Java Programming Techniques and APIs
How to Handle Exceptions in Java?
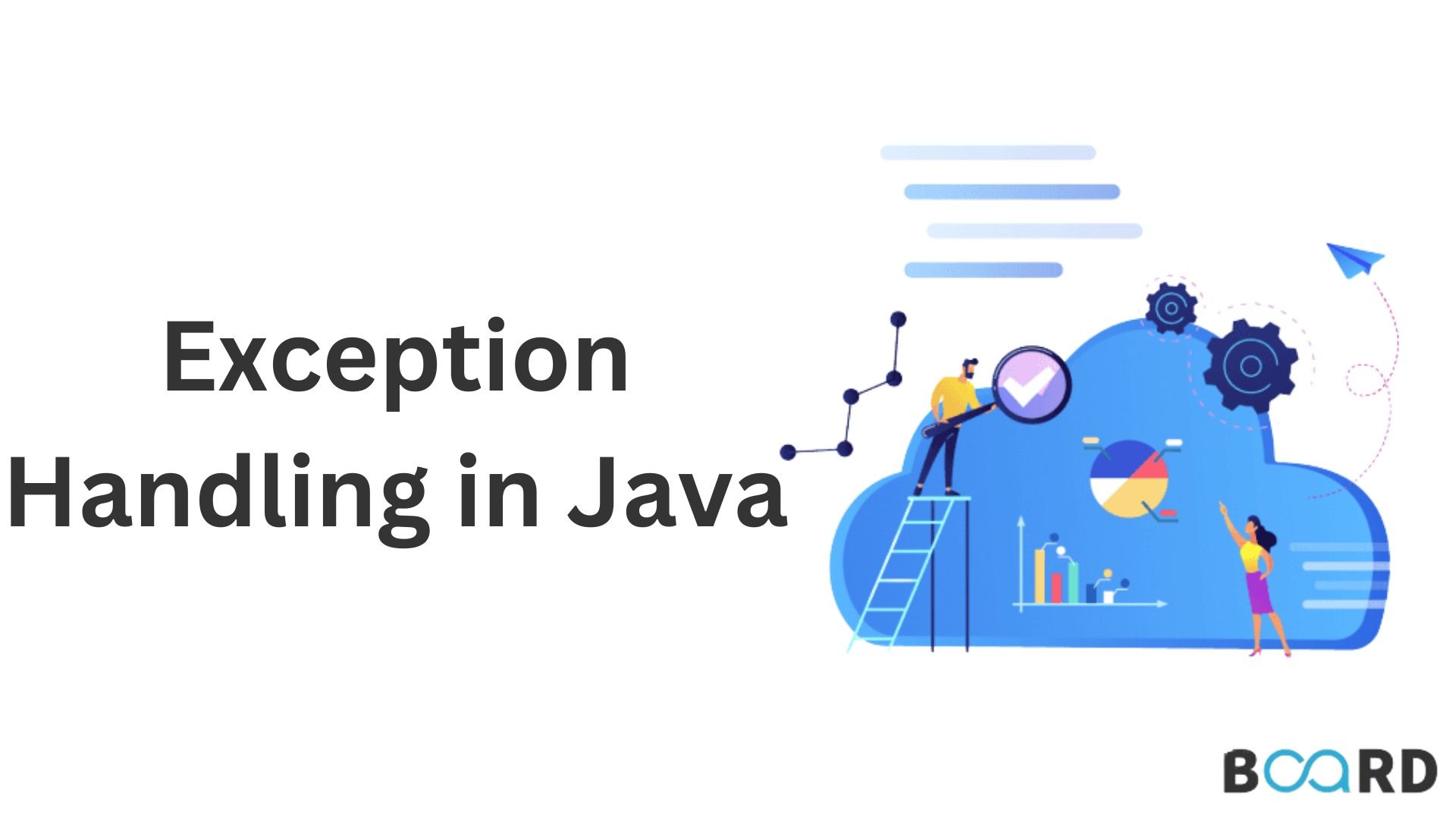
Exception In Java
Program exceptions occur when a problem arises during execution. When an exception occurs, the program/application terminates abnormally and should get avoided. It is necessary, therefore, to handle these exceptions. There are many reasons why an exception may occur.
A few scenarios where exceptions may occur are listed below.
- The user entered incorrect data.
- There is a problem opening a file.
- The JVM has run out of memory or the network connection has been lost.
There are several reasons why these exceptions occur, including user error, programmer error, or physical resource failure.
What Is Exception Handling In Java
Whenever an exception occurs within a method, the method creates an Exception Object and passes it on to the runtime system (JVM).
Exception objects contain information about the exception, including its name and description, with the program's current state.
It refers to throwing an Exception when the run-time system creates and handles the exception object.
There are specific keywords that can get used to handle exceptions in Java.
- throw –
The Java runtime creates an exception object as soon as an error occurs and begins processing the exception. In some cases, we may need to generate exceptions. Null passwords, for example, should be thrown by an authentication program to clients. The throw keyword allows you to throw exceptions to the runtime.
- throws –
Methods that throw an exception and don't handle it must use the throws keyword in their signature to inform the caller program of the exceptions they might throw. With the throws keyword, a caller method can handle these exceptions or propagate them to one of its caller methods. With the throws clause, we can specify multiple exceptions, including the main() method.
- try-catch –
For handling exceptions, we use a try-catch block. To handle exceptions, the try block starts with the keyword try and ends with the catch block. You can use more than one catch block for one try block. Try-catch blocks can also be nested. You need to specify an Exception type as a parameter in the catch block.
- finally –
In a try-catch block, the final block is optional. When an exception occurs, the execution process gets stopped, so it might be necessary to use the final block if we have some resources open that won't be closed when the exception occurs. Regardless of whether an exception occurs, the final block always executes.
The syntax for try-catch Clause
Hierarchy of Exception in Java
The Java.lang.Exception is the base class for all exception classes. Exceptions are subclasses of Throwable. A subclass derived from Throwable called Error is also available in addition to the exception class.
The Java program does not handle errors that occur due to severe failures. The runtime environment generates errors that indicate errors. An example would be that the JVM runs out of memory. In most cases, programs cannot recover from errors.
There are two main subclasses of the Exception class: IOException and RuntimeException.
Example of Java Exception Handling
In this example, we will use a try-catch statement to handle a Java Exception.
Conclusion
Exception handling is a critical part of any modern software development stack. Exceptions are encountered everywhere, from the user to system APIs and from external libraries to your own code. Understanding how to deal with exceptions will help you debug issues in your own code, and also make it easier to work with the many external systems your software interacts with.