Java Programming Techniques and APIs
A Guide to Association, Aggregation, and Composition in Java
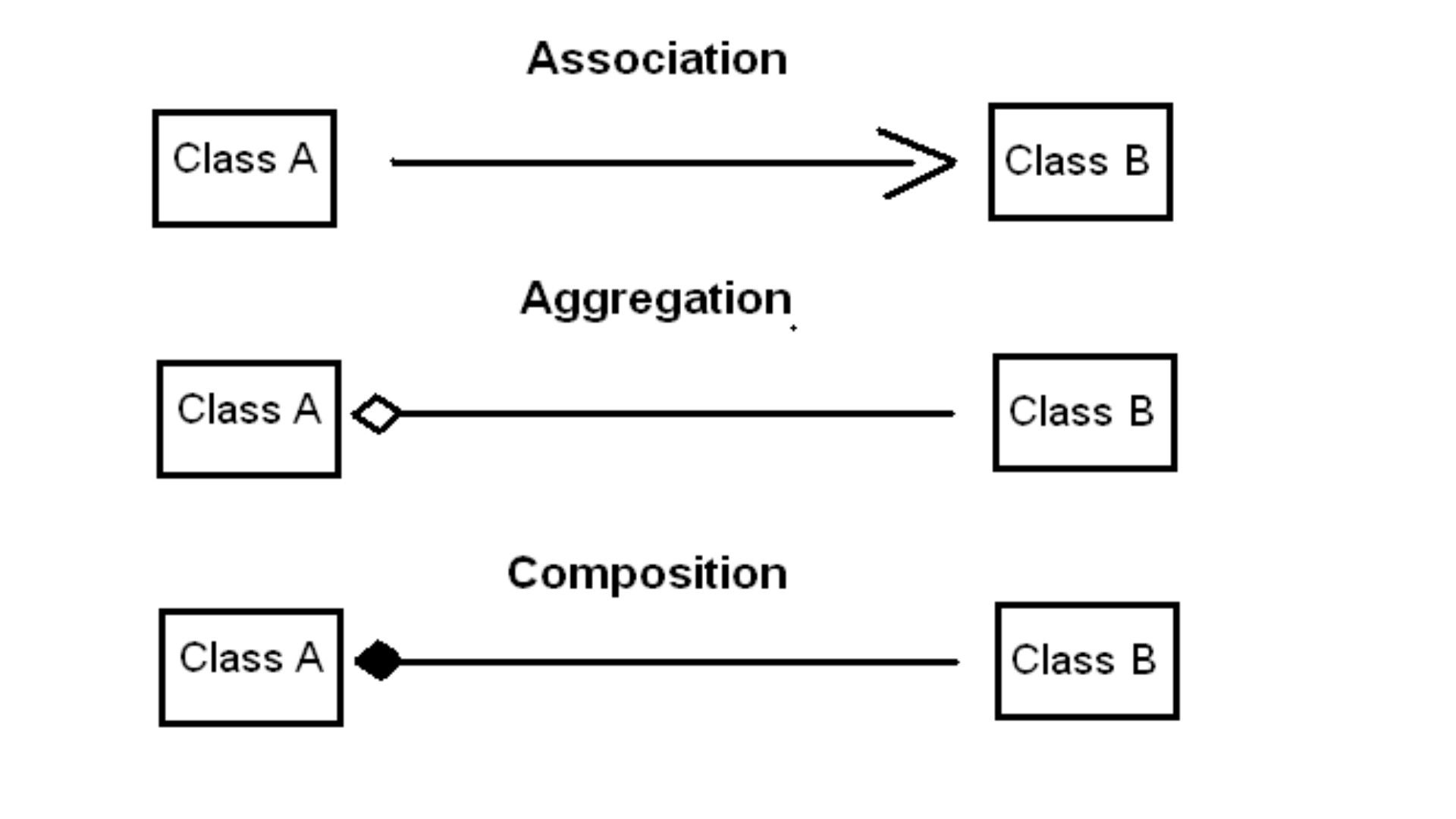
What Is Association In Java?
Any relationship between two entities is known as an association. In Java, associations are relationships that can get established between classes. In general, these relationships fall into four categories:
- One-to-One relation
- One-to-many relation
- Many-to-one relation
- Many-to-many relation
In Java, there are two forms of Association:
a) Aggregation
b) Composition
What is Aggregation in Java?
Aggregation relationships are "has-a" relationships. There is a difference between composition and aggregation because aggregation does not involve owning objects. There is no dependency between the objects, so they can exist even if their parent object gets deleted.
Here is an example to help you understand this:
In a car, wheels are attached to the body, so when those wheels get removed, the body will still have wheels.
This relationship initially developed for assembling parts to a larger construct capable of more than its parts since a car without wheels won't be as helpful as a car with wheels.
There should be no problem if we install these on another car or use other (preexisting) wheels. Obviously, a car that does not have wheels or one that has one detached won't be as helpful as one that does.
In fact, that's exactly the reason this relationship exists: to assemble the parts into a bigger construct that can do more than its parts can alone. In Aggregation, members don't have to get tied to one container since owning is not required.
What is Composition in Java?
In Java, the composition is a more tightly coupled form of relationship. Java composition is also called strong association. This association is also known as a Belongs-To association since one class is essentially a member of another and thus exists due to this relationship.
As part of a Composition association, classes cannot exist independently. When the larger class containing the objects of the smaller class gets removed, the smaller class also ceases to exist logically.
Rooms, for example, belong to buildings, so a building has rooms.
Therefore the difference between "belongs-to" and "has-a" can only be determined by one's point of view. Due to its ownership by the containing object, a composition is a strong form of "has a" relationship. Therefore, the objects' lifecycles are tied.
Therefore, if we destroy the owner's object, all its members will also get destroyed. The room in our previous example will get destroyed along with the building. Note that doesn't mean, however, that any of the components cannot exist without the containing object.
For example, tearing down all the walls in a building will destroy the rooms but leave the building intact.
Depending on the cardinality, a containing object may contain as many parts as we want. However, each part must have its own container.
Code Example
As shown in the following example, Employee has an object called Address, which contains information such as emp_city, emp_state, emp_country, etc. When such a relationship exists, it is Employee HAS-A address.