Java Programming Techniques and APIs
Java Array and ArrayList: Differences
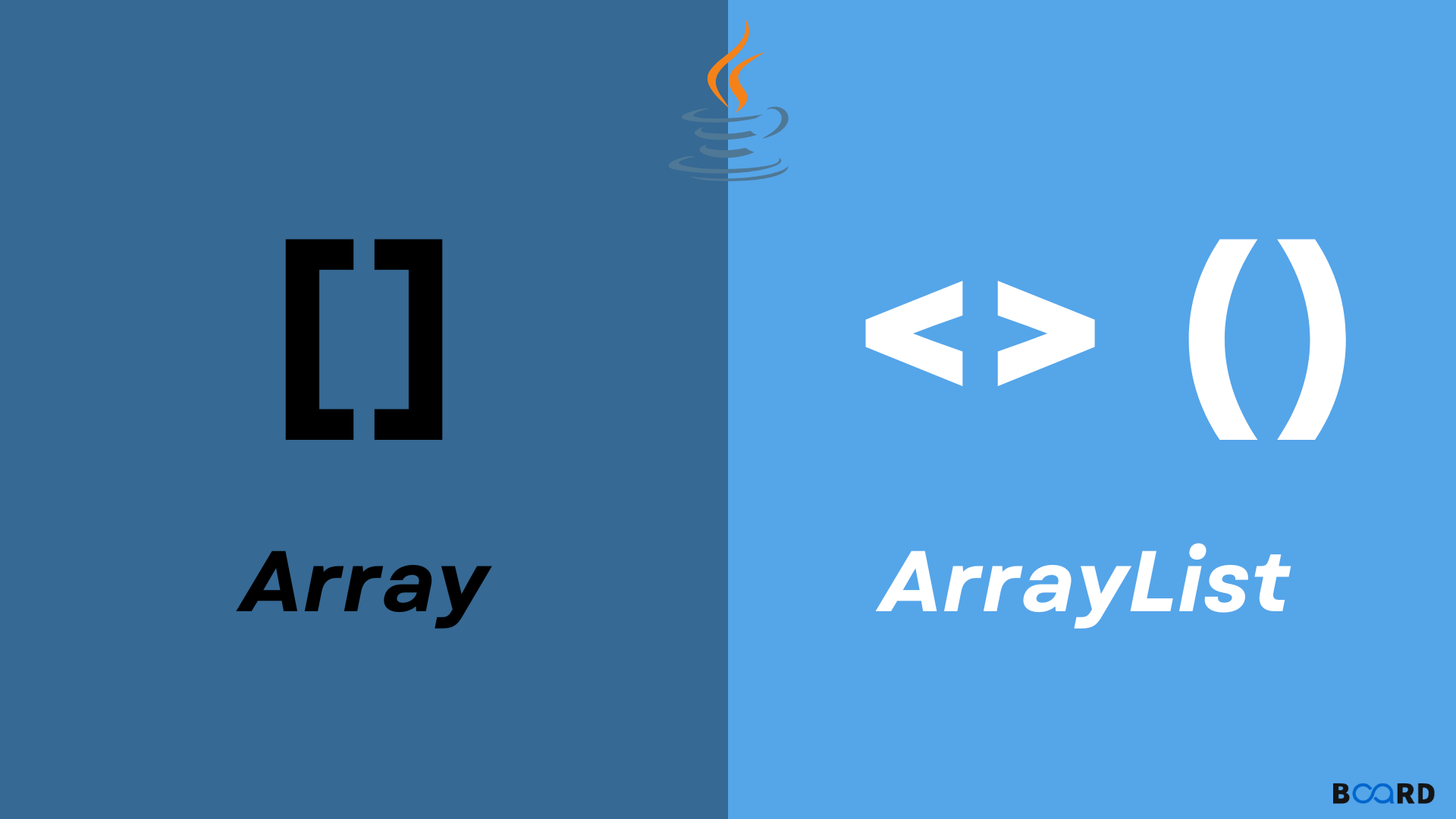
Introduction
An array is a linear data structure that is used to store elements in a contiguous memory location. The ArrayList is a class in Java that is similar to a dynamic array and belongs to the collection framework. The ArrayList class works almost similar to the vector class in C++.
Ways to create an array in Java
1. Static array or Simple fixed-sized arrays.
2. Dynamically sized arrays
We can declare a static array and initialize it later or we can initialize an array at the time of declaration. Let us consider the following program that illustrates how we can declare a static and dynamic array:
Source Code
Output:
Output Description:
As you can see in the output, the first element of both the arrays have been displayed.
Creating an ArrayList
Java provides us the ArrayList class using which we can store elements just like a dynamic array. The ArrayList is a part of the collection framework in Java. We can access the elements of an ArrayList using the [] operator. The ArrayList class also provides methods to access elements.
We can declare an ArrayList in Java using the following syntax:
Source Code
Output:
Output Description:
As you can see in the output, ArrayList elements have been displayed.
Differences Table
The differences between an ArrayList and an array are the following:
Conclusion
In this tutorial, we discussed how we can create an array and ArrayList in Java. We also highlighted differences between array and ArrayList. We believe this tutorial has helped you to improve your Java knowledge.