Method Overloading And Overriding In Java
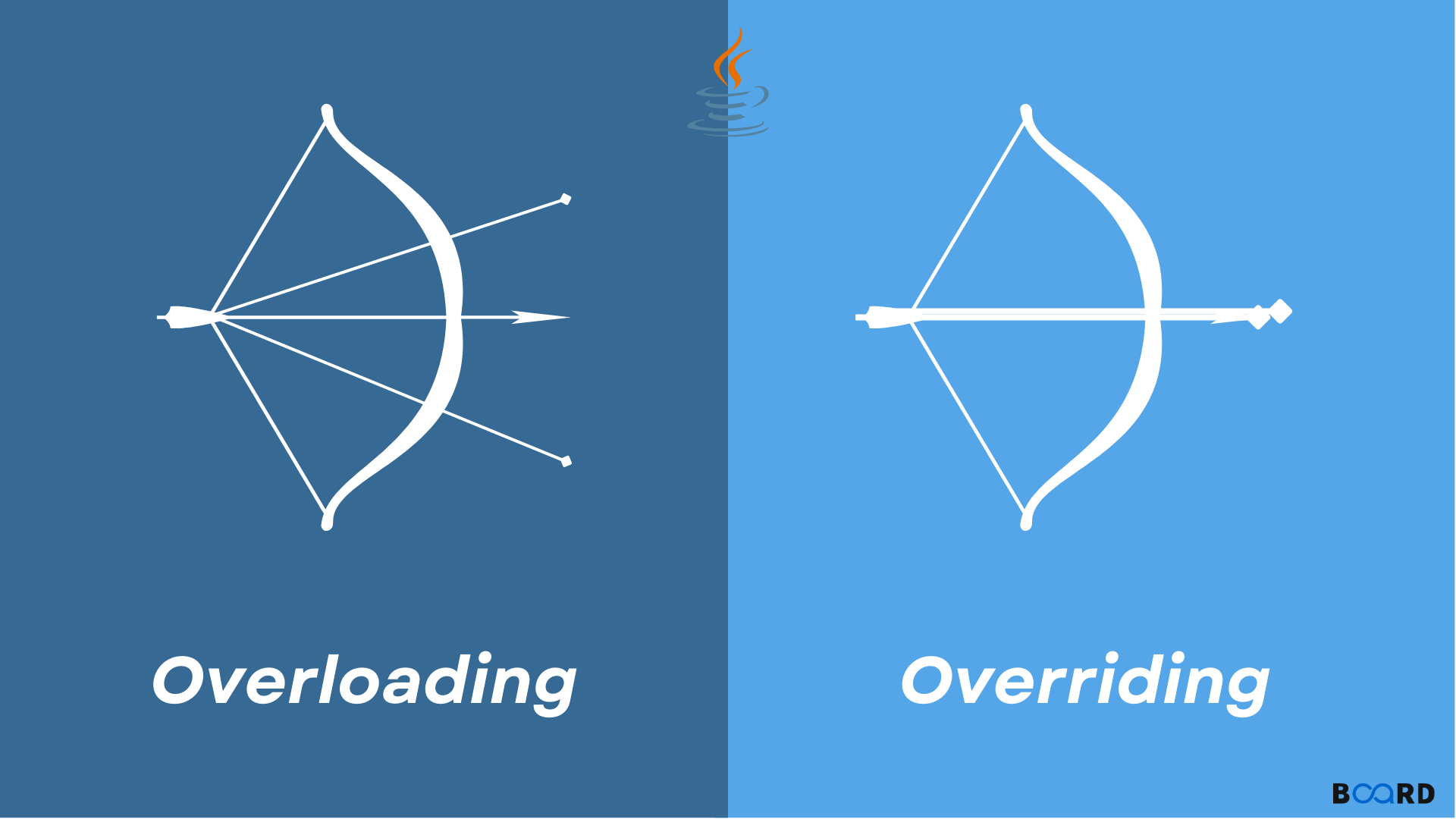
Introduction
Method overloading and overriding are two concepts that make Java programming enjoyable. They help developers save time, code, and effort when they have to write code repeatedly.
This post is about method overloading and overriding in java. These two programming concepts getting mainly used for extending existing methods or classes. This article teaches us more about its basic concepts and the difference between method overloading and method overriding.
What is Method Overloading in Java?
The method overloading technique allows a method to share the same name but differ based on its arguments. It is a compile-time polymorphism.
We can't overload return types. Overloading static methods is allowed, but the input parameters must be different. We cannot overload two methods if they only differ by a static keyword. It is similarly possible to overload the main() method, just as you can with other static methods.
To understand how Java method overloading works, let's take a look at a simple program.
What is Method Overriding in Java?
Overriding methods is a runtime polymorphism. In method overriding, a derived class provides specific implementations of methods present in the base class.
If both classes contain methods with the same name, arguments, or parameters, then one will always override the other. It depends on the object which method will get executed.
In any object-oriented programming language, overriding requires a subclass or child class to provide variants of methods previously provided by a superclass or parent class.
Difference Between Method Overloading And Method Overriding In Java
The difference between overloading and overriding a method in Java is quite complex. Following is a list of differences between overloading and overriding methods:
Conclusion
In this article, we discussed the difference between method overloading and method overriding. Java allows method overloading and method overriding in classes, which is called polymorphism. Polymorphism is the ability to solve a problem with more than one form or method.
Overriding methods allow you to change the behavior of a specific method in the hierarchy tree. This blog explains the meaning and difference between overloading and overriding so that it becomes clear when to use it