Java Programming Techniques and APIs
Priority Queue In Java
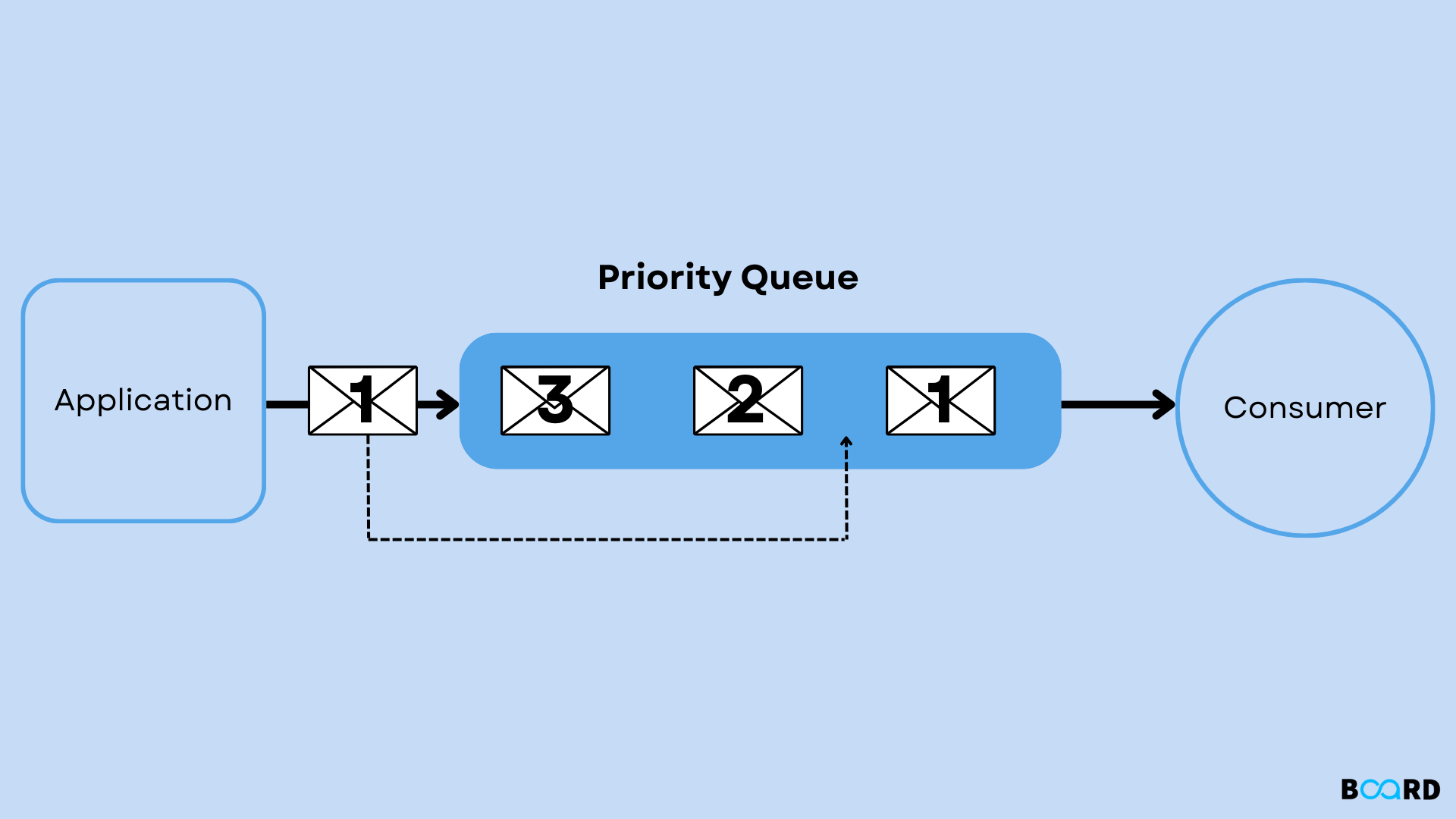
Introduction
A priority queue is an abstract data-type similar to regular queue or stack data structure. Each element in a priority queue has an associated priority.
In a priority queue, elements with high priority are served before elements with low priority.
The heap data structure's functionality is provided by the PriorityQueue class.
It conforms to the Queue interface.
The Queue interface is implemented by the Java PriorityQueue class.
Priority queue elements are retrieved in sorted order, as opposed to conventional queue elements.
Assume we wish to get elements in ascending order. In this situation, the smallest element will be at the top of the priority queue. When this element is retrieved, the queue's head will be the next smallest element.
It is vital to realize that priority queue entries may not be sorted. Elements, on the other hand, are always returned in sorted order.
Making a PriorityQueue
Importing the java.util.PriorityQueue package is required to establish a priority queue. Here's how to make a priority queue in Java when we import the library.
We've constructed a priority queue without any arguments. In this situation, the smallest member of the priority queue is at the top of the queue. And elements are deleted from the queue in ascending order.
The Comparator interface, on the other hand, allows us to change the ordering of components.
PriorityQueue Declaration
Let's look at the declaration for java.util.PriorityQueue class.
A few key points about Priority Queue are as follows:
- PriorityQueue does not support null values.
- We can't make a PriorityQueue of non-comparable objects.
- Unbound queues are PriorityQueues.
- The least element in this queue according to the provided ordering is at the top. If numerous elements are tied for the lowest value, the head is one of them – ties are broken at random.
- Because PriorityQueue is not thread-safe, Java provides the PriorityBlockingQueue class, which implements the BlockingQueue interface and can be used in a multithreading context.
- The queue retrieval operations poll, delete, peek, and element access the queue's first element.
- It has O(log(n)) time for the add and poll techniques.
- It inherits methods from the abstract classes AbstractQueue, AbstractCollection, Collection, and Object.
Examples
TestCollection12.java
Output
Java PriorityQueue: Book
Let's look at a PriorityQueue example in which we add books to the queue and print every book in the queue. PriorityQueue elements must be of Comparable type. Classes like String and Wrapper are by default Comparable. You must implement the Comparable interface in order to add user-defined objects to PriorityQueue.
LinkedListExample.java
Output:
Applications
- Dijkstra and Prim's algorithms are being implemented.
- After K negations, maximize the array sum.
Conclusion
The Priority Queue is an important data structure when dealing with items with various priorities. The main benefit of employing a priority queue is that you can immediately access the highest priority item with a time complexity of only O. (1). The sole drawback to employing Priority Queues is that the enqueue and dequeue operations are sluggish and have an O(1) time complexity (log n).