Java Programming Techniques and APIs
A practical guide on Synchronization in Java
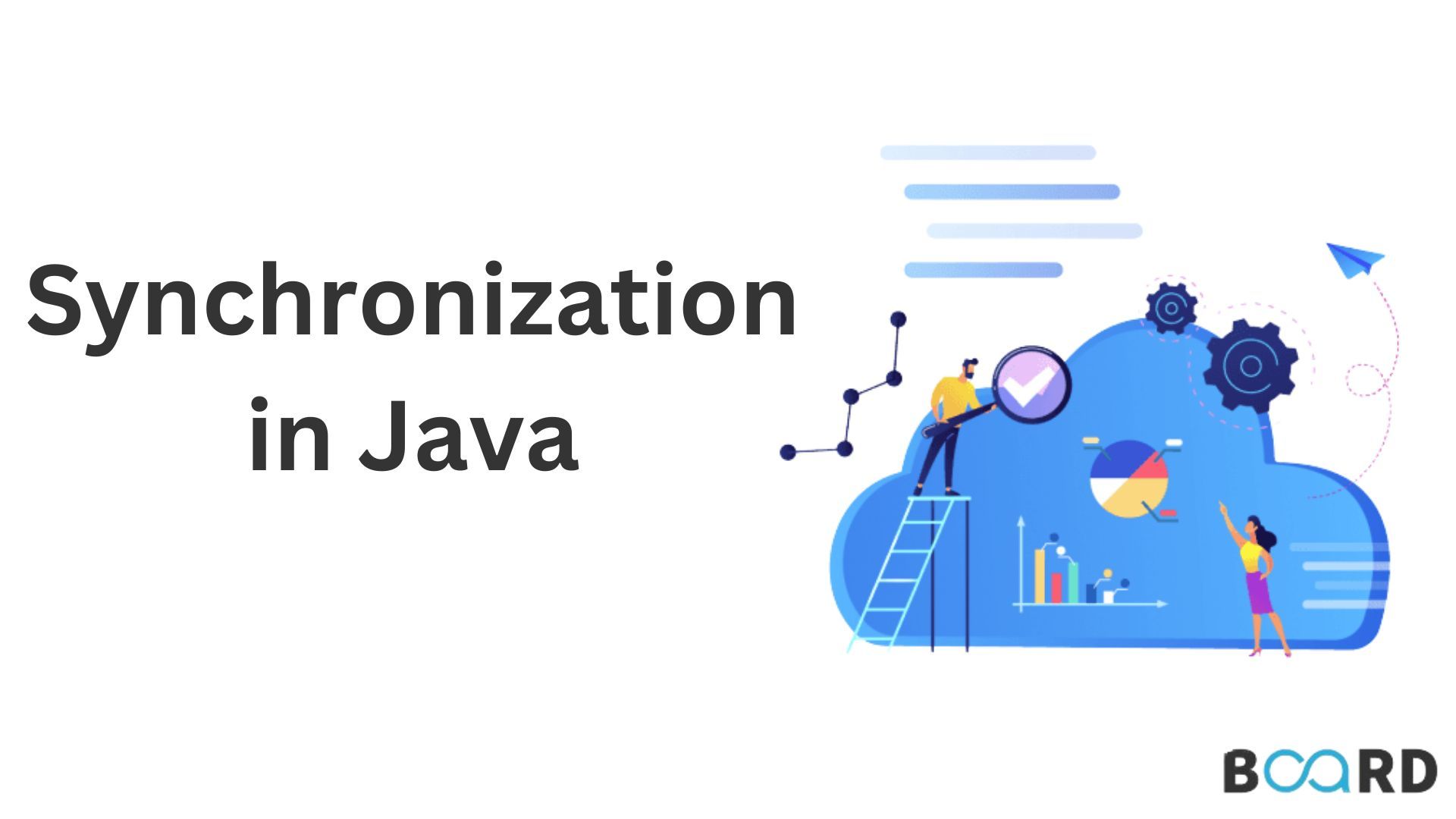
What Is Synchronization In Java?
Java's synchronization feature controls reference to shared resources by multiple threads.
In the Multithreading concept, multiple threads attempt to access the same resource simultaneously, which leads to inconsistent results.
Therefore, synchronization is essential to ensure reliable communication between threads.
Here, the objectidentifier refers to an object having a lock associated with the monitor represented by the synchronized statement.
Why do we use Synchronize in Java?
As we know, Synchronize method in java language allows multiple processes to access a shared resource simultaneously. And multiple threads attempt to access shared resources simultaneously under the Multithreading concept, resulting in inconsistent outcomes.
Hence, synchronization must ensure that only one thread can access a resource simultaneously. With the help of synchronized blocks, java allows you to create threads and synchronize their tasks.
The reliability of thread-to-thread communication depends on synchronization. As a result of synchronization, thread interference gets prevented, and with synchronizing, concurrency issues can get avoided.
The types of Synchronization
Synchronization can get divided into two types.
1. Process Synchronization
2. Thread Synchronization
Process Synchronization
Processes are nothing more than programs in action. In this case, it functions as a separate process having no connection to any other. The operating system provides memory and CPU resources to the process. As a result of "process synchronization," two or more processes can share capabilities while ensuring the consistency of their data. Several processes share the Critical Section in a programming language.
Thread Synchronization
As the name implies, Thread Synchronization refers to the simultaneous execution of a vital resource by more than one thread. In a single process, a thread consists of a subroutine that can run independently.
There can be numerous threads in a single process, and the program can schedule each thread to use a particular resource simultaneously. A single thread actually consists of several threads.
There are two forms of thread synchronization:
1. Mutual Exclusive
2. Collaboration (Inter Thread Communication in java)
Code Example
This code will help you understand the concept of Java synchronized methods.
Conclusion
Synchronization in Java regulates the access of multiple processes to a shared resource. The Multithreading concept results in inconsistent outcomes when multiple threads try to access shared resources simultaneously. Communication between threads requires synchronization. The synchronization of threads can prevent interference between them.