Java Programming Techniques and APIs
A quick guide to Stack Class in Java
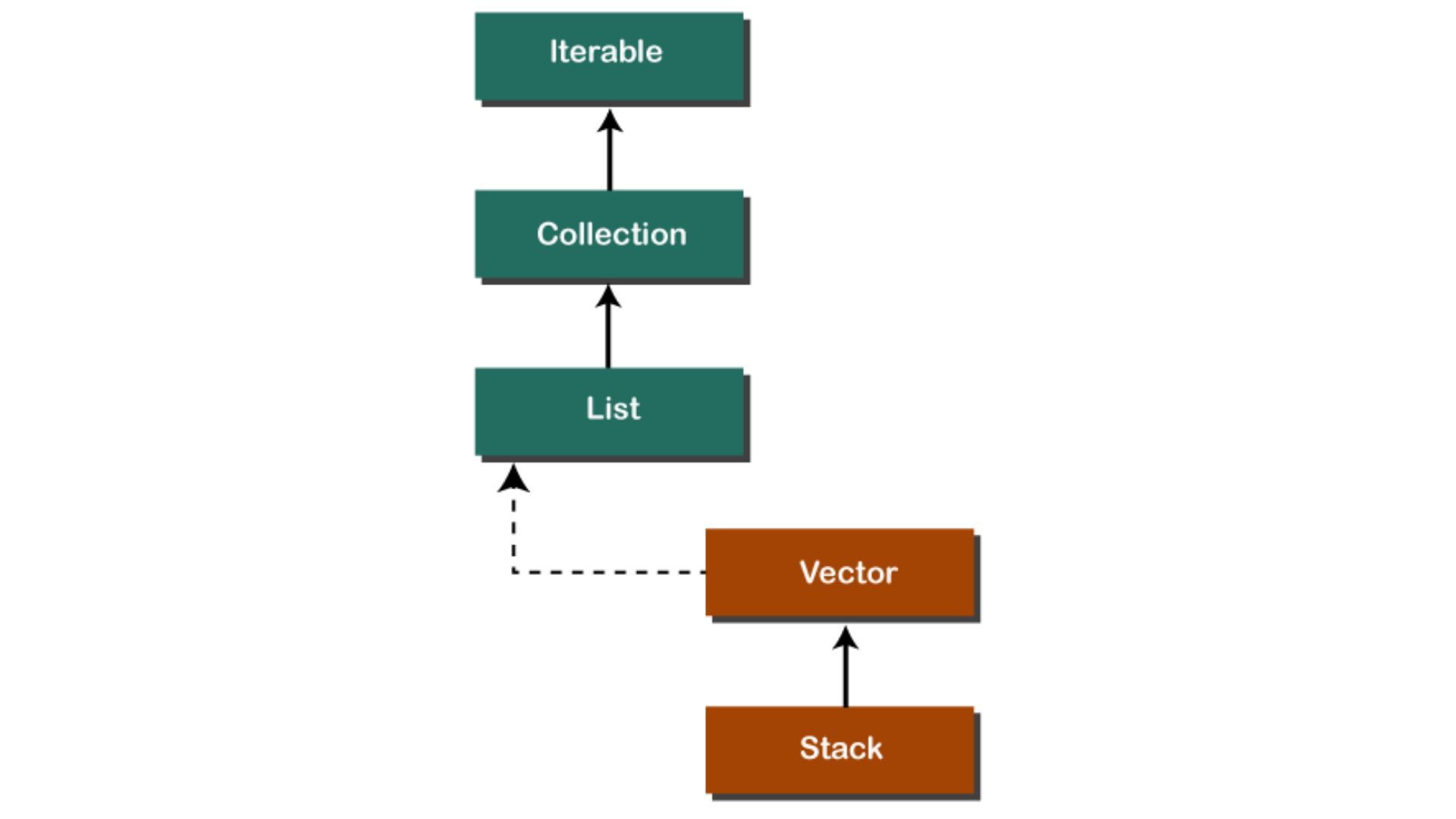
Introduction
Java is a general-purpose computing language and is particularly suitable for developing Internet and enterprise applications. This article will help you understand the concepts of the stack in java and its implementation.
What Is Stack Class In Java?
Stack is a Java class that extends Vector in the Collection framework. In addition, it implements the interfaces List, Collection, Iterable, Cloneable, and Serializable. A LIFO (last-in-first-out) stack represents a set of objects stacked in ascending order.
To use the Stack class, we must first import the java.util package. This diagram illustrates how the stack class gets organized within the Collections framework.
Stack Class Constructor
In the Stack class, there is only one constructor, which creates an empty stack by default.
public Stack()
Creating a Stack
The java.util.Stack package must get imported first to create a stack. The following steps show how to create a stack in Java once you import the required package.
The Type field indicates the datatype of the stack. For example,
Methods of the Stack Class
On the stack, we can perform push, pop, peek, and search operations. To implement these operations, the Java Stack class provides five methods. Moreover, it supports all the Java Vector class methods as well.
1. empty() Method
In the Stack class, the empty() method determines whether the stack is empty or not. It returns true if the stack is empty, and false otherwise. Alternatively, we can use the vector class's isEmpty() method.
Syntax:
Result: If the stack is empty, the method returns true, else it returns false.
2. push() Method
In this method, an item gets inserted at the top of the stack. As with the Vector class, it works similarly to addElement(item). It passes a parameter item to push into the stack.
Syntax:
Result: The method returns the parameter we passed as an argument.
3. pop() Method
Methods remove objects from the stack and return the same object. When the stack is empty, it throws EmptyStackException.
Syntax:
Result: It returns an object positioned at the top of the stack.
4. peek() Method
This function looks at the element at the top of the stack. If the stack is empty, it also throws an EmptyStackException.
Syntax:
Results: This function returns the top elements of the stack.
5. search() Method
This method searches for the object in the stack starting at the top. This function parses a parameter to get searched for. As a result, it returns the object's 1-based position in the stack. In the stack, distance 1 is considered the topmost object.
Consider the case where o is a stack object we are trying to locate. The method returns the distance between the nearest occurrence and the top of the stack. To search in the stack, it uses the equals() method.
Syntax:
Result: The object's location from the stack's top. The return value of -1 indicates that the object is not on the stack.
Stack Implementation in Java
Following example will help you understand how to implement Stack class in java
Output:
Conclusion
The Stack class is one of the most widely used classes in Java. It is a data structure where items can be added and removed in a last-in-first-out(LIFO) manner. Once an item has been placed on the Stack, it can only get removed.