Java Programming Techniques and APIs
What is Static Class in JAVA?
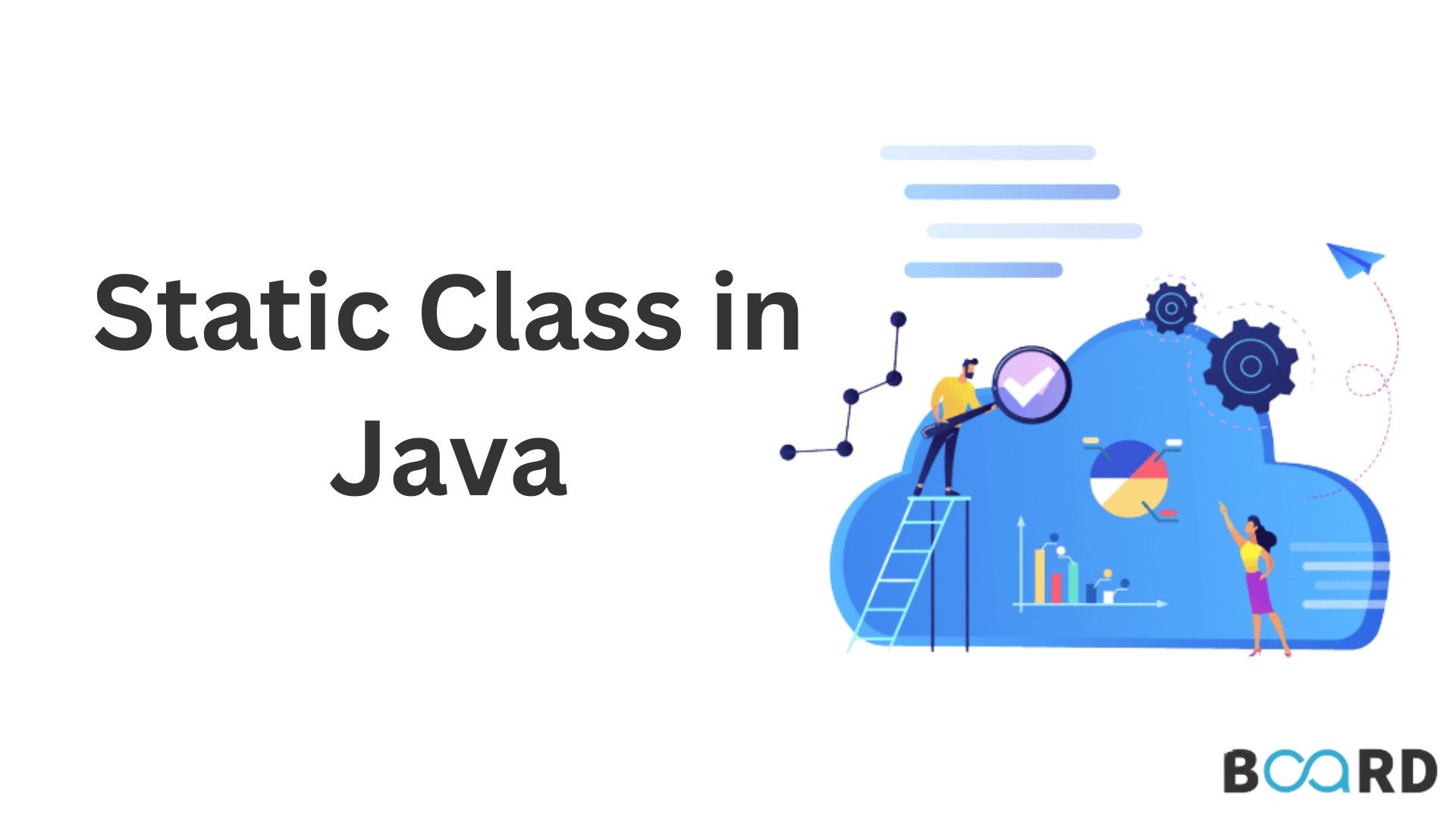
Introduction
In Java, a class is a group of objects from which different types of objects can be produced. For instance, you could make a class called "Car" with SUVs, sedans, trucks, and luxury cars as possible members. The declaration is simple:
Nested Classes
Classes that may be defined inside the code of another class are called nested classes. We may organize a group of logically linked classes using nested classes. It aids in simplifying and making the code more legible. Additionally helps us better apply the idea of encapsulation (data hiding).
Static Classes in Java
Only nested classes may be designated as static classes in Java. A top-level class cannot be declared as static, hence this will result in an error. How do static classes work? Even though they may be nested classes that appear in the middle or at the bottom of the program hierarchy, static classes behave as top-level classes (within a class). The reference to the outer class is not required for static classes. Within themselves, they behave as the upper class. On the other hand, reference to the outer class is required for typical non-static nested classes. Static classes, in contrast to other inner classes, are dependent on the outer class, to put it another way.
Declaring a Static Class
Within our "Car" class, we can declare a static class using the following syntax:
The term "static" in this context guarantees that class sedan would be regarded as upper class. In contrast to non-static nested classes, static classes can only interact with static members of an outer class, whereas static classes can only access non-static members of such an outer class. This feature enables code reuse and interaction between static nested classes and other program elements.
Adding a Declarative Object to a Static Class
The name of the outer class can be used to access a static class. For instance
Only by using the outer class to access the static class can you build objects for it. The syntax is as follows:
The keyword "new" is being used here to create a new object.
Code Example for static class
Here is a straightforward Java application that shows how to use a static class. The usage of the keyword "static" is the only way that the static class is able to access a variable of its outer class.
In this case, a message has been specified as a static string value. It is static, thus only the static class "sedans" may access it. The compiler will display the phrase "Cars are meant for transportation" when it comes across the System.out.println line.
Benefits of a Static Class
A static class may access the inner class's private variables and methods, just like other nested classes can. Static classes might be declared to remove restrictions put on an outer class's member classes. If we believe the outer class is too limited and we want it to perform more activities, we may use the "static" keyword to give a member class greater capability. A static class can never have an instance. Static classes are unable to directly access a class's non-static members. It is the only way it can speak to them without using an object reference. This is both a gain and a drawback of a static class.