Java Programming Techniques and APIs
Trim in Java
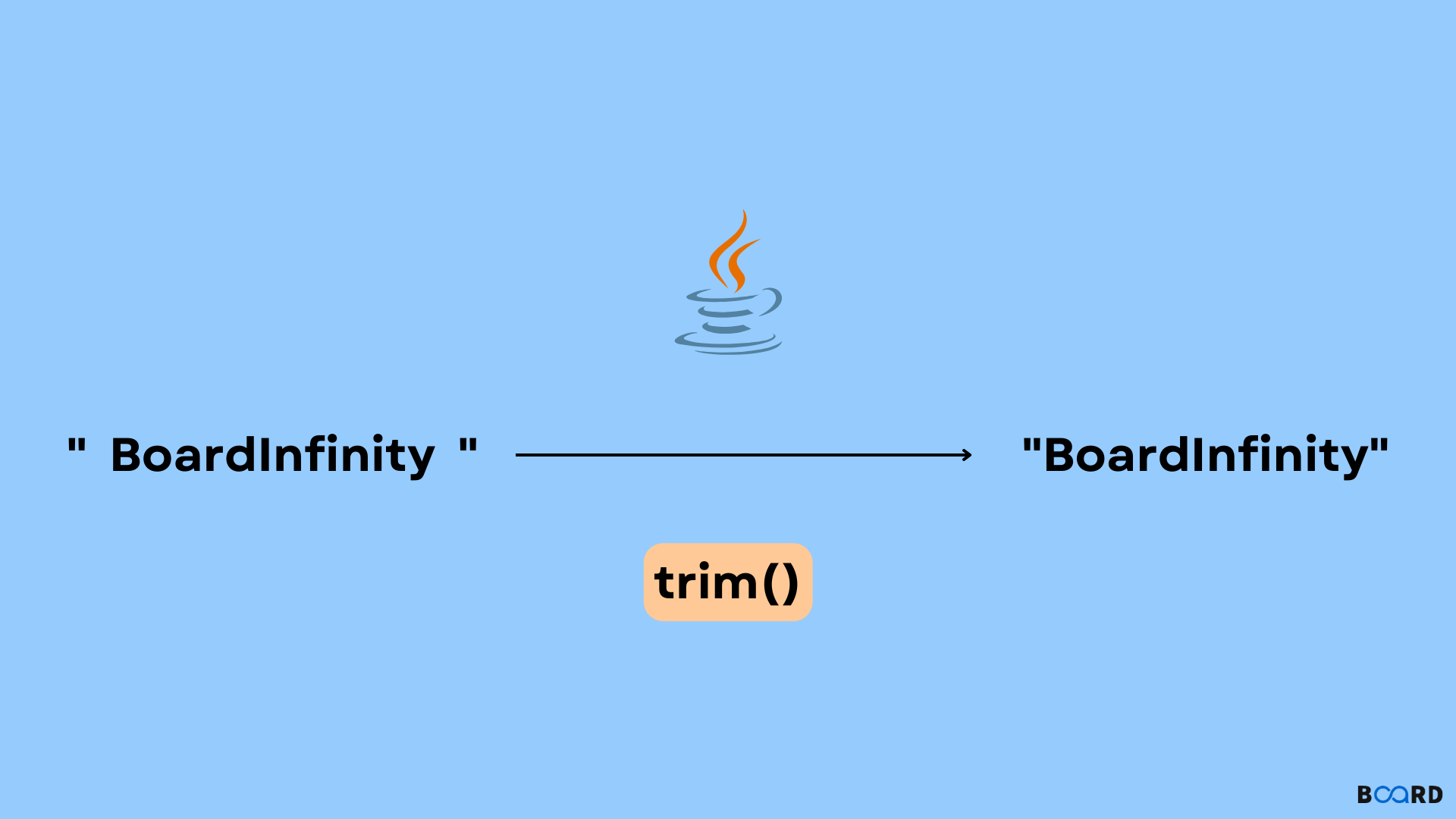
Introduction
The trim() function in Java removes all leading and trailing whitespace.
Java.lang package's String class contains the definition and usage of this method. The trim() function String as return value. A copy of the original string that has leading and trailing spaces removed is returned.
Syntax
The following is the Java syntax for trim():
Parameters
In Java, the trim() function does not accept any parameters.
Code Sample
Assume that "BoardInfinity" has whitespaces at its beginning and end. Then, as shown in the code below, we can remove spaces with the trim() method.
Code:
Working Of The Trim() In Java
The white space characters have the Unicode value '\u0020'. During the Java code execution, the trim() method checks whether the Unicode value '/u0020' appears before or after the string. The trim() method trims the string if it contains the Unicode value '/u0020' and returns a new string without a Unicode value.
Note:
- As an output of the trim() method in Java, a new String object is returned without leading or trailing spaces.
- Unlike other methods, this method does not modify the original string.
- As far as Java is concerned, the trim() function does not remove middle spaces between two strings.