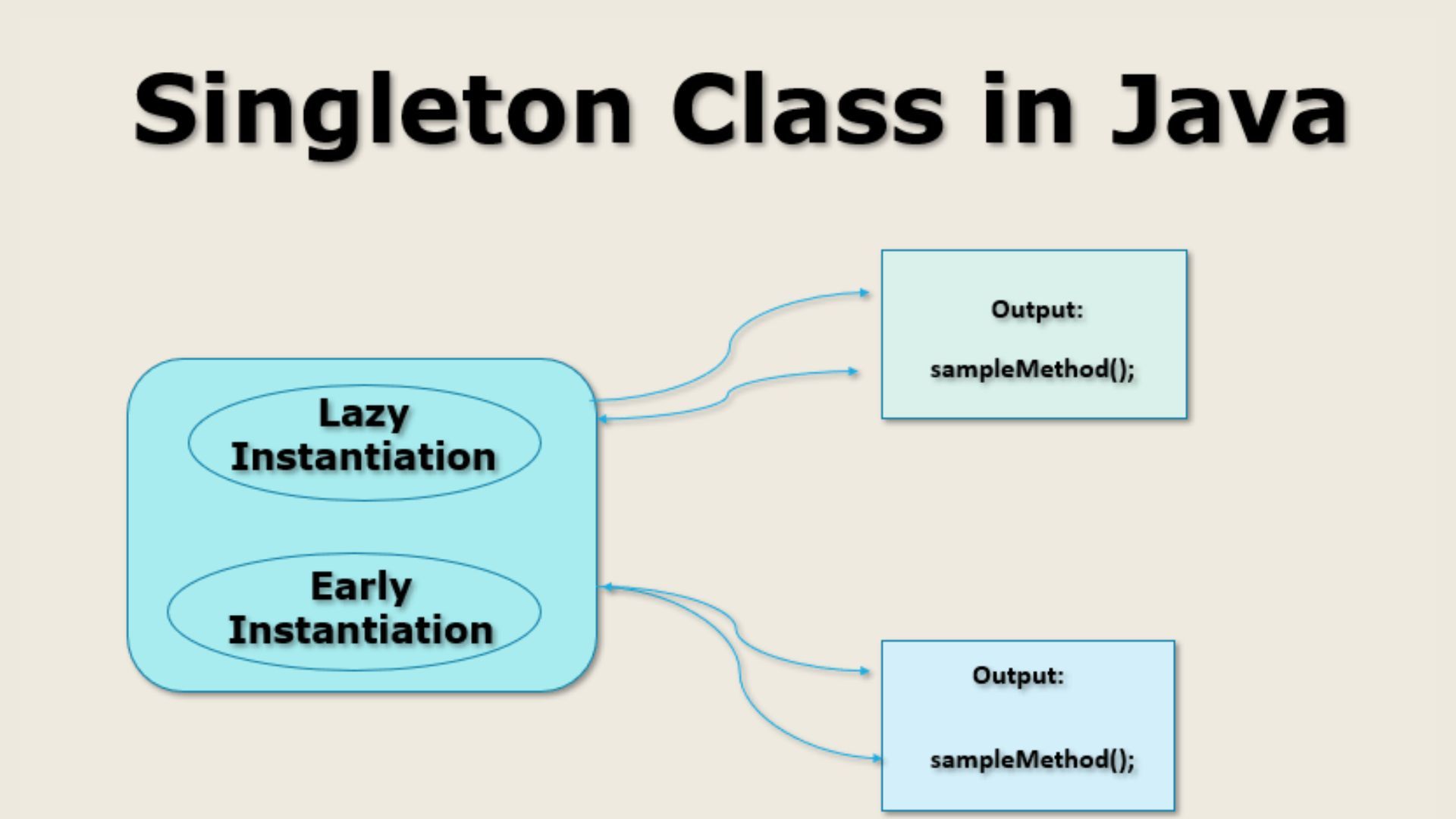
Introduction
The purpose of design patterns is to solve a specific problem.
A popular design pattern is the Singleton design pattern. This method solves various problems by creating only one object of one class and using it wherever needed.
What is a Singleton class in Java?
The Singleton Pattern recommends defining a class with only one instance and providing global access to it.
To put it another way, a class should only create a single instance, and only one object should get used by all other classes. In the Singleton class, the goal is to limit the number of objects, controlling their creation.
The singleton design pattern comes in two forms:
- Early Instantiation: Creating instances at runtime.
- Lazy Instantiation: Creating new instances as needed.
Singletons get instantiated once, and their variable points to their instance. Consequently, anything we modify inside a class is visible if it is accessed through any variable of a class type defined and affects all variables within the class type.
Java Singleton Class Syntax:
Benefits of using Singleton Design pattern
- The Singleton object prevents other objects from instantiating their own copies, allowing them to access the same instance.
- Due to the class's control over the instantiation process, it is flexible in how it can change the instantiation.
- As we know, when we create objects of any class, they occupy memory space, so creating many of them will consume more memory. Because singletons have only one object, they save memory.
How to create a Singleton class in Java?
Creating a singleton class requires creating a static member, a private constructor, and a static factory method.
- Static member: Because it is static, it only gets memory once, containing the singleton instance.
- Private constructor: This prevents outsiders from instantiating the Singleton class.
- Factory static method: The static factory method returns the instance of the Singleton object to its caller.
The following properties must get implemented to create a singleton class:
- Create a private constructor of the class so that objects can only get created within the class.
- Create a private attribute of the class type referencing the single object.
- Create a public static method for creating and accessing the object. We will create a condition inside the method to prevent multiple object creation.
The following example shows how a singleton class works in Java.
Conclusion
The Singleton Pattern is a design pattern that restricts client access to a single of class. It is used to provide a global point of access to an object and avoid the use of multiple objects in this case.
It ensures that there is only one instance of an object in the system which makes it easier for the programmer to deal with it.