Java Programming Techniques and APIs
Java Bytecode
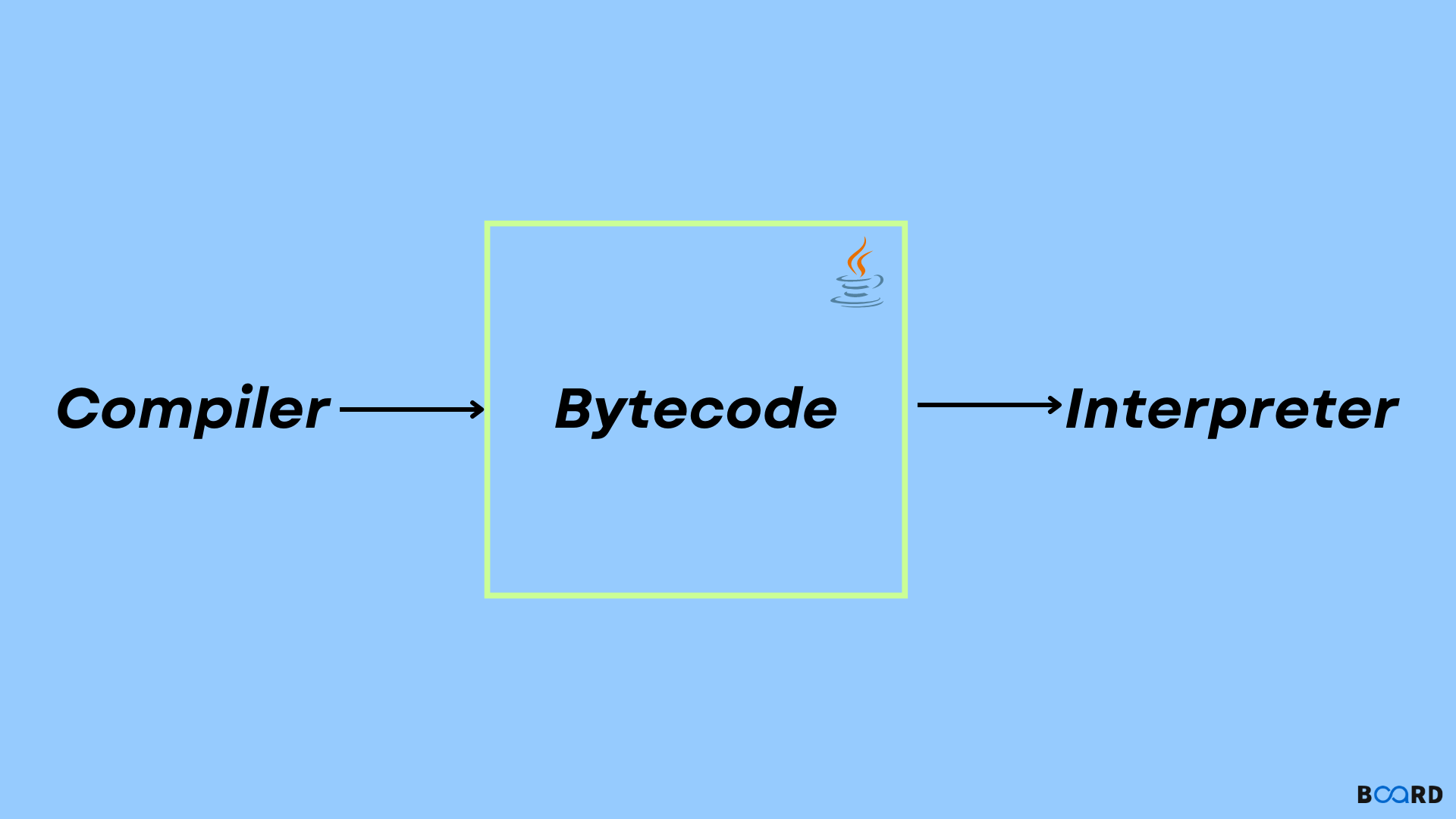
Overview
Bytecode is the result of a Java program’s compilation and is an intermediate machine-independent representation of the Java program. The bytecode of a Java program is processed by the J.V.M. (Java Virtual Machine). JVM is also responsible to make the required resource calls to the CPU for running the bytecode.
Scope
- How is bytecode generated?
- A code example.
- Advantages of bytecode.
How is bytecode generated?
The Java compiler converts Java program source into machine code, also referred to as bytecode. The interpreter, i.e. the JVM then executes the bytecode on the system. The bytecode is the common code form between the Interpreter and the compiler.
Bytecode can be seen as a machine-level code that is executed in the JVM. When we load a class, it gets a bytecode stream per function of that class. When during the execution of a program, a function is called, the bytecode for that function is invoked.
A code example
For a clearer understanding, have a look at the snippet of code below:
Output
The code block written above is a Java source program, which is compiled by the compiler. Eventually, the interpreter runs the compiled code. When one writes a Java program, it is not directly written in machine code but rather in a high-level language, in this case, java.
However, one’s computer system can understand only machine code. Therefore when one executes one’s program, the code is initially transformed into bytecode (machine code) by the compiler, and then the interpreter executes it. The bytecode is the intermediate code and can be executed on any platform.
Advantages of bytecode
- Its platform-independent nature is the most prominent reason why James Gosling began the development of Java. It is the implementation of bytecode that enables one to achieve the platform independence of Java.
- Hence bytecode becomes a very crucial concept in Java programming. The instruction set produced by JVM may vary with the system, but any system can interpret a bytecode. It must be noted that a bytecode is not itself a runnable code, but relies upon the presence of an interpreter that can execute it. This is where JVM comes into the scene.
- The implementation of the concept of bytecode makes Java a platform-independent language, helping in adding to the portability of Java, something that many other languages, such as C, C++, etc. Its portable nature ensures that Java is implementable on a plethora of platforms such as mobile phones, severs, desktops, etc.