Java Programming Techniques and APIs
Thread Priority in Java: What is it & How to set?
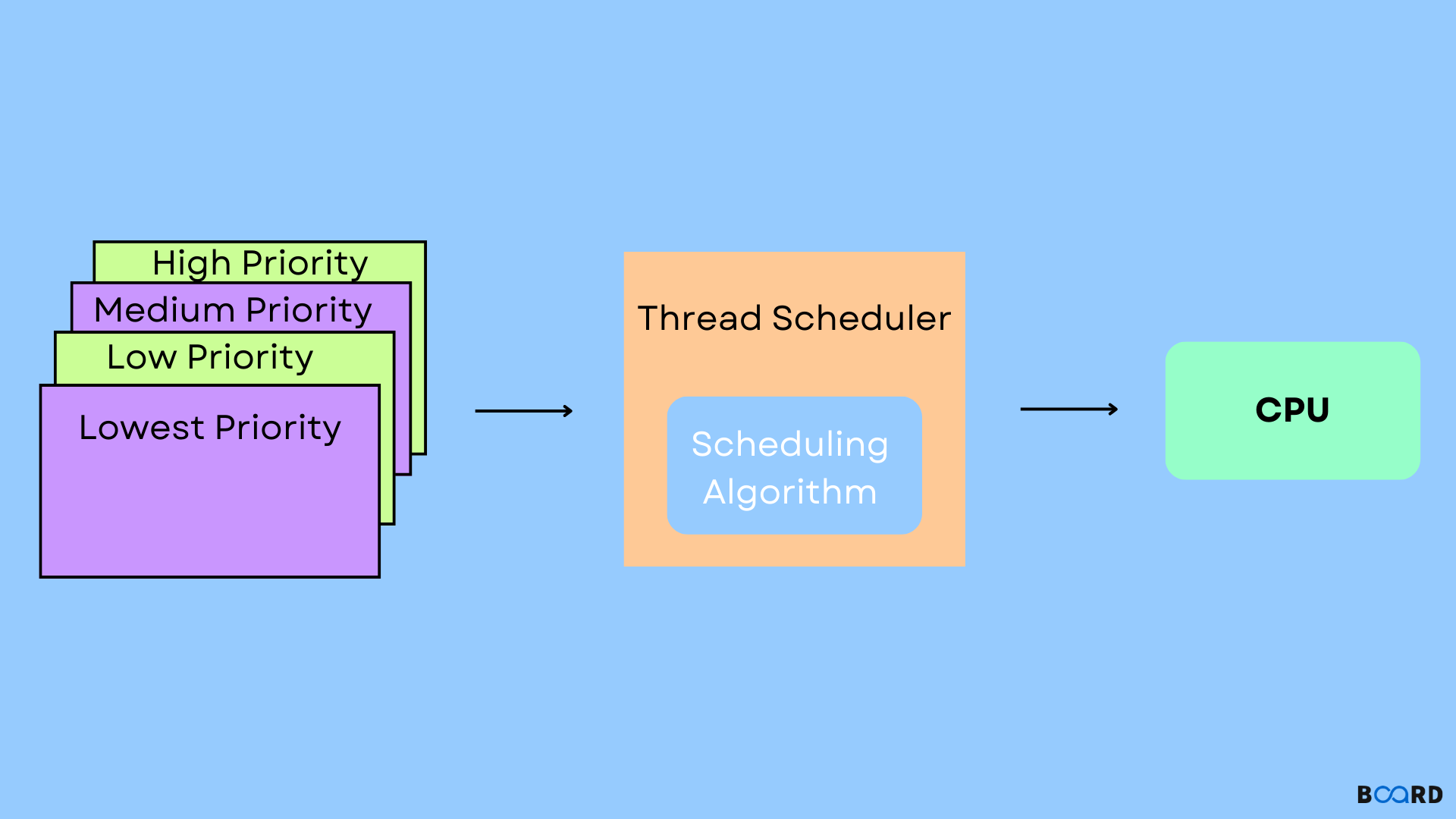
Overview of Thread Execution
JVMs support a scheduling algorithm called fixed-priority preemptive scheduling. Java threads have priorities, and the JVM serves the highest priority first. Whenever we create a Thread, it has its default priority. If multiple threads are waiting to run, the JVM selects the highest-priority thread and executes it.
The lower-priority threads will replace this thread if it is stopped or cannot run. The JVM executes threads in FIFO order if they have the same priority. When a different thread runs, one of two things can happen:
- First, threads with higher priorities become runnable.
- The current thread is terminated or yielded (temporarily paused to allow other threads to run)
Generally, the highest priority thread runs at all times. It is possible for the thread scheduler, however, to choose low-priority threads for execution to prevent starvation.
Thread Priority
Each thread executes with a specific priority. Those Priorities range from 1 to 10. Thread schedulers usually schedule threads based on priority, also known as preemptive scheduling.
As JVM determines the priority scheduling, it cannot be guaranteed. Note that Java programmers can also explicitly assign thread priorities in a Java program in addition to the JVM.
The default thread priority in java is 5 for a thread. There are several priority constants in the Thread class in Java that define the priority of a thread. The following are listed:
1. MIN_PRIORITY = 1
2. NORM_PRIORITY = 5
3. MAX_PRIORTY = 10
Thread Priority: Setter & Getter Method
We will discuss the thread priority setter and getter methods.
public final int getPriority(): The java.lang.Thread.getPriority() function returns the thread's priority.
public final void setPriority(int newPriority): The java.lang.Thread.setPriority() function updates or assigns the thread's priority to newPriority. If newPriority is outside the range, the method throws an IllegalArgumentException. The range is 1 (minimum) and max priority of thread is 10.
Code Sample
Here is the simple code demonstrating Thread Priority works in java. Here we have tried the getter and setter methods to manupulate the thread priority.
Output
Conclusion
Threads are independent sub-processes of processing that share memory and are independent of each other. The Thread Priority in Java determines the priority at which multiple threads get processed.