Java Programming Techniques and APIs
A Quick Guide to Linked Hashmap in Java
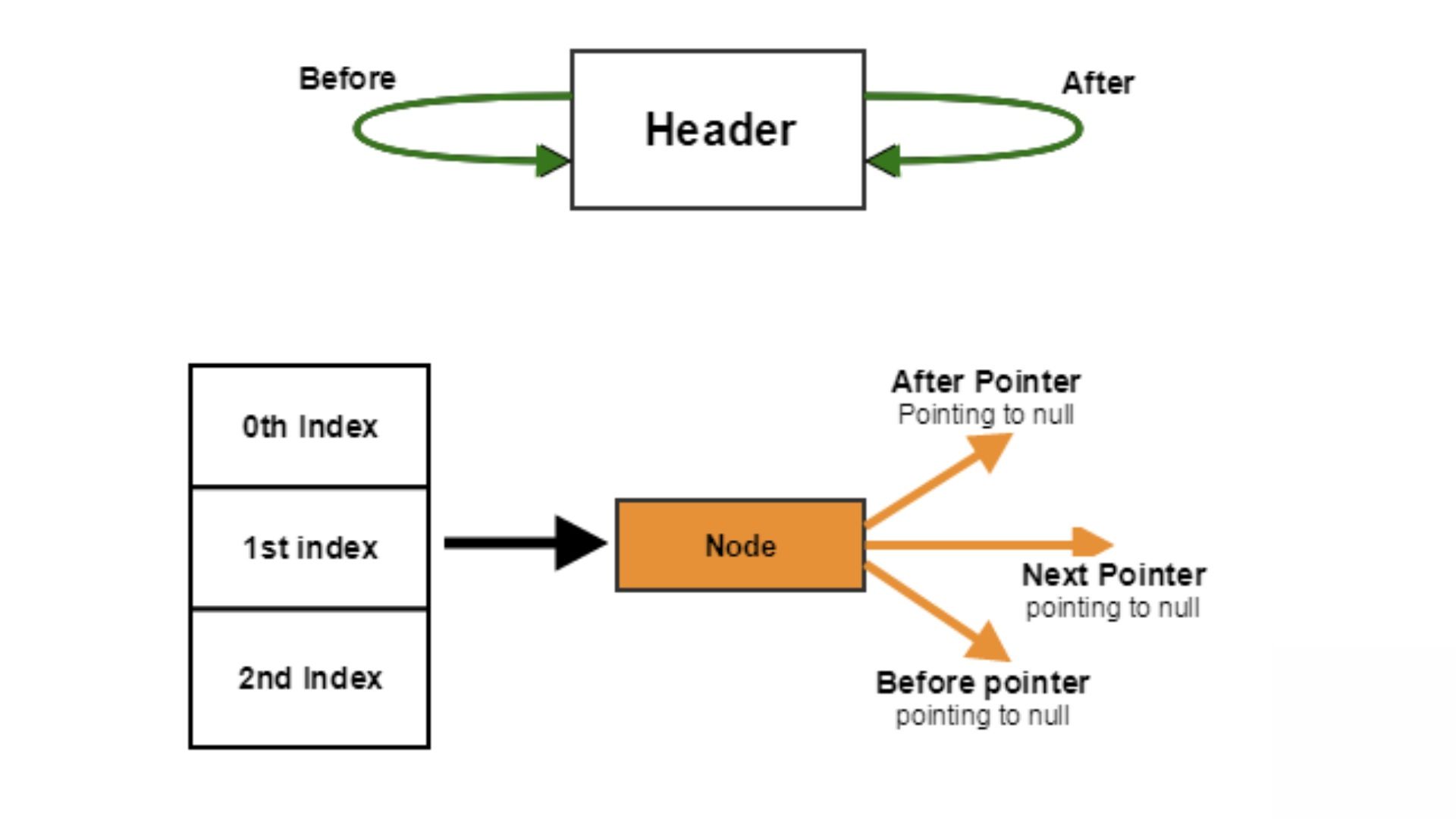
Java Class: Linked Hashmap
The Java LinkedHashMap class implements the Map interface by combining Hashtables and Linked Lists. It has predictable iteration order. This class inherits the HashMap class and implements the Map interface.
Few things to remember :
- LinkedHashMaps contain values based on their keys.
- A LinkedHashMap in Java contains unique elements.
- The Java LinkedHashMap can store a null key and a null value.
- The Java LinkedHashMap does not support synchronization.
- LinkedHashMaps in Java maintains insertion order.
- Java's HashMap class has a default capacity of 16, with a 0.75 load factor.
Syntax
It is necessary to import the Java.util.LinkedHashMap package before creating a linked hashmap.
Here,
Key - A unique identifier for each element of a map (value)
Value - a map's elements that correspond to its keys
Java LinkedHashMap class Constructors
LinkedHashMap Methods
The following are some of the methods provided by LinkedHashMap class for performing various tasks on the map.
Element Insertion into LinkedHashMap.
- put() -
This function inserts the key/value mapping into the map.
- putAll() -
This map contains all the entries from the specified map.
- putIfAbsent() -
In the absence of the specified key, inserts the specified key/value mapping into the map.
Accessing Elements from LinkedHashMap
1. Using entrySet(), keySet() and values()
- entrySet() -
This function returns a set of the map's key/value mappings.
- keySet() -
Returns a list of all the map's keys
- values() -
Provides a list of all map values
2. Using get() and getOrDefault()
- get() -
Specifies the key and returns the value corresponding to that key. If no key exists, it returns null.
- getOrDefault() -
The key value returns if the key exists. It returns the default value if the key does not exist.
Remove Elements from LinkedHashMap
- remove(key) -
This function deletes the entry associated with the specified key from the map.
- remove(key, value) -
Using this function, you remove a key from a map based on whether it maps to a value and return a boolean.
Code Sample
The following code will demonstrate how to use linked hashmap in java along with performing various operations.