Java Programming Techniques and APIs
What are Functional Interfaces in Java?
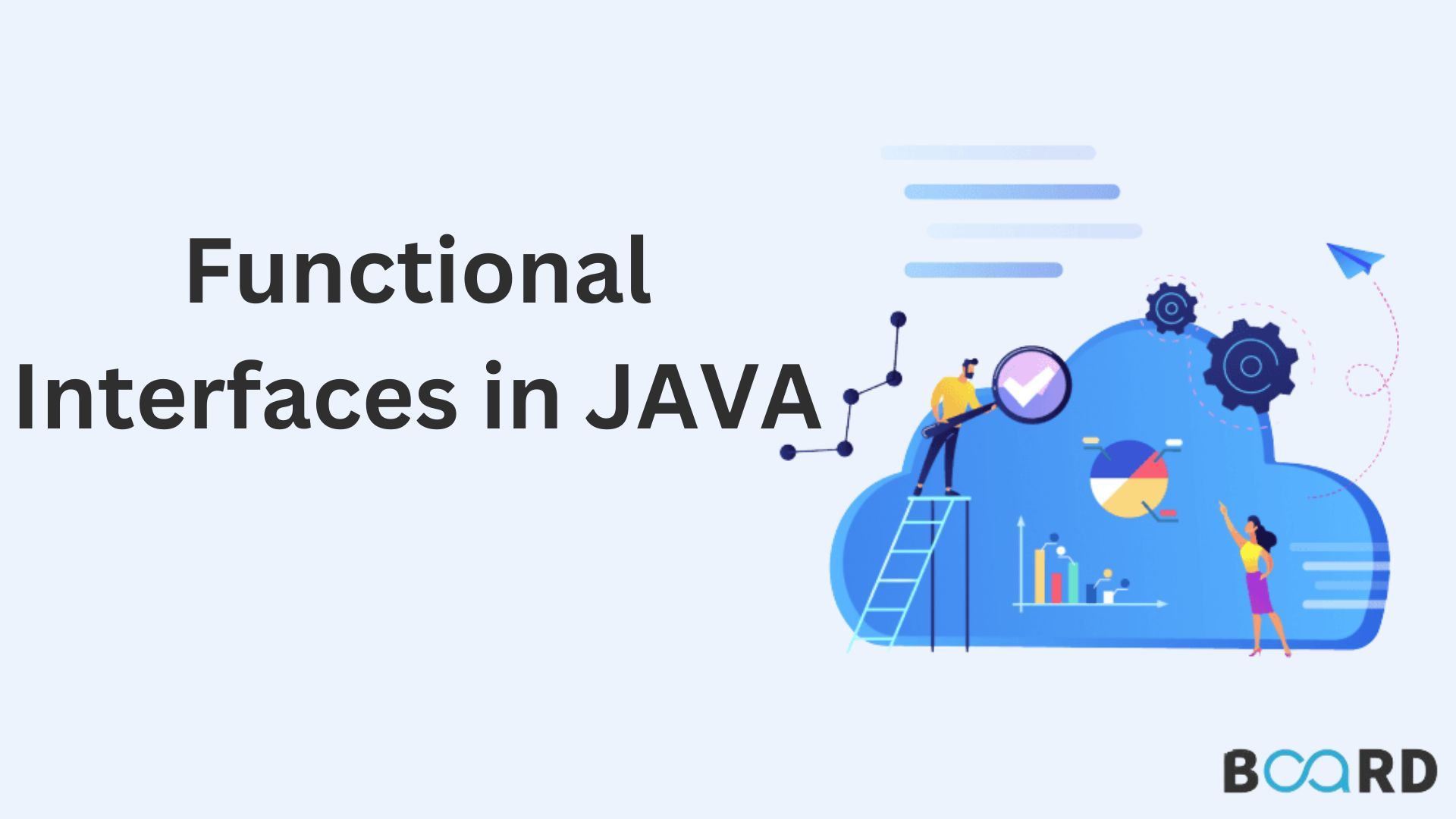
Introduction
Objects and classes are at the heart of Java since Java is an object-oriented programming language. Java does not have any independent functions. They are either part of a class or an interface. And to use them, we must either call the class or the object of that class.
Java's functional interface makes it possible to implement functional programming. Functions are independent entities in functional programming. Using the function is just like using a variable, such as passing a function as a parameter or returning a function.
Java 8 introduced functional interfaces. There can only be one abstract method in functional interfaces and any number of static or default methods (non-abstract). During its declaration, an abstract method does not require implementation and must get overridden by the class that implements it.
In interface classes, default methods can get directly used, overridden, or redefined. The method name must get preceded by the interface name for static methods. These cannot get overridden by interface implementations.
Java also refers to functional interfaces as Single Abstract Method (SAM) interfaces. Java 8 uses lambda expressions to represent functional interface instances. The lambda expression is an anonymous (has no name) shortcode block that accepts parameters and returns a value.
Following syntax will demonstrate how we can use functional interface in Java
@FunctionalInterface Annotation
@FunctionalInterface ensures that there can be no more than one abstract method in a functional interface. The compiler flags an 'Unexpected @FunctionalInterface annotation' if there is more than one abstract method. This annotation is optional, however.
Built-in Java Functional Interfaces
For common cases, Java provides predefined functional interfaces. The functional interface gets converted into many interfaces. By using the @FunctionalInterface annotation. The following are a few of these interfaces:
#1. Runnable -
It only contains the run() method. This allows you to write applications that can run on separate threads.
#2. Comparable -
There is only one method in this interface, compareTo(). Classes implementing the Comparable interface can compare and sort their objects.
#3. ActionListener -
There is only one method in it, actionPerformed(). This component handles all the action events like mouse clicks on components.
#4. Callable -
In this class, there is only one method called call(). Using this method, you can monitor the progress of a thread's execution of a function.
Types of Functional interfaces
There are four main types of functional interfaces in Java:
#1. Consumer
As of Java 8, the Consumer interface accepts one gentrified argument and does not return any value.
#2. Predicate
Predicate functions in Java return booleans based on one argument. In most cases, it gets used to filter values from a collection. Additionally, it is a function that returns a boolean in response to a gentrified argument.
#3. Supplier
Suppliers with functional interfaces in Java don't take arguments, unlike functional interfaces. A value gets returned when we call the supplier. Supplier is a generic interface, so the type of value must get specified using <> (Angular brackets) for the get() function to return it.
#4. Function
Function type functional interfaces receive a single argument, process it, and return a result. In this type of functional interface, a user inputs a key, and the map searches for the value corresponding to the key.
Observations
Functional interfaces in Java can be summarized as follows:
- The abstract method of functional interfaces is the only one supported. In an annotation of a functional interface, more than one abstract method can be declared, i.e., @FunctionalInterface, if the annotation does not implement or include a function interface. When there is more than one functional interface, it is not get called a functional interface. Interfaces without functionalities get referred to as non-functional interfaces.
- There is no such need for the @FunctionalInterface annotation as it is voluntary only. The purpose of this is to check the compiler level. Besides this, it is optional.
- Static or default methods can get added indefinitely to the functional interface. Simply, there is no limit to a functional interface containing static and default methods.
- Java does not prohibit overriding methods from the parent class.
- Several functional interfaces are included in Java 8's java.util.function package