Java Programming Techniques and APIs
Packages in Java
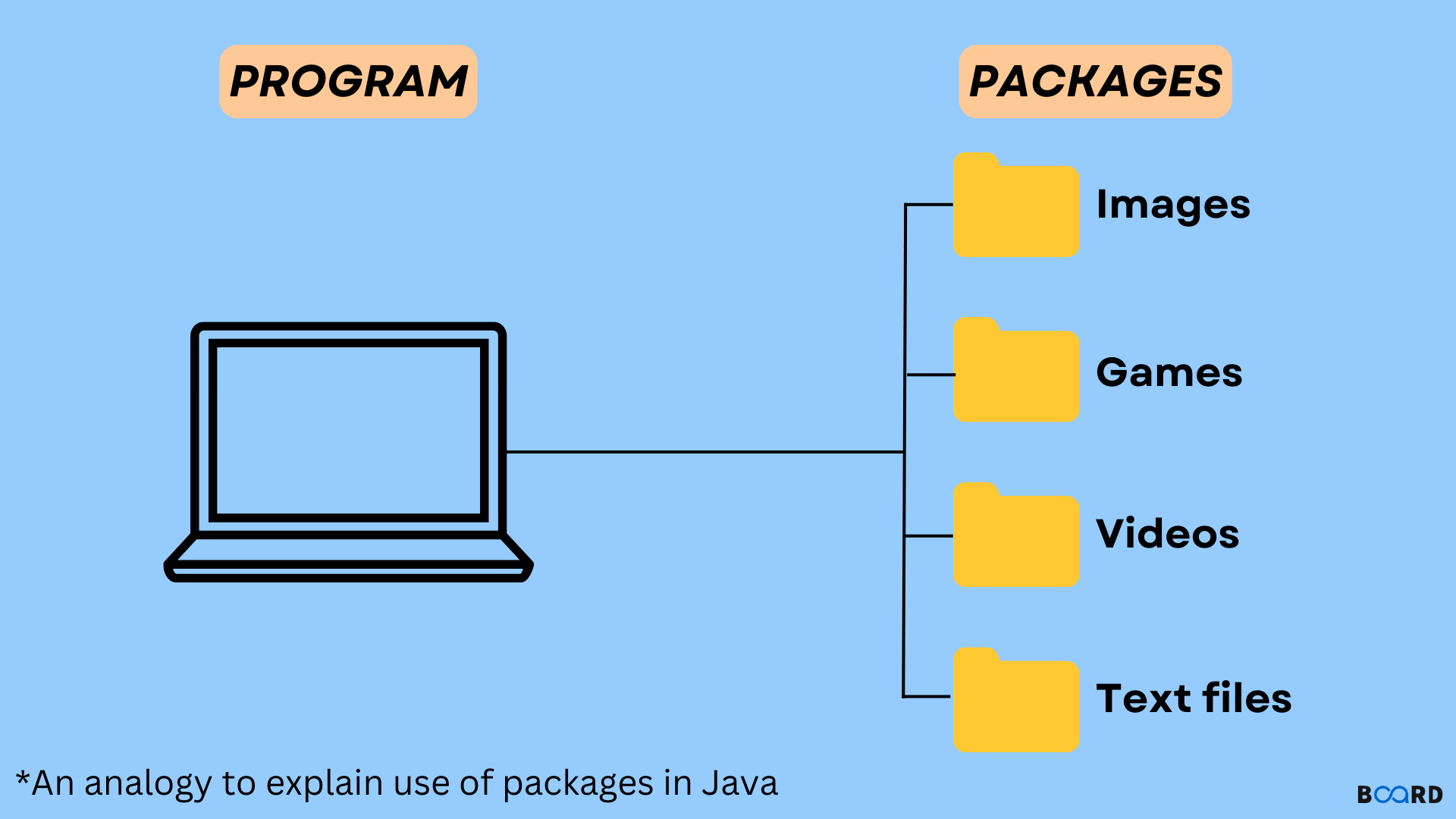
Introduction
In Java, a package is a way to bundle together related classes, packages, and interfaces. Uses for packages include:
Avoiding name clashes Making classes, interfaces, enumerations, and annotation usage easy, for instance, there may be two classes with the name Employee in two packages, college.staff.cse.Employee and college.staff.ee.Employee.
Protected and default have access control at the package level, providing regulated access. Classes in the same package and its subclasses can access a protected member. Classes belonging to the same package are the only ones with access to a default member (without any access specifier).
Packages are an example of data encapsulation (or data-hiding).The only thing left to do is group similar classes into packages. After that, we can use it in our software by writing a straightforward import class from pre-existing packages. A package is a container for a collection of related classes, some of which are maintained for internal use while others are made accessible and exposed.
As many times as we need to in our software, we can reuse already-existing classes from the packages.
How packages work?
Directory structure and package names are strongly connected. The three directories college, staff, and cse are present in both staff and college, for instance, if the package name is college.staff.cse. Additionally, the variable CLASSPATH makes the directory college accessible; specifically, the path to the parent directory of the college is listed in CLASSPATH. Making sure that classes are simple to find is the goal.
Package naming guidelines: Package names are arranged in the domain names' reverse order, for example, org.geeksforgeeks.practice. The preferred convention, for instance, in a college is college.tech.cse, college.tech.ee, college.art.history, etc.
Adding a class to a package: Using the package name at the top of the programme and putting it in the package directory, we may add new classes to a package that has already been established. To declare a public class, we must create a new java file; otherwise, we may just add the new class to an existing java file and recompile it.
Subpackages: Subpackages are packages that are contained within a larger package. These must be specifically imported because they are not imported by default. Additionally, members of a subpackage are treated as a different package for protected and default access specifiers because they have no access privileges.
Example:
Types of packages
Built-In Packages
Numerous classes from the Java API are included in these packages.
Several of the frequently used built-in packages include:
1) Java.lang: Provides classes for languages in use (e.g classed which defines primitive data types, math operations). This package is imported automatically.
2) Java.io: This package contains classes that handle input and output operations.
3) java.util: Contains utility classes which provide data structures like Linked List, Dictionary and support ; for Date / Time operations.
4) java.applet: This package includes classes for building Applets.
5) Classes for implementing the elements of graphical user interfaces are implemented in the Java.awt library (like button , ;menus etc).
6) Java.net: This library includes classes that support networking operations.
User-defined packages
These are the packages the user has defined. MyPackage is first created as a directory (name should be same as the name of the package). Then construct the MyClass inside the directory with the first sentence being the package names.
Now we can use the Newclass in our program.
Application
- A collection of classes, interfaces, etc. can be bundled together by programmers using their own packages.
- It is a good idea to arrange relevant classes that you build so that a programmer can quickly ascertain the relationship between the classes, interfaces, enumerations, and annotations.
- Since the package generates a new namespace there won't be any name conflicts with names in other packages.
- Using packages, it is easier to provide access control
- Additionally, it is simpler to find the linked classes.
Summary
A Java package is a collection of sub-packages, interfaces, and classes of a common type.In Java, there are two types of packages: built-in packages and user-defined packages.Many pre-built packages are available, including java, lang, awt, javax, swing, net, io, util, sql, etc.