Java Programming Techniques and APIs
Understand Serialization and Deserialization in Java
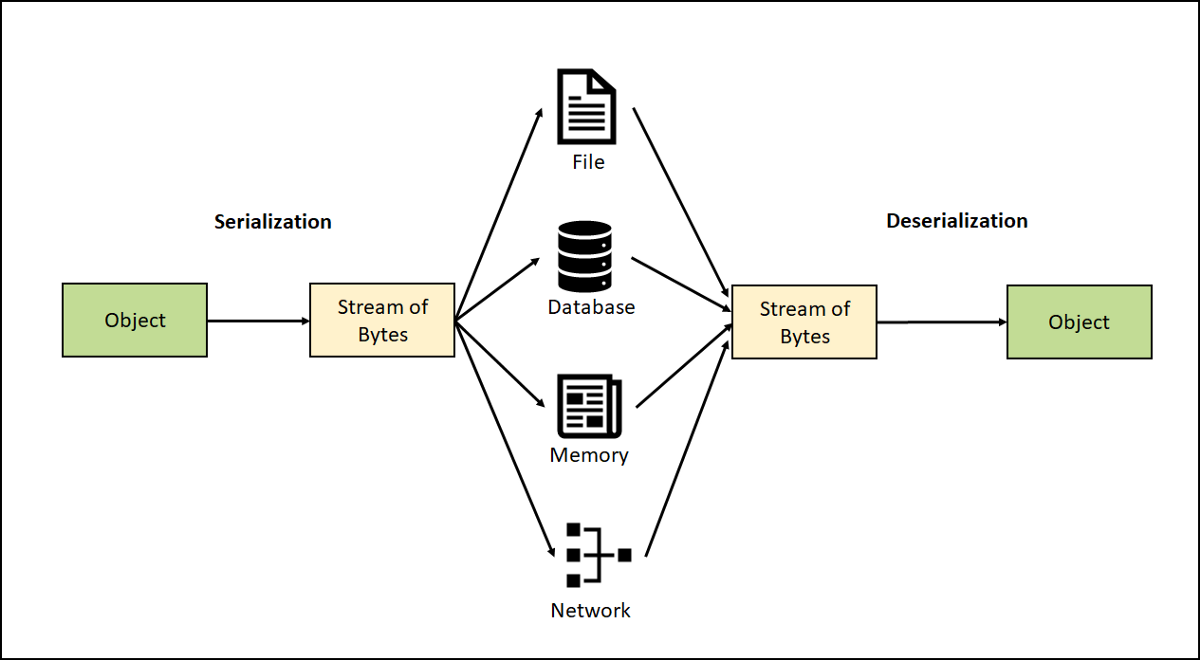
Introduction
One of the most misunderstood aspects of Java programming is its serialization feature, a process that allows you to store and transfer data in an efficient way between different programming languages.
This article will give you an overview of the topic, explaining what it is and how it works.
What Is Serialization in Java?
Serialization in Java refers to the step of converting an object into a byte stream that can then pass through a network, be stored in a disk, and get rebuilt when needed.
For this, Java provides a serialization API.
It is platform-independent to create a byte stream. Therefore, the program can deserialize the objects serialized from another platform. Serializing a Java object requires implementing the java.io.Serializable interface.
We can use writeObject() method from ObjectOutputStream for serializing any required object. The general syntax for calling the default function is
How Does Java Serialization Work?
As part of Java serialization, Java reflection scrapes data from all object fields, including final and private. The serialization process recursively serializes objects that exist in fields. Although Java has getters and setters, these functions are ineffective during serialization.
What Is Deserialization in Java?
As the name suggests, deserialization is the opposite of serialization. By deserializing an object, you re-create it in its original state, starting with a byte stream. For a successful re-creation, you must first have the definition of the object.
How Does Java Deserialization Work?
The deserialization process of a byte stream back to an object does not use the constructor. By using reflection, it writes data into the fields of the empty object. In the same way, as serialization, private and final fields are also included.
Benefits of Serialization
- Provides a means of transferring objects over a network.
- Entity statuses become persistent when they are saved.
- No requirement for a Java Virtual Machine.
- It's easy to understand and personalize.
Sample Code
Here is an example of saving a Student object to a local file and reading it back:
Here we used ObjectOutputStream to save the object's state using FileOutputStream. The file “outputfile.txt” will get created in the project directory.
After this, the file gets loaded using FileInputStream. ObjectInputStream picks this input and converts it into a new object called s2.
Our final step will be to ensure that the loaded object matches the original object's state.
Conclusion
Serialization and deserialization are simple in many programming languages, such as Java, where space is allocated for the object's memory, data is stored, and it is read back into another object. If you want to learn more about serialization in Java or front-end programming, we would highly recommend checking out our Discussion board as well.