Java Programming Techniques and APIs
Finally Block In Java
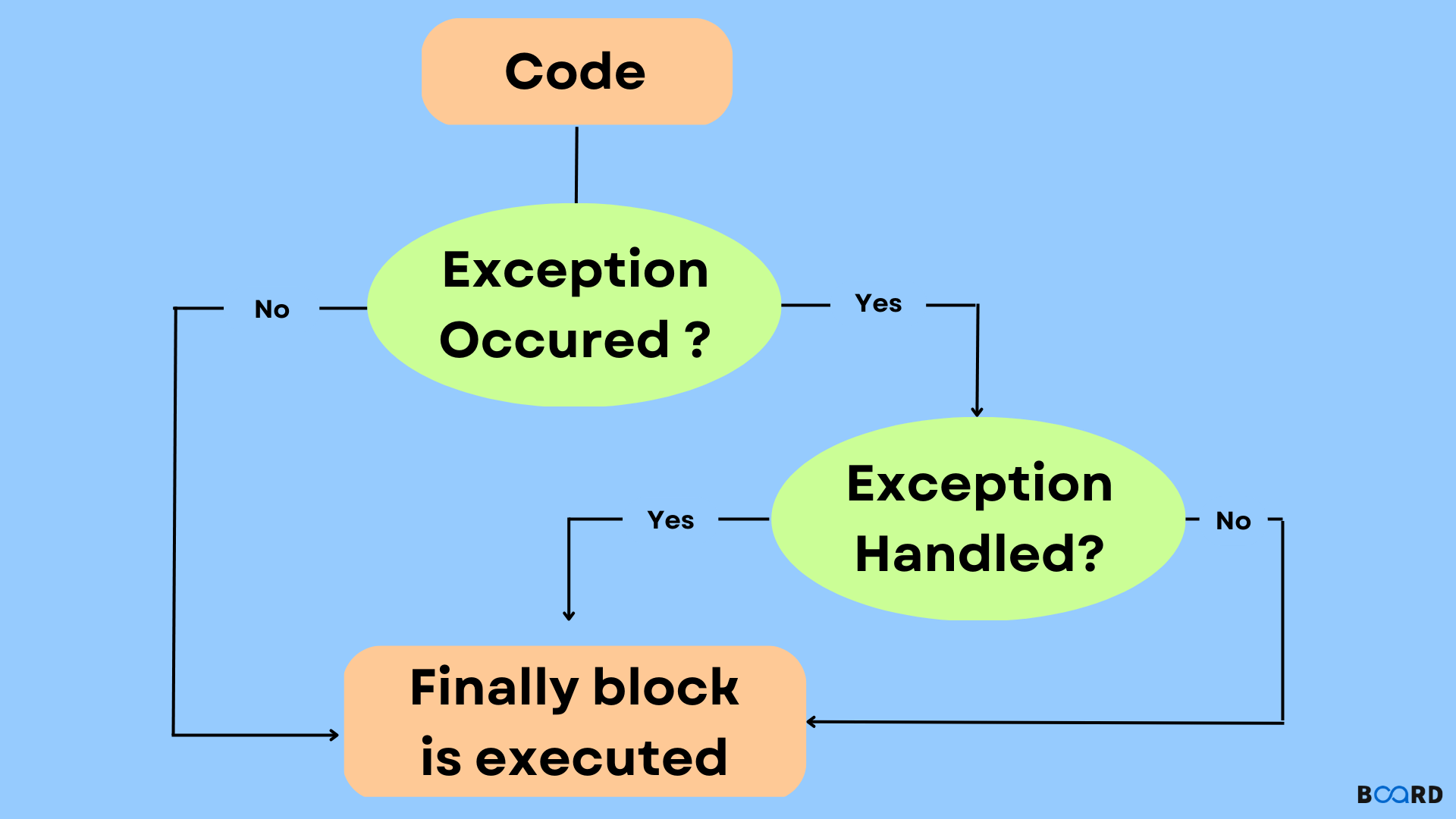
Overview
In Java, we are provided with the “finally” block, which we may use to place important code snippets, for example, clean-up code, code for closing a file, code for closing a network connection, etc.
The code in the finally block runs regardless of whether or not an exception arises whether or not a thrown exception is handled, etc. Following are the three common practical use cases of the final block in Java:
When no exception arises
The program, in this case, executes properly and does not raise any exceptions. After the try block has completed running, the finally block starts running. Let us have a look at the code snippet below to gain a better understanding:
Output
An exception is thrown and handled
The program, in this case, raises an exception which is later handled by a catch block. Lastly, once the catch block has completed running, the finally block executes.
Output
When an exception is thrown and not handled by any catch block
The program, in this case, throws an exception that is not handled by any catch block. Hence, after the try block has finished executing, the finally block starts running. Once the finally block completes executing, our program ends abnormally. Although, the finally block will have run just fine.
Output