Java Programming Techniques and APIs
Checked vs Unchecked Exceptions in Java
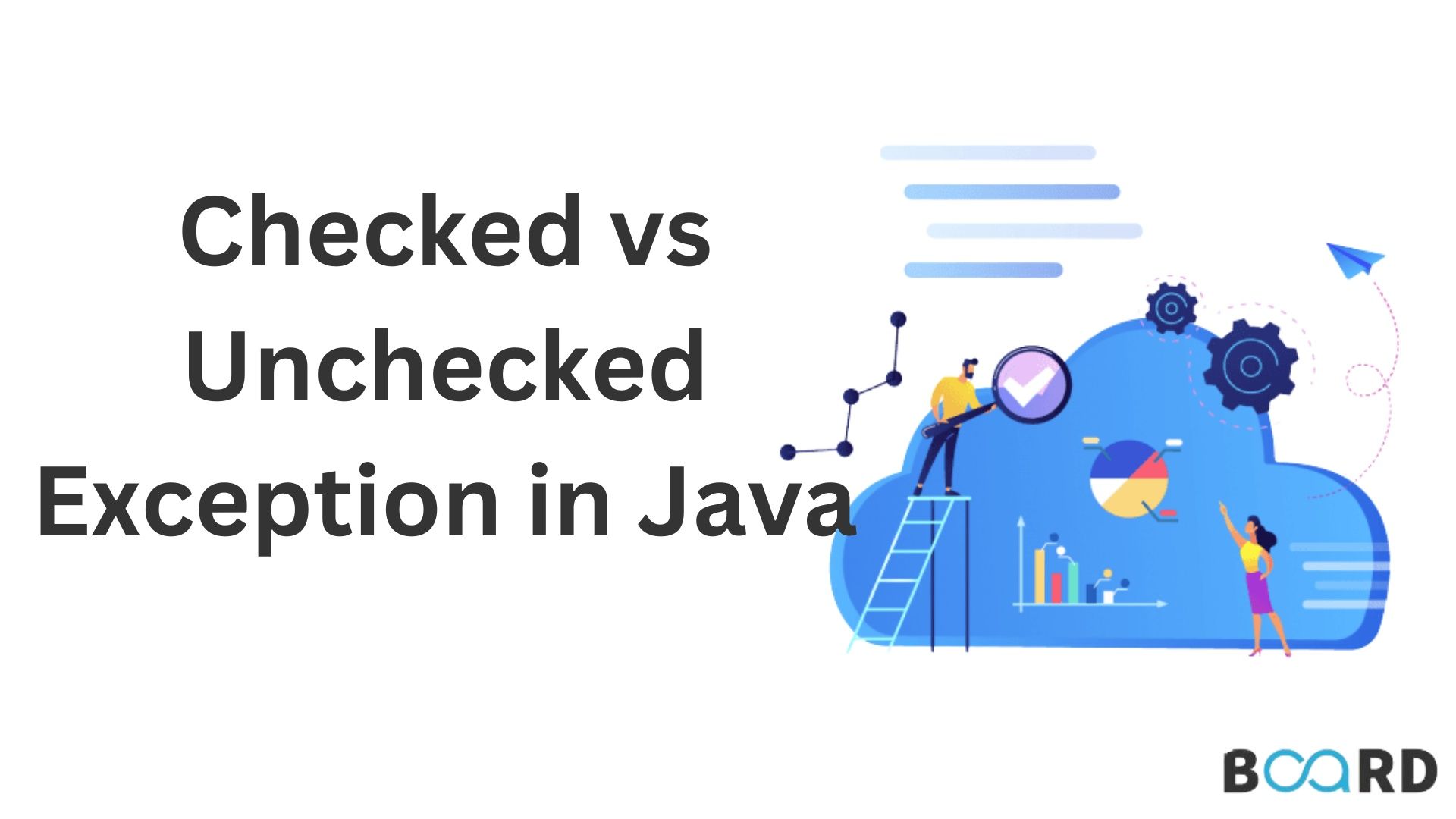
Checked Exceptions
Generally, a checked exception (also called a logical exception) in Java occurs when your code goes wrong and is potentially recoverable.
Suppose we get a client error when calling another API; then, we could retry from that exception and see if it runs again.
The compiler catches checked exceptions at compile time, so if something throws one, the compiler will make sure you handle it. A checked exception represents an error outside of the program's control. FileInputStream, for example, throws a FileNotFoundException if the input file isn't found.
Checked Exception Example Code
Therefore, we should declare a checked exception by using the try-catch block.
Try-Catch
A try-catch block wraps the Java code that throws the checked exception. As a result, you can now process and handle the exception.
Unchecked Exceptions
Unlike checked exceptions, unchecked exceptions represent errors in programming logic, not erroneous situations that may reasonably occur when using an API.
The compiler cannot anticipate logical errors occurring only at runtime, so it cannot identify these problems at compile time. This is why they are called "unchecked" exceptions. The program won't generate a compilation error even if the programmer doesn't handle an unchecked exception.
Moreover, unchecked exceptions don't have to get declared in methods with throws. It occurs most often when the user provides insufficient or wrong data when interacting with the program. A programmer must determine which conditions will cause such exceptions and take appropriate action accordingly.
The unchecked exception is a direct subclass of the RuntimeException class. In software, unchecked exceptions can arise from faulty logic anywhere in the code.
For example, A NullPointerException occurs if a developer invokes a method on a null object. Unchecked ArrayIndexOutOfBoundsException occurs if an array element does not exist.
Here are some unchecked exception classes:
- NullPointerException
- ArrayIndexOutOfBoundsException
- ArithmeticException
- IllegalArgumentException
- NumberFormatException