Java Programming Techniques and APIs
Java Stream
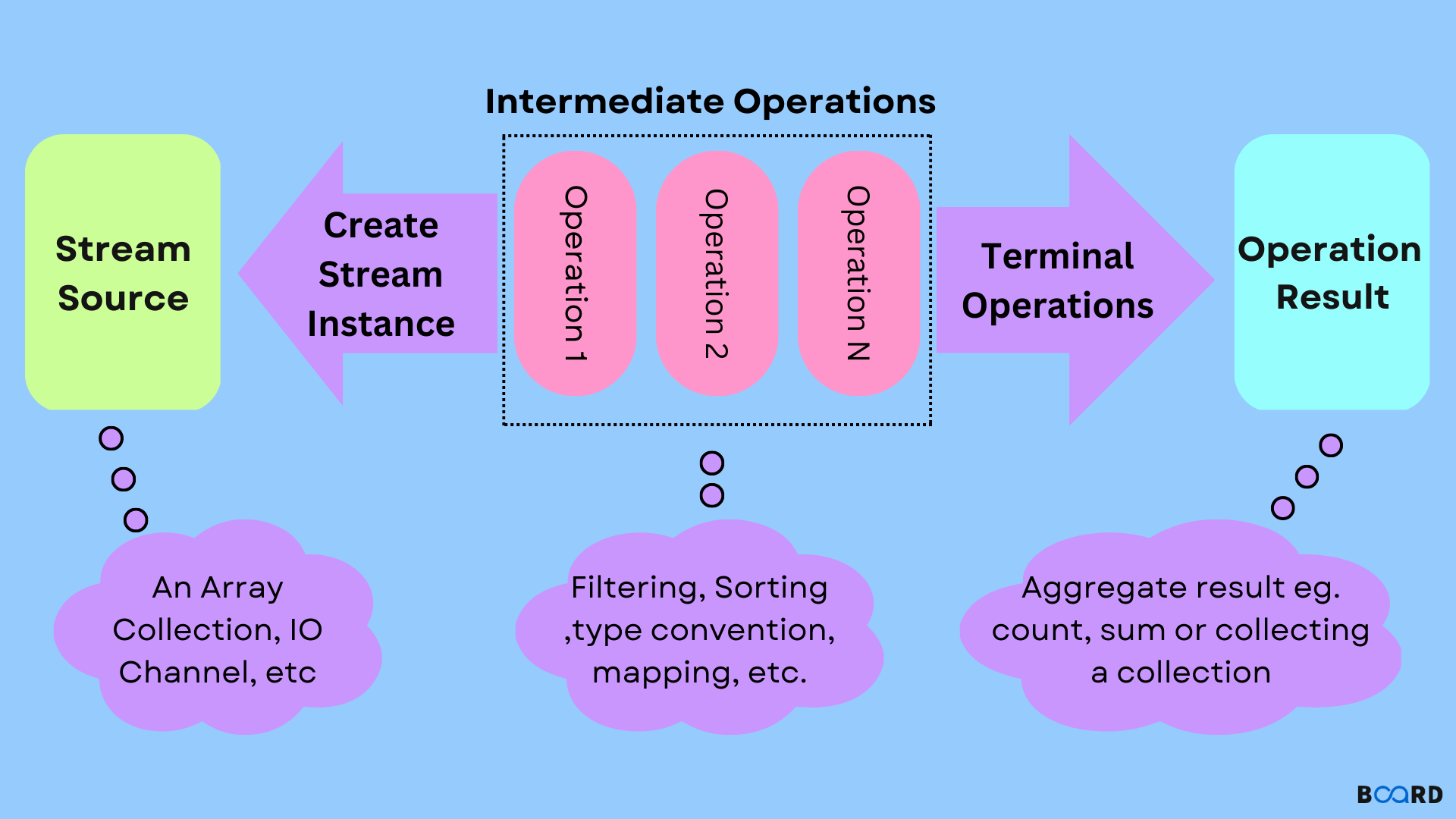
Introduction
Stream is one of the most important and commonly used concepts in java. Streams are powerful and flexible, providing a mechanism for reading and writing data, and allowing you to build your own solution to handle a specific problem.
This article will help you understand what stream actually is, as well as its implementation in Java.
What Is Stream In Java And Its Types?
The java.util.stream is a new package in Java 8 that provides additional functionality. This package supports functional-style operations on elements through classes, interfaces, and enums. By importing the java.util.stream package, you can use stream.
A Java stream typically supports multiple devices, including keyboards, networking sockets, and disk files. Thus, it is convenient to handle various types of I/O operations similarly.
Streams in java fall into two basic types: byte streams and character streams. Byte stream classes allow inputs and outputs of bytes, while character stream classes allow inputs and outputs of characters.
Features of Streams in Java
The features of the stream are as follows:
- There are no elements stored in the stream. Using a pipeline of computational operations, it conveys elements from a source (a data structure, an array, or an I/O channel).
- The function is the essence of the stream. Stream operations do not alter their source. For instance, if you filter a Stream obtained from a collection, rather than removing elements from the collection from which the stream gets obtained, you end up with a new Stream without the filtered elements.
- As a lazy evaluation system, streams evaluate code only when needed.
- A stream only visits its elements once. For each source element to get revisited, a new stream must get generated.
Different Operations On Streams
Following are some of the operations that you can perform on Streams to get better values.
Intermediate Operations
map:
This method returns a stream of elements after being applied to the specified function.
filter:
By using the filter method, you can select elements according to a predicate.
sorted:
To sort the stream, we use the sorted method.
Terminal Operations
collect:
Collection methods return the results of intermediate operations performed on a stream.
forEach:
ForEach iterates through the stream element by element.
reduce:
When a stream contains multiple elements, the reduce method reduces them to one value. A BinaryOperator is passed as a parameter to reduce the method.
Initially, ans has 0 as its value, and I get added to it.
Java Stream Example
Filtering Collections with Streams. In this example, we are filtering data using a stream.
Output:
Conclusion
Stream operations are one of the most powerful tools in the Java collection framework. They provide many benefits with high performance, multiple functions support, and easier-to-understand abstraction over data sets.
The benefit of the stream is that it allows data processing to be performed lazily. This is the main reason why the Java Stream API is used so often within collection pipelines, whenever you need pre-calculating some processing on each element